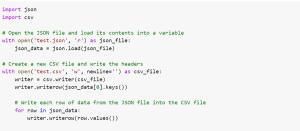
Have you ever wondered how to convert JSON data to CSV using Python? JSON (JavaScript Object Notation) is a popular data format used to exchange data between servers and web applications. However, sometimes it’s necessary to convert this data into another format, such as CSV (Comma Separated Values). CSV is a simple text format that is commonly used to store and exchange tabular data.
In this blog post, a sample Python code is provided for converting JSON to CSV using Python. The code showcases the Python code that uses the json and csv modules to read and write data. But before going forward with the code, let’s take a look at what the JSON dataset looks like and how the CSV file would be structured after the conversion.
The JSON dataset that is used for the demonstration purpose is as follows:
[
{"name": "Ajjitesh", "percentage": 90.5, "section": "A"},
{"name": "Nidhi", "percentage": 94.4, "section": "B"}
]
This dataset contains two rows of data, where each row has a name, percentage, and section (class). The percentage is a floating-point number, and the other two fields are strings.
Python Code for Converting JSON to CSV
The following Python code converts the above given JSON data into a CSV file.
import json
import csv
# Open the JSON file and load its contents into a variable
with open('test.json', 'r') as json_file:
json_data = json.load(json_file)
# Create a new CSV file and write the headers
with open('test.csv', 'w', newline='') as csv_file:
writer = csv.writer(csv_file)
writer.writerow(json_data[0].keys())
# Write each row of data from the JSON file into the CSV file
for row in json_data:
writer.writerow(row.values())
Note some of the following in the above code:
- Necessary modules such as json and csv are imported
- with statement is used to open the JSON file and load its contents into a variable using the json.load function
- A new CSV file is created using the open() function and csv.writer() function.
- writerow() method is used to write the header row to the CSV file. The header row contains the keys from the first row of the JSON data.
- The for loop is used to write each row of data from the JSON file into the CSV file using the writerow() method
The resulting CSV file will contain the following:
name,percentage,section
Ajjitesh,90.5,A
Nidhi,94.4,B
Each row of the CSV file contains the values from the corresponding row of the JSON data. The first row of the CSV file contains the headers, which are the keys from the first row of the JSON data. The values in each row of the CSV file are separated by commas.
Conclusion
Converting JSON data to CSV is a crucial skill for anyone working with data processing and analysis. Python provides powerful modules for working with both JSON and CSV data, making it easy to convert between the two formats. With the code and explanation provided in this blog post, I hope you should now have a good understanding of how to convert JSON to CSV using Python.
- Completion Model vs Chat Model: Python Examples - June 30, 2024
- LLM Hosting Strategy, Options & Cost: Examples - June 30, 2024
- Application Architecture for LLM Applications: Examples - June 25, 2024
Leave a Reply