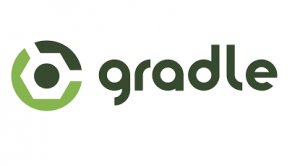
The article aims to briefly describe key concepts of Gradle along with providing code samples for hello world. The intended audience are those inquisitive souls who want to quickly get up and running with Gradle tool. For detailed information, you may want to check out the book, Building & Testing with Gradle.
Following is discussed in this article:
- What is Gradle?
- Coding Build Files with Groovy DSL
- Task Definition
- Closures
- HelloWorld Code Samples
What is Gradle?
As defined on http://www.gradle.org/, “Gradle is build automation evolved. Gradle can automate the building, testing, publishing, deployment and more of software packages or other types of projects such as generated static websites, generated documentation or indeed anything else.
Gradle combines the power and flexibility of Ant with the dependency management and conventions of Maven into a more effective way to build.”
Download Gradle and install & configure it based on instructions given on this page.
Gradle executes a build through three lifecycle phases: initialization, configuration, and execution. Initialization is the phase in which Gradle decides which projects are to participate in the build. Configuration is the phase in which those task objects are assembled into an internal object model.Execution is the phase in which build tasks are executed in the order required by their dependency relationships.
Coding Build Files with Groovy DSL
- Gradle build files syntax in Groovy language. It was chosen this way and NOT XML owing to drawbacks that XML has in relation with readability and hierarchical structure that does not fit very well in terms of representing program flow and data access. In addition, one could program one or more tasks, thanks to Groovy language.
- Given Groovy, one would get tempted to write complex conditional-based build tasks. However, after a while, this may become cumbersome to maintain such code. Thus, Gradle provides domain-specific language (DSL) tailored to the task of building code.
What are Tasks?
Gradle tasks are objects to which actions can be appended over the course of the build file. Gradle tasks are represented using keyword “task” followed by name followed “<<” and { actions }. As like any object, all Gradle tasks are objects that have properties and methods and extends the base class called as DefaultTask. As part of inheritence, every task have access to following methods and properties:
- Methods
- doFirst { <action code> }
- doLast { <action code> }
- onlyIf { <action code> }
- Properties
- description
- didWork
- enabled
- path
- logger
- logging
- temporaryDir
Following is the sample code of a helloworld task. Detailed code is provided later in the article.
task hello << {
println 'hello world'
}
Closures
Code between curly braces shown in above examples can be termed as Closures. This code block could be seen as objects which could be passed to methods like an argument or assigned to variable. In above example, code between curly braces such as println ‘hello world’ could be termed as closure.
HelloWorld Gradle Tasks
Following is the code samples that represents some of the following key aspects:
- task configuration
- task definition
- Methods such as doFirst, doLast, onlyIf
Code Samples
task hello
task pre_hello
// pre_hello task
pre_hello << {
println 'executing pre-hello...'
}
// Configuration for hello task; Notice that there is no << sign
hello {
dependsOn pre_hello // Note that dependsOn needs to be called from configuration block only.
println 'configuration block...'
}
// hello task
hello << {
println 'hello ' + System.properties['name']
}
// The doFirst method is called before the execution of task hello
hello.doFirst {
println '--- HelloWorld Program ---'
println ''
}
// The onlyIf method is used as conditional making sure that hello task gets executed only if condition satisfies
hello.onlyIf {
System.properties['name'] != ''
}
// The doLast method is called after the execution of task hello
hello.doLast {
println ''
println '--- Hope you liked it! ---'
}
Execution Steps
Install & configure Gradle as per the instructions manual. Once installed, create a gradle file named as build.gradle and paste the code shown above. Execute the command “gradle <taskname>”. In above case, it would be gradle -Dname=vitalflux hello
[adsenseyu1]
- Mathematics Topics for Machine Learning Beginners - July 6, 2025
- Questions to Ask When Thinking Like a Product Leader - July 3, 2025
- Three Approaches to Creating AI Agents: Code Examples - June 27, 2025
Nice introduction to Gradle. The syntax is pretty simple and intuitive, reminds me of authoring the markdown (.md) files.
thanks Ravi..!