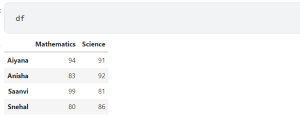
Pandas is a powerful data analysis tool in Python that can be used for tasks such as data cleaning, exploratory data analysis, feature engineering, and predictive modeling. In this article, we will focus on how to use Pandas’ loc and iloc functions on Dataframe, as well as brackets with Dataframe, with examples. As a data scientist or data analyst, it is very important to understand how these functions work and when to use them.
In this post, we will work with the following Pandas data frame.
import pandas as pd
marks = {
"Mathematics": [94, 83, 99, 80],
"Science": [91, 92, 81, 86]
}
students = ["Aiyana", "Anisha", "Saanvi", "Snehal"]
#
# Create Dataframe
#
df = pd.DataFrame(marks, index=students)
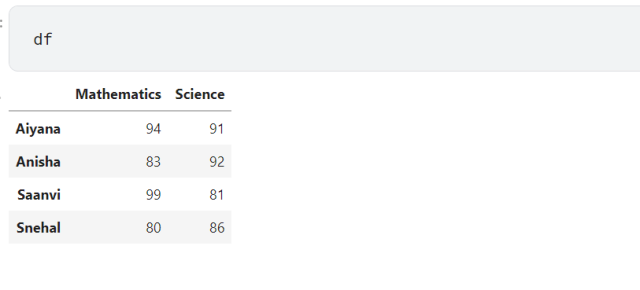
Use loc and iloc functions to get Rows of Dataframe
The loc function is used to get a particular row in a Dataframe by label location. The loc function is used for label-based indexing. The code below represents the following scenarios:
- Get a particular row by label
- Get multiple rows by label
- Get specific columns of selected rows by labels
# Get marks of specific student (Get row by label)
#
df.loc["Anisha"]
# Get marks of Anisha and Snehal (Get multiple rows by labels)
#
df.loc[["Anisha", "Snehal"]]
# Get Mathematics marks of Anisha and Snehal (Get specific columns of
# specific rows by labels)
#
df.loc[["Anisha", "Snehal"], ["Mathematics"]]
The iloc function is used to get any particular row by integer location index and hence iloc name. The iloc function can be used to get one or more elements at specified indices. The iloc is used for positional indexing. The code below represents the following scenarios:
- Get particular rows by index
- Get multiple rows by indices
- Get specific columns for multiple rows by indices
# Get particular rows by index
#
df.iloc[0]
# Get multiple rows by indices
#
df.iloc[0:2]
# Get specific columns for multiple rows by indices
#
df.iloc[0:2, [1]]
Use Brackets to get Columns of Dataframe
The brackets operator can be used to extract a single column or multiple columns from a Dataframe. The code below represents the following scenarios:
- Extract a single column
- Extract multiple columns; Note how the list of column names is passed to the data frame to extract multiple columns
# Extract single column
#
df["Mathematics"]
# Extract multiple columns
#
df[["Mathematics", "Science"]]
Conclusion
In this article, we have covered how to use the loc and iloc functions and brackets in Pandas Dataframe. We also looked at how to use brackets to extract columns from a data frame. These are important functions that every data analyst should be familiar with. With practice, you will be able to use them quickly and easily on your own dataframes. Thanks for reading!
- Completion Model vs Chat Model: Python Examples - June 30, 2024
- LLM Hosting Strategy, Options & Cost: Examples - June 30, 2024
- Application Architecture for LLM Applications: Examples - June 25, 2024
Leave a Reply