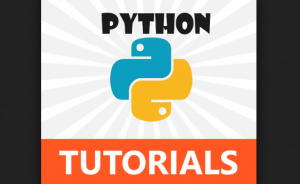
Learning Python has taken centerstage for many developers as Python is one of the key language for working in the field of data science/machine learning. If you are an experienced developer, this post would help you quickly get started with Python programming.
In this post, you will quickly learn some of the following in relation to Python programming:
- Data types
- Input/output operations
- Defining functions
- Conditional expressions
- Looping constructs
- String functions
- Defining Module
- Defining Classes
- Exception handling
Python Programming Concepts
- Data types: Python interprets and declares variables when they are equated to a value. The following represents how variables are casted to specific data types.
- float(variable): Casts variable to float
- int(variable): Casts variable to integer
- str(variable): : Casts variable to string
- Container data types:
- List is assigned using “[” and “]”. For example, the following is a list:
subjects = ['Maths', 'English', 'Hindi']
- List is assigned using “[” and “]”. For example, the following is a list:
- Input-output (I/O) Operations: The following different aspects related with performing input and output from Python program:
- Reading inputs from command prompt: _input(string_enclosed_in_singlequotes) is used to get the inputs from the user
- Printing the statements: _print(string_enclosed_in_singlequotes) is used to print a statement
- Reading from file: The following commands can be used for opening a file and reading from same:
fileVar = open("/home/support/Documents/hello.txt", "r"); print(fileVar.read())
When requiring to read lines, following methods can be used:
fileVar.readline() // Read one line fileVar.readlines() // Read all lines for line in fileVar: // Read line-by-line print(line)
- Writing to the file: The following commands can be used for opening a file and writing to the same:
fileVar = open("/home/support/Documents/hello.txt", "w") // Write mode; File is rewritten fileVar = open("/home/support/Documents/hello.txt", "a") // Append mode fileVar = open("/home/support/Documents/hello.txt", "r+") // Read-append mode fileVar.write("Hey, Whassup\n")
- Defining functions: Function is defined using the following code format. Note that functions are defined using keyword such as def.
def functionName(parameters): function code goes here
The following is a sample code representing a function, calculateInterest, and the code invoking the function:
def calculateInterest(amount, roi, yrs): total = (amount * pow(1 + (roi/100), yrs)) interest = total - amount return interest print('Interest Calculator:') # # Take the user inputs # amount = float(input('Principal amount ?')) roi = float(input('Rate of Interest ?')) yrs = int(input('Duration (no. of years) ?')) # # Invoke the function to calculate the interest # interest = calculateInterest(amount, roi, yrs) # # Print the interest # print('\nInterest calculated = %0.2f' %interest)
- Conditional (if-else) Constructs: The following is format for conditional expressions:
if condition_expression: code_goes_here else: code_goes_here
The following represents a sample code:
if(interest > 100): print('Interest is greater than 100') else: print('Interest is less than 100')
- Looping Constructs: The following represents looping constructs such as for and while loops.
- For loop: The following code represents for loop:
for variable in range(lowerlimit, upperlimit): code_goes_here
The following is a sample code:
count = 0 lowerlimit = 0 upperlimit = 3 for count in range(lowerlimit, upperlimit): print 'hello world'
- While loop: The following is the code format for while loop:
while conditional_expression: code_goes_here
The following is the sample code:
count = 0 upperlimit = 2 while count < upperlimit: print 'hello world' count += 1;
- For loop: The following code represents for loop:
- String Functions: Here are some out-of-the-box string functions which could be useful at times:
strVar = 'Hello world, how are you doing?' strVar.lower() // Change the string into lowercase strVar.upper() // Change the string into uppercase strVar.find('how') // Prints 13; Sstring index starts with 0 strVar.replace('world', 'vitalflux') // Replace the occurence of world with vitalflux strVar.split(' ') // Split the string with delimiter
- Defining Modules: A module can defined as a set of cohesive functions serving similar functionality. One can create module using following technique:
- Module name is defined as filename with “.py” extension
- Module consists of one or more functions
- Module can be imported using some of the following:
- import moduleName
- from moduleName import *
- The following is a sample module saved in a file named as maths.py comprising of function for adding, subtracting, multiplying and dividing
def add(number1, number2): return number1 + number2 def subtract(number1, number2): return number1 - number2 def multiply(number1, number2): return number1*number2 def divide(number1, number2): return number1/number2
The module can be imported in another file such as hello.py.
import maths print(maths.add(10, 20)) print(maths.subtract(10, 20)) print(maths.multiply(10, 20)) print(maths.divide(10, 20))
- Defining Classes: The class can be defined using the following technique:
- Save the class in a file name with “.py” extension. This becomes a module. A module can comprise of both classes and functions.
- The following is a module comprising of a class and a function
def printHello(name): print('Hello ' + name) class MachineLearner: def __init__(self, name): self.name = name def toString(self): return self.name def sayHello(self): print('hello ' + self.name)
- The following represents usage of class and function from above defined module:
from ml import MachineLearner, printHello # Instantiate the class mlearner = MachineLearner('HelloWorld') print(mlearner.toString()) mlearner.sayHello() # Use printHello method printHello('Vitalflux')
- Exception Handling: As like other programming language, Python also supports exception handling using try: and except:. The following is a sample code:
try: f = open('/home/support/Documents/hello.txt') s = f.readline() except IOError as e: print "I/O error({0}): {1}".format(e.errno, e.strerror)
Further Reading / References
- Python Tutorials
- Python Website
- PythonAnywhere to code and run python in the cloud using online console
Summary
In this post, you learned about how to get started Python programming if you are an experienced developer.
Did you find this article useful? Do you have any questions or suggestions about this article in relation to getting started with Python programming? Leave a comment and ask your questions and I shall do my best to address your queries.
- Completion Model vs Chat Model: Python Examples - June 30, 2024
- LLM Hosting Strategy, Options & Cost: Examples - June 30, 2024
- Application Architecture for LLM Applications: Examples - June 25, 2024
Leave a Reply