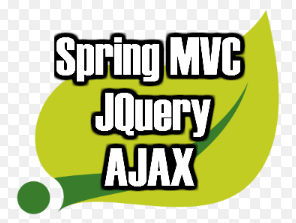
Step 1: Get JQuery Library
Download JQuery library and place it in JS folder within one of your web assets folder. Even simpler, include following google script code and you are all set.
<script src="//ajax.googleapis.com/ajax/libs/jquery/1.11.1/jquery.min.js"></script>
Step 2: Create a Form
Create a form such as following that would be dealt in this example.
<form id="sampleForm" method="post" action="/profile"> <div> <input type="text" name="firstname" id="firstname"> </div> <div> <input type="text" name="lastname" id="lastname"> </div> <div> <button type="submit" name="submit">Submit</button> </div> </form>
Step 3: Create AJAX Code
Step 3 is about creating AJAX code on UI side that would send the request to server. Following is the sample code that needs to be placed within code.
$(document).ready(function() { $('#sampleForm').submit( function(event) { var firstname = $('#firstname').val(); var lastname = $('#lastname').val(); var data = 'firstname=' + encodeURIComponent(firstname) + '&lastname=' + encodeURIComponent(lastname); $.ajax({ url : $("#sampleForm").attr("action"), data : data, type : "GET", success : function(response) { alert( response ); }, error : function(xhr, status, error) { alert(xhr.responseText); } }); return false; }); });
Step 4: Create Server-side Code
Last step is about creating server-side code that would recieve AJAX request, process it and send back the response. Following is the code which should be defined within a controller:
package com.vitalflux.sample; @Controller public class SomeController { @RequestMapping(value = "/profile", method = RequestMethod.GET) public @ResponseBody String processAJAXRequest( @RequestParam("firstname") String firstname, @RequestParam("lastname") String lastname ) { String response = ""; // Process the request // Prepare the response string return response; } }
Make sure that you included following in spring-servlet.xml. Note that package name of above controller is same as that mentioned below:
<context:component-scan base-package="com.vitalflux.sample" />
- Autoencoder vs Variational Autoencoder (VAE): Differences - May 8, 2024
- Linear Regression T-test: Formula, Example - May 7, 2024
- Feature Engineering in Machine Learning: Python Examples - May 3, 2024
superb post, work fine for me.
Superb Ajitesh Kumar….
thanks a lot