Category Archives: Web
Java – How to Scrape Web using Multi-threading (ExecutorService)
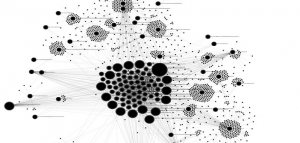
This article represents code examples on how to Scrape multiple URLs at once using Java Multi-threading API such as ExecutorService. The sole reason why I have been doing scraping lately is the need to get data from web to apply data analytics/science (machine learning algorithms) and extract knowledge from the data. Please feel free to comment/suggest if I missed to mention one or more important points. Also, sorry for the typos. Following are three different methods whose code samples have been presented below: scrapeURLs that takes input file consisting of URLs to be scraped and output file where the output needs to be written scrapeIndividualURLs which takes as an argument, URL …
Java – How to Create Binary Search Tree for String Search
This article represents code samples and high level concepts for creating a binary search tree to do String related operations such as some of the following: Search one or more words Replace word with new word Find number of occurences of a given word in a string For those who are new to Binary Search Tree, note that Binary Search Tree is defined as tree that satisfy some of the following criteria: Each node in the tree has at most only two children Each node is represented with a key and associated data Key in left children is less than the parent node and key in the right node is …
Java – How to Create a Binary Search Tree
This article represents the high level concept and code samples which could be used to create a binary search tree in Java. Please feel free to comment/suggest if I missed to mention one or more important points. Also, sorry for the typos. Following are the key points described later in this article: What is a binary search tree? What are different kind of traversals? Code Samples What is a binary search tree? A binary search tree is a binary tree in which every node contains a key that satisfies following criteria: The key in left child is less than the key in the parent node The key in the right …
Java – One-Liner Lambda Expression to Print Map/List Objects
This article represents code samples to print element of collections such as Map and List/Set using lambda expression. I just love it as it has made life so simpler by helping me achieve this using just one line of code. Please feel free to comment/suggest if I missed to mention one or more important points. Also, sorry for the typos. Following are the key points described later in this article: Print Map key-Value Pair using Lambda Expression Print List value using Lambda Expression Print Map key-Value Pair using Lambda Expression Pay attention to forEach function that takes a reference to BiConsumer function interface and prints the key and value. This …
Java – Map & BiConsumer Function Lambda Expression Example
This article represents code samples representing lambda expression and the related ease with which one could print key and value of a Map object using one liner. Please feel free to comment/suggest if I missed to mention one or more important points. Also, sorry for the typos. Code Sample – Printing Map using BiConsumer Functional Interface Following is detail for Map.forEach API in Java 8. Read further on this page. default void forEach(BiConsumer<? super K,? super V> action) Performs the given action for each entry in this map until all entries have been processed or the action throws an exception. Unless otherwise specified by the implementing class, actions are performed in …
Java – How to Scrape Content from a URL
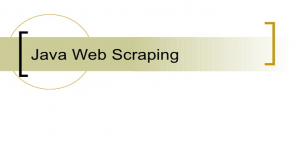
This article represents take-away code sample that could be used to get or scrape content from a given URL. Those wanting to do a quick web scrape could use this piece of code. I shall be posting a series of blogs which would help one to create a web scraper using Java. The reason why I am hooked to Java web scraping is the need to get the data from web for data analysis. Please feel free to comment/suggest if I missed to mention one or more important points. Also, sorry for the typos. Code Sample – Get Content from URL Pay attention to some of the following aspects of fetching …
Productivity – Sublime, AngularJS, Bootstrap Form Template
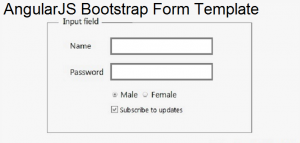
This article represents sublime text editor snippet code sample for an AngularJS-Bootstrap form template which could be used to quickly create an angular app with a simplistic bootstrap form. Please feel free to comment/suggest if I missed to mention one or more important points. Also, sorry for the typos. Following are the key points described later in this article: Why AngularJS-Bootstrap Form Template? How to Create Sublime Snippet? Code Samples – AngularJS-Bootstrap Form Template Why AngularJS-Bootstrap Form Template? Many a times, I wanted to experiment with AngularJS features on an HTML form. This required me to re-write or copy & paste angularjs code and bootstrap form code and create …
AngularJS – Sublime Template for Hello World
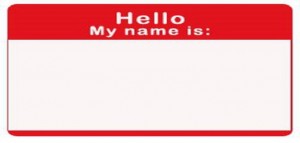
This article represents sublime snippet code sample which you could use to create an auto-complete hello world template to quickly get started with Hello World code for AngularJS. Please feel free to comment/suggest if I missed to mention one or more important points. Also, sorry for the typos. Following are the key points described later in this article: Why Sublime Snippet for AngularJS Hello World How to Create HelloNG Snippet? Code Samples Why Sublime Snippet for AngularJS Hello World Many a time, while starting on new AngularJS app for doing quick POC or testing purpose, I ended up creating boilerplate code or copied and pasted minimum AngularJS app code …
ReactJS – Online Playground for JSX-HTML Expertise
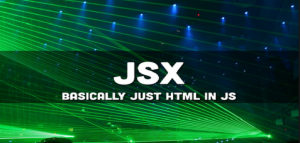
This article represents quick introduction to online playground for JSX to HTML conversion and vice-versa. Very handy for those, especially the Javascript beginners, to get a hang on component-oriented programming related with ReactJS which is at the heart of it. Please feel free to comment/suggest if I missed to mention one or more important points. Also, sorry for the typos. Following are the key points described later in this article: HTML to JSX Compiler JSX to HTML Compiler Following are two online tool or playground to enhance familiarity with JSX. HTML to JSX Compiler: This Online playground for HTML-JSX compiler would help you to write HTML on one end, …
AngularJS – How to Handle XSS Vulnerability Scenarios
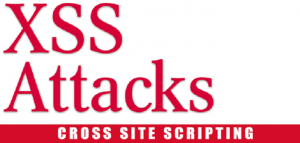
This article represents different scenarios related with XSS (cross-site scripting) and how to handle them appropriately using AngularJS features such as SCE ($sceProvider) and sanitize service ($SanitizeProvider). Please feel free to comment/suggest if I missed to mention one or more important points. Also, sorry for the typos. Do visit the page, how to prevent XSS attacks in Angular 2.*, Angular 4.* or Angular 5.*, if you are looking forward for handling XSS vulnerabilities in latest version of Angular apps. You may also want to check the page, Top 10 Angular Security Best Practices vis-a-vis vulnerability issues. Following are the key XSS-related scenarios described later in this article: Escape HTML completely …
AngularJS – Two Ways to Initialize an Angular App
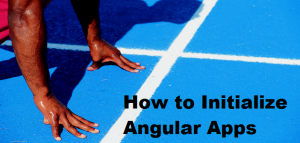
This article represents code samples along with related concepts for two different ways in which Angular app can be defined. Please feel free to comment/suggest if I missed to mention one or more important points. Also, sorry for the typos. Following are the key points described later in this article: Automatic Initialization Manual Initialization Automatic Initialization All that is needed for automatic initialization (as of current AngularJS version) is to define “ng-app” on an element and you should be all set. Take a look at following code sample. Pay attention to some of the following: ng-app=”HelloApp” defined on div element ng-controller=”HelloCtrl” defined on the same element. It could as …
Java – Top 10 Java-based Web Frameworks for 2014-2015
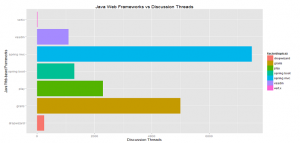
This article represents an analysis on Java-based web frameworks that emerged in the top 10 list this year 2014, and worth consideration for your next project starting this year or next year (2015). I have done data analysis based on following: Job openings (as of today) on a very popular website, indeed.com Discussion threads (for this year) on a very popular Q&A based website, stackoverflow.com Responses on a very popular social bookmarking website, reddit.com Based on the analysis of top 10 frameworks, I have listed the top 5 frameworks which emerged as clear winner. Please feel free to comment/suggest if I missed to mention one or more important frameworks. Also, …
Productivity – Top 3 Javascript IDEs/Text Editors to Consider
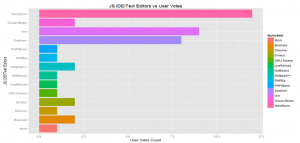
This article represents the top 3 Javascript IDE/Text Editors that you may want to explore for your next project for Javascript related development. The way the top 3 editors are chosen is the number of votes (thumbs up) given by different users in one of the Javascript IDE/Text Editor related discussion thread on one of the very popular social bookmarking website, as of today. Interestingly, newer editors such as Brackets and Atom are catching attention of some of the users. Please feel free to comment/suggest if I missed to mention one or more important points. Also, sorry for the typos. Following are different Javascript Text Editors (at times, also called …
Javascript Frameworks Job Pattern with IT Companies in India
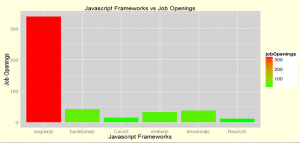
This article represents my analysis on job patterns vis-a-vis javascript frameworks with IT companies in India. This may also be read as a pattern of adoption of JS frameworks by developers community with IT companies in India. The analysis has been done based on number of job openings related with below listed JS frameworks, posted last month e.g., octobar 2014, on one of the topmost job portal catering to Indian IT market. Please feel free to comment/suggest if I missed to mention one or more important points. Also, sorry for the typos. Following are top 7 javascript frameworks which are most seeked after skillsets in potential UI related candidates, from …
AngularJS – Top 6 Concepts That Developers Loved
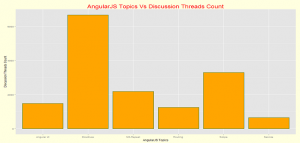
This article represents top 6 popular AngularJS topics that has been used most by the AngularJS developer community till date. The inference is derived based on number of tagged discussions happening on Stackoverflow. Clearly, “Directive” is the winner and attracts most of them all. The article presents my thoughts on why these topics have been most popular. Please feel free to comment/suggest if I missed to mention one or more important points. Also, sorry for the typos. Following is the list of top 6 popular topics: Directives Scope Object Ng-repeat Angular UI & Bootstrap Routing Service Following plot demonstrates the popularity of different feature/topics in relation with AngularJS. …
AngularJS – Hello World with Angular-Seed – Code Example
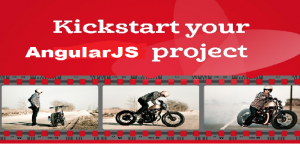
This article introduces Angular-Seed project for AngularJS beginners and, presents a code example along with instructions to get started with Angular-Seed project. Please feel free to comment/suggest if I missed to mention one or more important points. Also, sorry for the typos. Following are the key points described later in this article: What is Angular-Seed project? Pre-requisites for installing Angular Seed Project Install and Configure Angular-Seed Project Hello World – Code Example If you are an AngularJS beginner or have started developing angular apps and, have been wondering about the standard folder structure layout to put your HTML, CSS and JS files, you would want to consider Angular-Seed project. …
I found it very helpful. However the differences are not too understandable for me