Category Archives: Java
AngularJS – How to Get Data from AJAX & Spring MVC
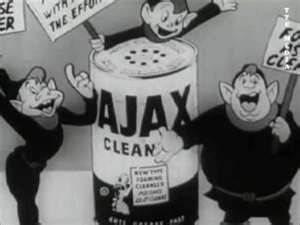
The article presents steps one need to code in order to get data from server using XMLHttpRequest (XHR) while working with Spring MVC Java web application. Watch the demo and code samples at http://hello-angularjs.appspot.com/angularjs-http-service-ajax-get-code-example. Following are key steps: Write two methods in Spring MVC controller, one to load the page and other to serve AJAX request Write AngularJS code to get the data (model) in the controller method using $http Write the view to display the data Spring MVC Controller Methods Following are two methods one need to write in the Spring MVC Controller: Method below serves the page, views/httpservice_get.jsp when accessed using the following URL: http://hello-angularjs.appspot.com/angularjs-http-service-ajax-get-code-example. Try …
Eclipse Key Shortcuts for Greater Developers Productivity
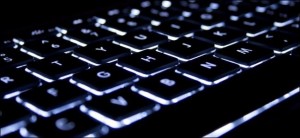
The article presents Eclipse Key Shortcuts (for Windows) which could be used to perform most common coding tasks in a much efficient/faster and effective manner thereby enhancing overall productivity of the Java developers. Please note there are lot more key shortcuts which could be accessed from Eclipse IDE Windows/Preferences/Keys. However, I have made a mention of only those shortcuts which I found very useful in coding faster. If I missed on any shortcuts keys that you feel would be useful to be added in the list below, please give a shout. There were some useful feedbacks on reddit post from where I took some commands and added to the list …
Template Project for Spring MVC 4 & Eclipse Dynamic Web Project
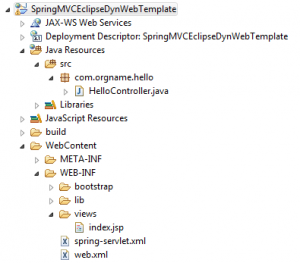
The article presents information, instructions and a downloadable eclipse dynamic web project that one could import in his/her Eclipse IDE and quickly get started with Spring MVC Hello World project. Web Application Folder Structure Following is the Eclipse dynamic web project folder structure based on which files in the template are laid out. Important Files Pay attention to some of the following important files: /WebContent/WEB-INF/web.xml: A file that cnsists of configuration related with how web requests will be handled using DispatcherServlet. /WebContent/WEB-INF/spring-servlet.xml: A file that consists of information on component model/lifecycle along with view handling. /WebContent/WEB-INF/views: A folder that consists of JSP files that acts as a view and referred …
Spring Data MongoDB Hello World with Spring MVC – Example
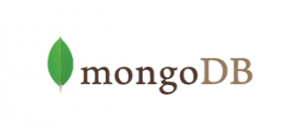
The article presents detailed steps on what is needed to get started with Spring Data MongoDB while you are working with Spring MVC web application. The article assumes that you have got Spring MVC application setup done. Step 1: Create Documents in MongoDB One could download MongoDB from http://www.mongodb.org/downloads page. Once downloaded, do the following to get started. Open a command prompt & goto bin folder found within MongoDB root folder. Before starting MongoDB server, create the data directory within root folder. Start the MongoDB server with command such as “mongod -dbpath <path-to-mongodb-root-folder>” Open another command prompt and goto bin folder. Execute “mongo” command and you are all set. Access …
Spring MVC Web.xml & Spring-Servlet.xml – Code Example
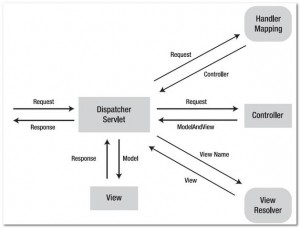
The article presents information around two key configuration files and code samples that one could pickup, put in their web application folder and get up and running. What & Why Web.xml? Web.xml is a deployment descriptor file. Simply speaking, see web.xml as a file used to describe classes, resources and configurations which is used by web server to serve the requests. As the request reaches to the web server, the server uses web.xml to map the URL of the request to the code that would handle the request. While working with Spring MVC, the server, in turn, delegates the request to DispatcherServlet which retrieves appropriate controller that would be used …
How to Configure SpringLoaded with Eclipse Dynamic Web Project
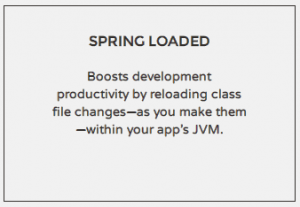
The article presents simple steps to use SpringLoaded framework to have your classes loaded onto the tomcat server without you having required to restart the server, while you are working with Eclipse Dynamic Web Project. The instructions below applies when you have installed Tomcat server and added the same to the eclipse. Quick Introduction on SpringLoaded SpringLoaded, a framework published by Spring.io, is a JVM agent for reloading class file changes whilst a JVM is running. It transforms classes at loadtime to make them amenable to later reloading. Read further on this github page. Personally, I have found it very useful as I do not have to build and restart servers …
How to Gradle Spring MVC Web Project
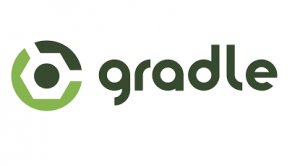
The article describes steps that are required to build the a Spring MVC web application project using Gradle tool. Step 1: Web Application Folder Make sure you have maven-based web application folder. We recommend you to check our article published on different possible layouts of web application folders. As a recap, following is how the web application folder structure would look like: src/main/java (Consists of Java files) src/main/resources src/main/scripts src/main/webapps: This would further have following folder structure: assets (publicly accessible files) css js images META-INF WEB-INF lib (spring/hibernate & other libraries) views (jsp files) hibernate.cfg.xml (if hibernate is used as well) spring-servlet.xml web.xml Step 2: Create a Gradle Script …
Google Datastore Query Get By ID & Filter – Code Example
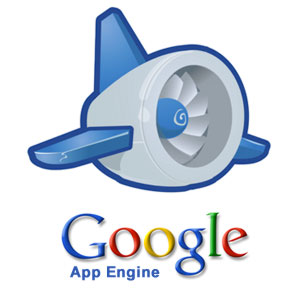
Following are code samples on Google App Engine Datastore Query and how to get entities by id and based on filters. Get Entity By Id Pay attention to the code “datastore.get(KeyFactory.createKey( “savedreport”, reportId). “savedreport” is the name of entity. DatastoreService datastore = DatastoreServiceFactory.getDatastoreService(); Entity entity = null; try { entity = datastore.get(KeyFactory.createKey(“savedreport”, reportId)); } catch(EntityNotFoundException e) { e.printStackTrace(); } Get Entity By One Filter Pay attention to “setFilter” method Filter createdByFilter = new FilterPredicate(“created_by”, FilterOperator.EQUAL, userId ); Query query = new Query(“sqm”).setFilter( createdByFilter ); DatastoreService datastore = DatastoreServiceFactory.getDatastoreService(); List entities = datastore.prepare(query).asList( FetchOptions.Builder.withLimit( count ) ); Get Entity By Multiple Filter Pay attention to usage of multiple FilterPredicate …
Code Example – User Authentication with Google App Engine Java Applications
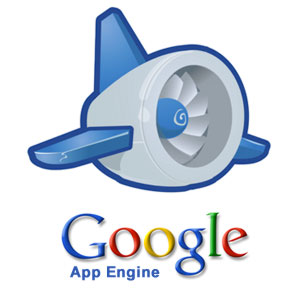
The article presents quick code samples that could be used to adopt Google User authentication service. These strategies have been used in the google app, http://agilesqm.appspot.com. Following key aspects are presented in this article. Web.xml configuration to restrict access to pages Use Google User service Web.xml configuration to restrict access to pages With following code in web.xml, one could restrict access to one or more pages in matter of no time. You could try on how below configuration works by accessing the page, http://agilesqm.appspot.com/createreport and you would be sent to Google login if not yet logged in on the browser. <security-constraint> <web-resource-collection> <web-resource-name>createreport</web-resource-name> <url-pattern>/createreport</url-pattern> </web-resource-collection> <auth-constraint> <role-name>*</role-name> </auth-constraint> <user-data-constraint> …
7 Reasons for Java Developers to Adopt Google App Engine
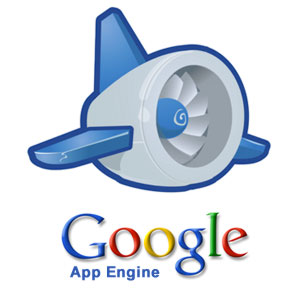
The article represents top 7 reasons why one would want to adopt Google App Engine to do quick Java applications. If you are a bunch of java developers who wants to try out your idea and give a demo to a select group of users, I would recommend you to try out google app engine. Following are top 7 reasons: Eclipse IDE Support As Google supports Eclipse IDE plugin for working with google app engine, it is very helpful to get started quickly in no time. All that is required is download Google App Engine plugin within your Eclipse and start immediately by creating a Google web application. You would find …
Code Example – How to Make AJAX Calls with Java Spring MVC
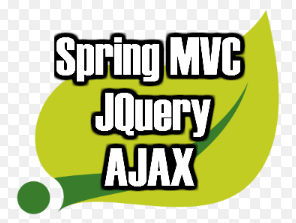
This article is aimed to provide quick code samples to rookies who would like to quickly get started with AJAX while working with Spring MVC based web application. In example below, the AJAX call is made on form submit to retrieve data from server and process it further. Step 1: Get JQuery Library Download JQuery library and place it in JS folder within one of your web assets folder. Even simpler, include following google script code and you are all set. Step 2: Create a Form Create a form such as following that would be dealt in this example. Step 3: Create AJAX Code Step 3 is about …
How to Convert JSON String to Google Datatable for Google Charts
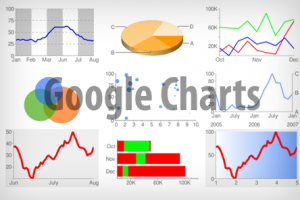
The article presents the solution to some of the issues that I faced while converting JSON string to Google Datatable. The primary reason for me to write an article on seemingly looking trivial issue is that I ended up spending lot of time in doing research and reaching to the solution. Problem Scenario/Issues One AJAX request is made to the server to retrieve the JSON data and draw the Google chart (LineChart in this example) using this JSON data. Following is how the JSON data looks like after being sent from the server-side code: {cols: [ {id: ‘task’, label: ‘Task’, type: ‘string’}, {id: ‘hours’, label: ‘Hours per Day’, type: …
Code Samples to get started with Google Charts & Visualization APIs
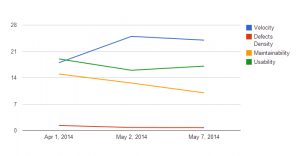
The article describes on some of the aspects related with Google Visualization APIs and how to quickly get started with it. To be able to do justice with this blog and more related blogs to come in near future, I went ahead and create a project, AgileSQM, on Google App Engine (cloud) and used following technologies to create a sample chart as shown below: Google App Engine as platform with Jetty as underlying web server Google NoSQL Datastore Google Visualization APIs Spring MVC (Component model) Bootstrap (UI framework) Eclipse IDE (with Google App Engine platform) Helpful Bookmarks on Visualization APIs While getting started with Google Visualization APIs and working on …
Spring MVC Web with Google NoSQL Datastore on GAE Cloud
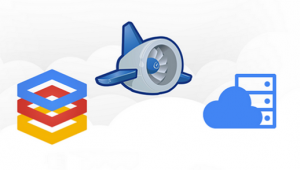
The article represents key aspects of integrating your Spring MVC web application with Google NoSQL Datastore while working on Google App Engine cloud computing platform. Additionally, it presents code samples for you to get started in a quick manner. You may access the sample application talked about in this article on this page, Welcome to GAE World! Following has been discussed: High-level architecture & design Setup NoSQL Data Model (NoSQL data entity vis-a-vis Google Datastore) Implementation including code samples such as following: Controller (HelloController) JSP (hello.jsp) DAOs (GoogleDSCommentDAO) High-level Architecture & Design Following are key technologies used for the implementation discussed in this article: Spring MVC Google Datastore (NoSQL Database) …
5 Steps to Get Spring MVC Web Application on Google App Engine
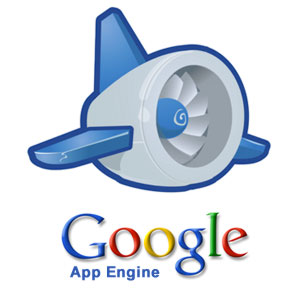
The article represents steps on what you need to do to get your first Spring MVC Hello World web application project on Google App Engine (GAE). As a pre-requisite, we recommend you to check our earlier article on How to get started with Google App Engine. The article would help you to quickly get your started with GAE based web development using Eclipse IDE. We shall work with our existing Non Spring MVC project (check this page) and convert it into Spring MVC based web application. Step 1: Spring MVC Libraries Get Spring MVC libraries within folder (war/WEB-INF/lib). Also, do not forget to get following two runtime dependent libraries: commons-logging-1.1.3.jar …
How to Get Started with Google App Engine Java Project
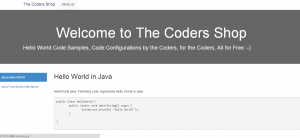
The article presents quick tips to get started with your next Java Web Application on Google App Engine (GAE). I was able to create my first web application on Cloud (GAE) named as “The Coders Shop”, in couple of hours, however, with little hiccups which I have mentioned in this article. Pre-requisites Following are pre-requisites to get started quickly: Create a Google account. This would be used to create an App Engine Id that would represent your web application on the web. For an app engine id, xyz, the web URL of your application will be xyz.appspot.com. You could alternatively create App Engine Id right from within Eclipse shown below. …
I found it very helpful. However the differences are not too understandable for me