Category Archives: Java
Java Functional Interface Explained with Code Samples
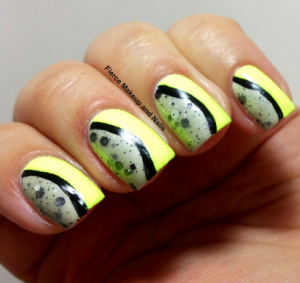
The article aims to describe functional interface in Java 8 with the help of hello world code samples. What is Functional Interface? Simply speaking, a functional interface is the plain old Java interface with JUST ONE and ONLY ONE abstract method also termed as the functional method. However, that does not stop functional interface to not contain default and static methods. Following is an example of a functional interface: @FunctionalInterface public interface HelloInterface { // Abstract method void sayHello( String name ); // Static method static void sayThanks() { System.out.println( “Thank You!” ); } // Default method default void sayHelloWorld() { System.out.println( “Hello World!” ); } } Observations: In the …
What does Interface in Java 8 Look Like?
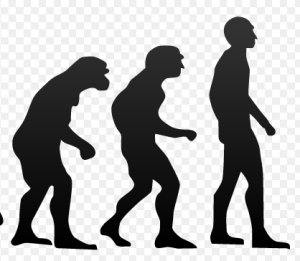
Before we go into looking at different aspects of interface in Java 8, lets look at some of the definitions found on some of the source-of-truth websites that seem to contradict the definition of Interface in Java by defining it with a generic definition despite the fact that interface got evolved (such as below 🙂 and has got a new definition in Java 8. Whether or not, the evolved interface in Java 8 is a good move, is beyond the scope of this article. However, please feel free to share your comments. Lets look at some of the following definitions of a Java interface I took from the web at the time of …
Java 8 Lambda Expressions Examples using Calculator Implementation
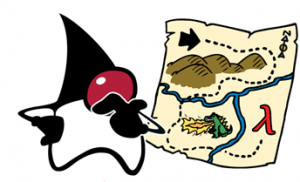
The article demonstrates the Lambda expressions using Calculator (interface) code samples. It also makes use of Functional interfaces from java.util.function package to demonstrate Calculator implementation using BiFunction and BinaryOperator interfaces. Calculator Implementation Demonstrating Lambda Expressions The Calculator methods implementation would be explained using both, traditional approach and the approach making use of Lambda expressions. Traditional Approach Using traditional approach, many would have gone implementing Calculator add, substract, multiply and divide function based on following: Define a Calculator interface with four methods namely add, subtract, multiply and divide; Another approach could be to create a single method with an operation flag and provide conditional implementation based on flag. Create a CalculatorImpl …
Gradle War Configuration for Eclipse Spring Web Application Project
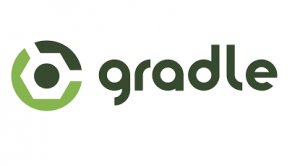
The article describes the configuration one would need to create WAR file for an Eclipse-base web application (Dynamic Web) project. Below code represents whats needed to compile your Java Eclipse-based web application project and create WAR file which can be deployed later, on any Java server such as Tomcat. Pay attention to some of the following facts: repositories: “repositories” is referred in a local directory rather than external repository such as MavenCentral etc. sourceSets: sourceSets define the path under which Java source code exists. It MUST BE NOTED that failing to define this would have Gradle look into Maven-based folder such as “src/main/java” for source code that would eventually result …
Steps to Get Started with Spring MVC 4 & Hibernate 4
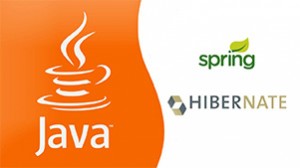
The article lists down steps one need to take in order to get up and running with Spring MVC 4 and Hibernate 4. The article will describe following three key aspects: General configurations to setup Java, Eclipse and Tomcat Server Spring MVC 4 configurations & code samples Hibernate 4 configurations & code samples Following are key components/artifacts that would be required to create the web application which makes use of Spring MVC 4 for managing MVC and Hibernate as an ORM framework: Libraries & Tools Configuration files Components Controller Views (JSP pages) Business logic classes Data access classes Key Libraries & Tools Following are key libraries and tools to download …
Top Bookmarks – How to Get Started with Spring MVC & Eclipse
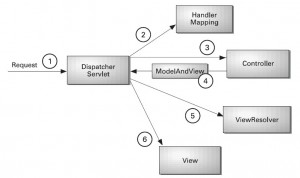
The article lists down top bookmarks (pages) which helped me to quickly get started with Spring MVC with Eclipse. Also, presents some key information at a very high level. Spring MVC Framework Tutorial Spring MVC Form Handling Example Spring MVC Hello World Example Spring 3 MVC Hello World Example Spring 4 MVC Hello World Tutorial – Full Example Following are high level steps to get started with your first Spring MVC project using Eclipse: Download Java EE eclipse and configure server for ease of deployment from within Eclipse. Create a Dynamic Web Project in Eclipse. Drag and drop below mentioned Spring and other libraries into the folder WebContent/WEB-INF/lib. One of …
How to Add Tomcat 8 to Eclipse Kepler
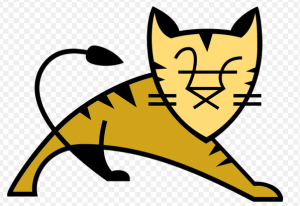
The article represents steps required to configure Tomcat 8 with Eclipse Kepler. Download Tomcat 8 and place it within any local folder. Download Eclipse Java EE Kepler As of date, Tomcat 8 is not supported in Eclipse JaveEE Kepler. However, you could add the Tomcat 8 by doing following: Go to the WTP downloads page, select the latest version (currently 3.6), and download the zip. Here’s the current link. Copy the all of the files in features and plugins directories of the downloaded WTP into the corresponding Eclipse directories in your Eclipse folder (overwriting the existing files). Start Eclipse and click on “Servers” tab in the workbench. Go ahead and …
Tea-Time Java Quiz #1
Java Interview Questions (Part 1) – Rookies Series
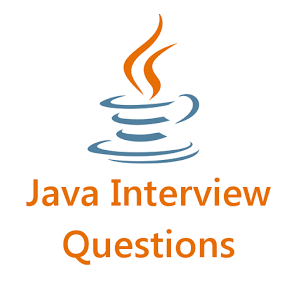
The article (first in the series) aims to present some tricky interview Java questions that could help you score high in your next Java interviews: Programming fundamentals Question: What is difference between object-oriented programming and functional programming? Answer: Following pages present good perspective on the differences: http://stackoverflow.com/questions/6720348/difference-between-oop-and-functional-programming-scheme http://c2.com/cgi/wiki?OoVsFunctional Java Objects Question: What is difference between comparing two objects using equals method and “==” operator? Answer: http://stackoverflow.com/questions/5848370/equals-and-operator-in-java Question: What is the significance of equals and hash method in Object class? Answer: http://www.javaworld.com/article/2074996/hashcode-and-equals-method-in-java-object—a-pragmatic-concept.html Collections Question: What is difference between LinkedList and ArrayList? When would you want to use one and not the other? Answer: http://javarevisited.blogspot.in/2012/02/difference-between-linkedlist-vs.html Question: How come you …
Why & When Use Java 8 Compact Profiles?
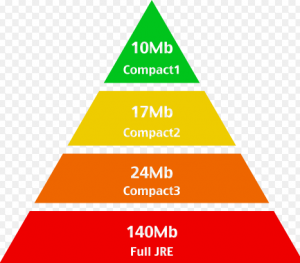
The article presents a perspective on different aspects of compact profiles in Java 8 including relevance of this feature in the first place and, when would you want to use it. As I am also getting up to speed with compact profiles, I do understand that there could be further detail to it that I might have missed and thus, would appreciate if you could share your perspectives as well. What is Compact Profile? As defined on Oracle compact profile overview page, compact profile enable reduced memory footprint for applications that do not require the entire Java platform. In Java 8 release, compact profiles provide three different groupings of libraries …
Java 8 to Face Challenges with Enterprise-wide Adoption
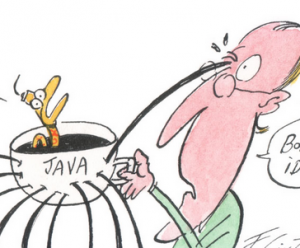
The article represents some of the challenges that an enterprise might face in relation to the adoption of Java 8 across different line of businesses (LOBs). Buy-in from Business & Technology Stakeholders Unlike previous Java releases, Java 8 release has come up with some unique features which are there to sort out some of the existing technical challenges (such as Lambda expressions, Collector APIs for map-reduce transformations, Nashorn engine etc) and, challenges in relation to making Java friendly to different devices and modular (compact profiles) in coming future. The primary issue that business and technology stakeholders are going to face is to find out and get convinced with business and technology relevance …
Java Developers Readiness to Get Started with Java 8 Release
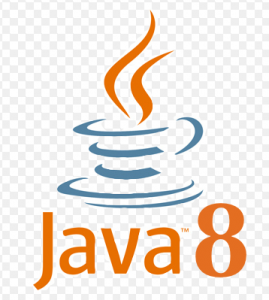
The article represents different set of information that Java developers (working on 5, 6, 7 versions) may need to learn prior to getting started and make best use of Java 8 features. In case, you feel there are other topics that developers need to look beforehand, please suggest. Following are different concepts which Java developers working with earlier versions of Java need to understand very clearly in order to get started comfortably with Java 8 features. Functional programming concepts Learning functional programming concepts would help you learn Java 8 flagship feature such as Lambda expressions. In short, Lambda expressions helps you treat functionality (represented as code) as data which you …
Java Lambda Expressions Explained with Examples
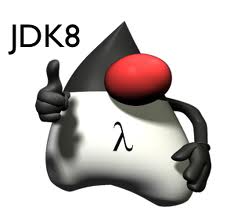
The article represents different viewpoints on Java Lambda Expressions (Java 8) to help Java developers understand what, why, when, how of Lambda expressions. Lambda expressions are nothing but a way to abstract behavior unlike the object-oriented programming which is based upon the abstraction of data in form of object. Lambda expressions are used to assign the behavior to a variable or pass the behavior in between method calls instead of wrapping the behavior in an object and working with the objects. Take a look at the following example to understand the Lambda expressions better.Remember, the famous addActionListener method on button to capture the button-click event and, take action when the …
5 Tricky Interview Questions for Java ArrayList
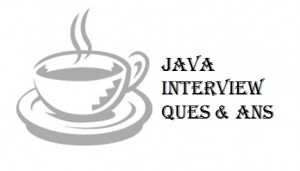
I have been involved in many a java interviews and following are five tricky ones where I found several junior to mid-level Java developers faltering once in a while. Thus, I thought to put an article around these questions to help junior Java developers make familiar with these questions. The article presents the 5 tricky interview questions in relation with Java ArrayList, I believe, could get the interviewee score some browny points, if answered well. Let me know if you agree and would like to add another set of questions to the list below: How does the size of Arraylist increases automatically? Could you share the code? This is the most …
6 Java Exceptions that Haunts a Newbie Java Developer
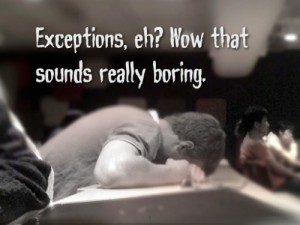
Every now and then, I come across various newbies Java developers who are found to get stuck with some of the following common exceptions where I need to explain them all over again. I do believe that this is same with many other senior Java developers who try and help these rookie developers to deal with following exceptions. Thus, I thought of coming up with this article and use it for myself going forward. Please feel free to comment or add to the below exceptions list. NoClassDefFoundError: This is one of those exception, with message such as Exception in thread “main” NoClassDefFoundError, that has been most commonly found to welcome newbie Java developers to …
3 Java 7 Features Aimed to Enhance Code Quality
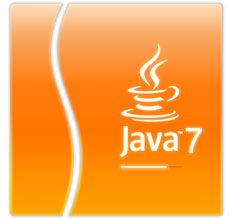
There are several new features that have been released in Java 7 to take care of several developers’ requirements. However, interestingly, I have figured out that three of those 7 features are primarily aimed to enhance following key characteristics of code quality. Please feel free to share your opinion on them. Code Readability Code Duplication Code Complexity Following is the detail of those 3 Java 7 features: Underscore in Numeric Literals (Enhances Code Readability) This feature primarily takes care of code readability, and hence, code usability, having large numbers having several digits. For example, credit card number, social security number etc. Many a times, we come across large number which seem to have an embedded …
I found it very helpful. However the differences are not too understandable for me