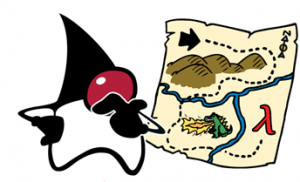
Calculator Implementation Demonstrating Lambda Expressions
The Calculator methods implementation would be explained using both, traditional approach and the approach making use of Lambda expressions.
Traditional Approach
Using traditional approach, many would have gone implementing Calculator add, substract, multiply and divide function based on following:
- Define a Calculator interface with four methods namely add, subtract, multiply and divide; Another approach could be to create a single method with an operation flag and provide conditional implementation based on flag.
- Create a CalculatorImpl class implementing Calculator interface and providing implementation for each method. Simply speaking, these methods would have code such as a + b, a – b, a*b and a/b.
Another approach would have been based on anonymous class displayed in example below. However, it only struck me when I was writing code for Lambda expressions.
Lamba Expressions
With Lambda expressions in Java 8, the same could be achieved in following manner.
Calculator.java (Interface)
Following can be termed as functional interface having just one method. The important point to note is that there is no need of writing separate methods. Also, one may not even need any conditional flag of any sort to execute different code for different operations.
public interface Calculator {
public Double calculate( Double num1, Double num2 );
}
HelloCalculator.java (Class)
public class HelloCalculator {
public double process( double num1, double num2, Calculator calculator ) {
return calculator.calculate( num1, num2 );
}
public static void main(String[] args) {
HelloCalculator hl = new HelloCalculator();
// Traditional Way using Anonymous class
System.out.println("Addition: " + hl.process(3, 4, new Calculator() {
public Double calculate(Double num1, Double num2) {
return num1 + num2;
}
}));
// Lambda Way; How simplified the code became
Calculator calcSubtraction = (Double num1, Double num2) -> {
return num1 - num2;
};
System.out.println("Subtraction: " + hl.process(3, 4, calcSubtraction));
// Lambda Way; Further simplification using Type Inference
System.out.println("Multiplication: "
+ hl.process(3, 4, (num1, num2) -> {
return num1 * num2;
}));
System.out.println("Divide: " + hl.process(3, 4, (num1, num2) -> {
return num1 / num2;
}));
}
}
Calculator Implementation Demonstrating Lambda Expressions & Functional Interfaces
Using Out-of-box functional interfaces from java.util.function package, one could simply implement Calculator operations such as add, subtract, multiply and divide without having need to define any Calculator interface. Take a look at the code below.
Isn’t that very neat?
import java.util.function.BiFunction;
import java.util.function.BinaryOperator;
public class HelloCalculatorUsingBiFunction {
public Long process( long num1, long num2, BiFunction biFunc ) {
return (Long) biFunc.apply( num1, num2);
}
public static void main(String[] args) {
HelloCalculatorUsingBiFunction hlbf = new HelloCalculatorUsingBiFunction();
BinaryOperator add = (x, y) -> x + y;
System.out.println( "Addition: " + hlbf.process( 4, 5, add ));
BinaryOperator subtract = (x, y) -> x - y;
System.out.println( "Subtraction: " + hlbf.process( 4, 5, subtract ));
BinaryOperator multiply = (x, y) -> x/y;
System.out.println( "Multiplication: " + hlbf.process( 4, 5, multiply ));
BinaryOperator division = (x, y) -> x/y;
System.out.println( "Division: " + hlbf.process( 4, 5, division ));
}
}
For another perspective on introduction to Lambda expressions, check out our article on this page.
[adsenseyu1]
- Mathematics Topics for Machine Learning Beginners - July 6, 2025
- Questions to Ask When Thinking Like a Product Leader - July 3, 2025
- Three Approaches to Creating AI Agents: Code Examples - June 27, 2025
hi, please correct “->” into “->” in your source example 🙂
thanks for the suggestion.
Pretty component of content. I simply stumbled upon your blog and in accession capital to say that I
acquire actually enjoyed account your blog posts. Anyway I’ll be subscribing in your feeds and
even I success you access constantly quickly.