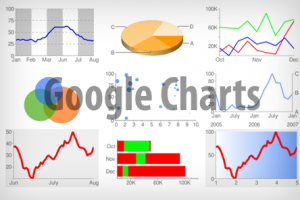
Problem Scenario/Issues
One AJAX request is made to the server to retrieve the JSON data and draw the Google chart (LineChart in this example) using this JSON data.
Following is how the JSON data looks like after being sent from the server-side code:
{cols: [
{id: 'task', label: 'Task', type: 'string'},
{id: 'hours', label: 'Hours per Day', type: 'number'}],
rows: [
{c:[{v: 'Work'}, {v: 11}]},
{c:[{v: 'Work'}, {v: 11}]},
{c:[{v: 'Eat'}, {v: 2}]},
{c:[{v: 'Commute'}, {v: 2}]},
{c:[{v: 'Watch TV'}, {v:2}]},
{c:[{v: 'Sleep'}, {v:7, f:'7.000'}]}
]}
Following is the AJAX & Javascript code which was written to achieve above mentioned scenario. However, the problem was that chart was not showing up.
$.ajax({
url : $("#displayReportsForm").attr("action"),
data : data,
type : "GET",
success : function(response) {
google.setOnLoadCallback(drawChart(response));
},
error : function(xhr, status, error) {
alert(xhr.responseText);
}
});
function drawChart(jsonData) {
var containerId = "divId"; //Id of a div container within HTML to hold the chart
try {
var data = new google.visualization.DataTable(jsonData);
var chart = new google.visualization.LineChart(document
.getElementById(containerID));
chart.draw(data, options);
} catch (err) {
alert( err.message );
}
}
On one of the related pages on stackoverflow, a problem was stated where the same jsonData was locally done like following, but the chart did not show up.
jsonData = "{cols: [{id: 'task', label: 'Task', type: 'string'},{id: 'hours', label: 'Hours per Day', type: 'number'}],rows: [{c:[{v: 'Work'}, {v: 11}]},{c:[{v: 'Work'}, {v: 11}]},{c:[{v: 'Eat'}, {v: 2}]},{c:[{v: 'Commute'}, {v: 2}]},{c:[{v: 'Watch TV'}, {v:2}]},{c:[{v: 'Sleep'}, {v:7, f:'7.000'}]}]}"
Solutions
Following could be used to resolve the above-mentioned issues and get the chart show up.
1. If testing locally, DO NOT enclose jsonData within “” as shown above. If you would remove outer double quotes (“”) sign, it would work fine.
2. For the above method to work correctly, one would require to write following additional code within the method.
jsonData = JSON.stringify(eval("(" + jsonData + ")"));
Thus, the method would look like following:
function drawChart(jsonData) {
jsonData = JSON.stringify(eval("(" + jsonData + ")"));
var containerId = "divId"; //Id of a div container within HTML to hold the chart
try {
var data = new google.visualization.DataTable(jsonData);
var chart = new google.visualization.LineChart(document
.getElementById(containerID));
chart.draw(data, options);
} catch (err) {
alert( err.message );
}
}
- Completion Model vs Chat Model: Python Examples - June 30, 2024
- LLM Hosting Strategy, Options & Cost: Examples - June 30, 2024
- Application Architecture for LLM Applications: Examples - June 25, 2024
Ajitesh – you have saved my sanity – thankyou! I’ve been trying to get this working for hours and your comments helped me resolve my problems