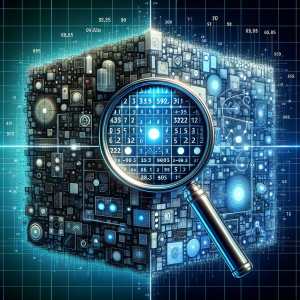
GridSearchCV method is a one of the popular technique for optimizing logistic regression models, automating the search for the best hyperparameters like regularization strength and type. It enhances model performance by incorporating cross-validation, ensuring robustness and generalizability to new data. This method saves time and ensures objective model selection, making it an essential technique in various domains where logistic regression is applied. Its integration with the scikit-learn library (sklearn.model_selection.GridSearchCV) simplifies its use in existing data pipelines, making it a valuable asset for both novice and experienced machine learning practitioners.
How is GridSearchCV used with Logistic Regression?
GridSearchCV is a technique used in machine learning for hyperparameter tuning. It is a method of systematically working through multiple combinations of parameter tunes, cross-validating as it goes to determine which tune gives the best performance. GridSearchCV is part of the scikit-learn library in Python and is widely used for model tuning. It ensures that the model is not just tuned to a specific subset of the data, and it helps in finding the most effective parameters. However, it can be computationally expensive, especially with a large dataset and a vast grid of parameters.
Here’s how GridSearchCV works in the context of logistic regression:
- Defining Parameter Grid: You create a grid of parameters that you want to test. For logistic regression, this might include parameters like C (inverse of regularization strength), penalty (type of regularization, such as L1 or L2), and others.
- Cross-Validation Setup: GridSearchCV uses cross-validation to evaluate each individual combination of parameters. Cross-validation involves splitting the dataset into a number of subsets (or “folds”) and then training and testing the model on these different combinations, which helps in assessing the model’s performance more robustly.
- Searching for Best Parameters: The algorithm fits the logistic regression model on your training data with each combination of parameters in the grid and evaluates the model’s performance using a specified scoring method (like accuracy, precision, recall, etc.).
- Selecting the Best Model: After evaluating all the combinations, GridSearchCV selects the parameters that yield the best performance according to the chosen scoring metric.
- Training the Final Model: Finally, the logistic regression model is retrained using the best parameters on the entire training set.
GridSearchCV Logistic Regression Python Example
In machine learning, optimizing the hyperparameters of a model is crucial for achieving the best performance. Logistic regression, a popular classification algorithm, has several hyperparameters like regularization strength and penalty type that can be tuned for better results. GridSearchCV method in the scikit-learn library automates this process by testing a range of hyperparameter values and selecting the best combination based on cross-validation.
Here’s a Python code example that demonstrates how to use GridSearchCV with logistic regression:
from sklearn.model_selection import GridSearchCV from sklearn.linear_model import LogisticRegression from sklearn.datasets import load_iris from sklearn.preprocessing import StandardScaler from sklearn.pipeline import make_pipeline # Load iris dataset iris = load_iris() X, y = iris.data, iris.target # Create a pipeline with scaler and logistic regression pipe = make_pipeline(StandardScaler(), LogisticRegression(max_iter=1000, solver='saga', tol=0.1)) # Create a parameter grid param_grid = { 'logisticregression__C': [0.1, 1, 10, 100], 'logisticregression__penalty': ['l1', 'l2'] } # Create GridSearchCV object grid_search = GridSearchCV(pipe, param_grid, cv=5) # Fit the model grid_search.fit(X, y) # Print best parameters and best score print("Best Parameters:", grid_search.best_params_) print("Best Score:", grid_search.best_score_)
Here is the explanation of the above Python code:
- Load Dataset: The Iris dataset, a common dataset in machine learning, is loaded for training the model.
- Define Logistic Regression Model: An instance of sklearn LogisticRegression is created.
- Parameter Grid: A grid of hyperparameters to test is defined. Here, C (regularization strength) and penalty (type of regularization) are varied.
- GridSearchCV Object: A GridSearchCV object is created with the logistic regression model, the parameter grid, and the number of folds (cv) for cross-validation.
- Model Fitting: The GridSearchCV object is fitted with the data, which runs the logistic regression model with all combinations of parameters in the grid.
Challenges when using GridSearchCV with Logistic Regression
The most common issues that happen when using GridSearchCV with Logistic Regression is failure to converge. The above code could throw error such as “ConvergenceWarning: lbfgs failed to converge“. This error indicates the logistic regression algorithm did not converge to a solution within the maximum number of iterations allowed. This error can be addressed using the following:
- Increase the Maximum Number of Iterations: By default, the maximum number of iterations in LogisticRegression might be too low for convergence. You can increase this number by setting the max_iter parameter to a higher value.
- Adjust the Regularization Strength: Sometimes, the convergence issue can be due to the regularization strength (C parameter). Experiment with different values for C. A higher value of C means less regularization.
- Feature Scaling: Ensure that your features are on a similar scale. Convergence can fail if features are on widely different scales. Using a scaler, like StandardScaler, can help.
- Solver Selection: If the default solver (‘lbfgs’) isn’t converging, try using a different solver. For instance, ‘saga’ is often a good choice for large datasets and supports both L1 and L2 regularization.
- Tolerance Parameter: Tweaking the tol parameter (tolerance for stopping criteria) might also help. A higher tolerance can lead to earlier stopping.
- Completion Model vs Chat Model: Python Examples - June 30, 2024
- LLM Hosting Strategy, Options & Cost: Examples - June 30, 2024
- Application Architecture for LLM Applications: Examples - June 25, 2024
Leave a Reply