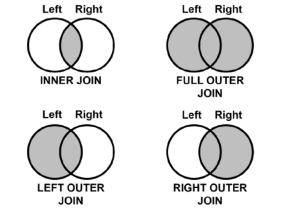
Structured Query Language (SQL) is one of the most important and widely used tools for data manipulation. It allows users to interact with databases, query and manipulate data, and create reports. One of SQL’s most important features is its ability to join tables together in order to enrich, compare and analyze related data. These joins are termed as inner join, outer join, left join and right join. In this article, we will discuss the different types of joins available in SQL, their differences and provide examples of how each can be used.
What is SQL Join?
SQL Joins are a technique used in Structured Query Language (SQL) to combine two separate tables into a single table. This is done by establishing relationships between the tables based on common fields, such as an ID field or a foreign key field. By joining two tables together, you can create a third table that combines information from both tables, enrich the dataset and creates new insights that would otherwise be impossible to obtain individually.
There are four main types of SQL Joins
- Inner join
- Left outer join (or Left join)
- Right outer join (or Right join)
- Full outer join
The following picture represents the most simplistic view of understanding SQL Joins. The left represents one table and the right represents another table. Combining two tables’ results can result in a different view of the data. The operation for combining two tables into a third one is called Join. By combining two tables together, the data is enriched as they get merged to have the information on a specific data entity.
In addition to these four joins, SQL also supports Cross Joins and Self Joins which allow complex data combining operations to be performed quickly and efficiently. Cross Joins allow you to combine every row in one table with every row in another table while Self Joins enable you to join a single table with itself using fields within that same table.
Let’s understand concepts for each of the main SQL joins in a detailed manner.
Inner Join
SQL Inner Join is a type of join operation that combines two tables into one result set. It takes the common information from both tables and returns it in a single row, allowing for more efficient data retrieval. In an inner join, only rows with matching values in both tables will be returned. This is unlike other types of joins such as outer joins, which return all possible matches between the two tables regardless of whether or not they have any common data. The criteria for inner join can be a shared column name between both tables or a predefined condition that must be true for each row returned in the result set. The following diagram / picture represents an example of SQL inner join:
The following is the inner join SQL query that can be help achieve above assuming left table is named as “country_units” and right table is named as “country”.
SELECT country_units.Date, country_units.CountryID, country_units.Units, country.Country FROM country_units INNER JOIN country ON country_units.CountryID = country.ID;
The INNER JOIN clause in the above listed inner join SQL query is used to select all rows from multiple tables as long as there is a match between the columns in these tables. If there are rows in one table that do not have corresponding rows in another, those rows will not be listed in the result set. An INNER JOIN typically requires a join condition. If the join condition is met, the query combines each row from the first table with the row from the second table and includes this row set in the results.
Left Outer Join (or Left Join)
Left Outer Join (LOJ) is an important concept in database design and query optimization. It works by matching rows from one table with those from the other and then combining the matches with rows from the left table, which contains all of the rows regardless of whether there are any matches in the other table. For instance, if you have two tables – one containing customer information and another containing product sales information – an LOJ can be used to return all customers as well as any associated sales data. This allows you to get a complete picture of your customers, even if they haven’t made any purchases yet. This type of join is useful because it allows developers to easily retrieve data related to records in one table even if there are no corresponding records in another table. The following diagram / picture below represents an example of joining two tables based on the left outer join:
The following represents the left outer join SQL query which can be written to achieve the above shown:
SELECT country_units.Date, country_units.CountryID, country_units.Units, country.Country FROM country_units LEFT OUTER JOIN country ON country_units.CountryID = country.ID;
The LEFT OUTER JOIN clause in above SQL query returns all rows from the left table (the table before the JOIN keyword), along with the matching rows from the right table (the table after the JOIN keyword). If there is no match, the result is NULL on the right side.
Left Outer Join is particularly advantageous when working with multiple tables that have a hierarchical relationship. For example, say you have three tables containing customer information: Customers, Orders, and Order Details. The Customers table contains general customer data such as name and address; the Orders table contains customer orders; and the Order Details table contains information about each order item such as product number, quantity, and price. With Left Outer Join, you can quickly pull all customer-related information into a single result set without needing to query each individual table separately.
In addition to providing an efficient way to combine multiple data sources into a single result set for reporting or analytics purposes, Left Outer Joins can be used for error detection purposes as well. By performing this type of join on two tables, any values that appear in one but not in the other can be quickly identified and addressed if necessary. This makes it easier for developers to catch errors early on before they become bigger problems down the line.
Right Outer Join (or Right Join)
Right Outer Join is a type of join used in relational databases that merges two tables and returns all the records from the right table, as well as the matched records from the left table. It is useful when trying to combine data from multiple sources in order to gain a better understanding of how they interact. The following picture / diagram provides an example of how the right outer join works.
The following represents the right outer join SQL query which can be written to achieve the above:
SELECT Customers.customer_id, Customers.first_name, Orders.amount FROM Customers RIGHT OUTER JOIN Orders ON Orders.customer = Customers.customer_id;
The syntax for a right outer join SQL query involves specifying two tables after the “FROM” statement followed by the RIGHT OUTER JOIN clause and then an optional condition on which both tables should match their respective columns after the ON clause. The result set will contain all records from a second (right) table, plus those records that match the given criteria from the first (left) table. Depending on how many tables are involved in the query and their size this can yield more efficient performance than a full outer join since it does not require combining records from multiple tables first before filtering out unnecessary ones.
Full Outer Join
Full Outer Join is a type of join in SQL Join that combines the results of both the left and right outer joins. It includes rows that are present in either one or both of the tables used in the join. The result set contains all joined records, including duplicates. This type of join is useful when we need to find all records from both tables regardless if they have matching data or not. The following diagram / picture provides an example of how the full outer join works.
The following represents the full outer join SQL query which can be written to achieve the above shown:
SELECT country_units.Date, country_units.CountryID, country_units.Units, country.Country FROM country_units FULL OUTER JOIN country ON country_units.CountryID = country.ID;
The FULL OUTER JOIN clause in above SQL query returns all rows when there is a match in either the left or right table. This means it combines the results of both left and right outer joins. Where there is no match, the result is NULL on the side of the table that has no match.
The primary purpose of a full outer join is to identify differences between two sets of data by combining them into one common set. This can be quite useful for comparing large datasets quickly and efficiently. For example, say you have two tables; one containing customer information and the other containing sales information. When using a full outer join, this query will return all customers and their corresponding sales orders if available and also display any customers who do not have any sales orders associated with them as well as any sales orders which do not have an associated customer record.
Full outer join can also help with performance optimization when dealing with large datasets because it only requires reading each table once, rather than multiple times when performing separate inner joins on the same tables.
Frequently Asked Questions (FAQs)
The following are some of the most popular FAQs in relation to SQL joins:
- What’s the difference between Inner join vs Left join or Left outer join?
- An INNER JOIN retrieves records that have matching values in both tables involved in the join, effectively returning the intersection of the two tables. A LEFT JOIN (or LEFT OUTER JOIN), on the other hand, returns all records from the left table, and the matched records from the right table; if there is no match, the result from the right table will be NULL. Essentially, an INNER JOIN gives you the overlap, while a LEFT JOIN ensures all records from the left table are included, matched or not.
- What’s the difference between Inner join vs Outer join?
- An INNER JOIN returns rows where there is a match in both joined tables. Conversely, an OUTER JOIN (which can be a LEFT, RIGHT, or FULL OUTER JOIN) returns all rows from one or both tables regardless of a match. Specifically, a LEFT OUTER JOIN returns every row from the left table, and a RIGHT OUTER JOIN from the right table, with NULLs for non-matching rows from the opposite table. A FULL OUTER JOIN combines the results of both left and right outer joins, including all records from both tables.
- What’s the difference between Inner join vs Full Outer join?
- An INNER JOIN selects records that have matching values in both tables, so only the rows with a match in both tables are returned. In contrast, a FULL OUTER JOIN selects all records from both tables, regardless of matches. Where there is no match, the result set will have NULL values for every column from the table that lacks a corresponding match. In essence, an INNER JOIN combines rows with matching data, while a FULL OUTER JOIN combines all rows from both tables, matching or not.
- What’s the difference between Left Outer join vs Right Outer join?
- A LEFT OUTER JOIN (or Left Join) returns all records from the left table and the matched records from the right table. If there’s no match, the result set will include NULL values for the right table’s columns. A RIGHT OUTER JOIN (or Right Join), conversely, returns all records from the right table and the matched records from the left table, with NULL values in the result set for unmatched columns from the left table. Essentially, a LEFT OUTER JOIN prioritizes the left table, and a RIGHT OUTER JOIN prioritizes the right table in terms of which rows are included in the result set.
- What’s the difference between Right join vs Right Outer join?
- They are one and the same thing. Right join and Right Outer Join are used interchangeably.
- What’s the difference between Left Outer join vs Left join?
- They are one and the same thing. Left join and Left Outer Join are used interchangeably.
Conclusion
SQL Joins are used to connect two or more relational database tables. The main purpose of using SQL joins is to retrieve data from multiple tables and combine it into a single result set. There are four different types of SQL joins: inner join, outer join, left outer join, and right outer join. Each type of join has its own use case. Depending on your particular needs, you will need to choose the right type of SQL joins for your query in order to get the desired results. If you have any questions about which type of SQL join to use in your situation, please reach out for a detailed conversation.
- Completion Model vs Chat Model: Python Examples - June 30, 2024
- LLM Hosting Strategy, Options & Cost: Examples - June 30, 2024
- Application Architecture for LLM Applications: Examples - June 25, 2024
Leave a Reply