Unit Testing with JUnit & Mockito – Code Examples
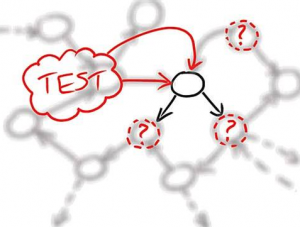
The article presents quick code example for doing unit testing with JUnit & Mockito mocking framework. It also represents unit testing naming convention where features text is used for naming the test methods thereby representing test class as alternate documentation for the class under test. Following is done while defining Mock member variables Use @Mock annotation ahead of member variable definitions Following are steps done in the setup method: Initialize the mock using MockitoAnnotations.initMocks(this); Object of class under test is initialized with the mock objects Following steps are used in each of the unit test methods: Create/Initialize required objects for working with mocks and methods under test Set the mock …
7 Popular Unit Test Naming Conventions
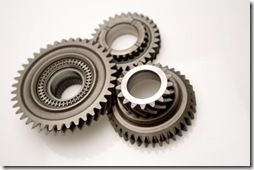
The article presents a compiled list of unit tests naming strategy that one could follow for naming their unit tests. The article is intended to be a quick reference instead of going through multiple great pages such as following. That said, to know greater details, please feel free access one of these pages listed below and know for yourself. Naming Standards for Unit Tests Naming Unit Tests Responsibly What are some popular naming conventions for unit tests? Unit Tests Naming Best Practices GivenWhenThen Technique Following are 7 popular unit tests naming conventions that are found to be used by majority of developers and compiled from above pages: MethodName_StateUnderTest_ExpectedBehavior: There are …
What is Difference Between Select and Datalist?
The article represents the key difference between Select and Datalist and emphasize on related best practices. Following are key differences Select is an form input type while Datalist isn’t Select input element presents options for the users from which they need to select one of them. On the otherhand, Datalist presents a list of suggested values to the associated input form (text) field and users are free to select one of those suggested values or type in their own value. With Select, users may have to scan a long list for selecting one of the values, while with Datalist, the values are provided as hints and users ain’t bound to …
AngularJS & Bootstrap Hello World UI Template
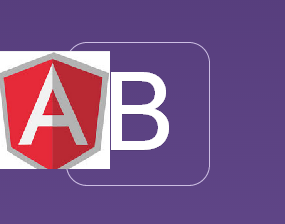
The article is a placeholder for the copy & paste code for AngularJS and Bootstrap. If one wants to quickly get started with AngularJS and Bootstrap, one may want to bookmark this page. Points to pay attention: AngularJS script referenced from Google hosted libraries Bootstrap scripts and CSS files from bootstrapcdn <!DOCTYPE html> <html ng-app=”helloApp”> <head> <title>HelloWorld</title> <link rel=”stylesheet” href=”//netdna.bootstrapcdn.com/bootstrap/3.1.1/css/bootstrap.min.css”> <script src=”//ajax.googleapis.com/ajax/libs/angularjs/1.2.17/angular.min.js”></script> <script src=”//netdna.bootstrapcdn.com/bootstrap/3.1.1/js/bootstrap.min.js”></script> <script> var helloApp = angular.module( “helloApp”, [] ); helloApp.controller( “HelloCtrl”, [ ‘$scope’, function($scope) { $scope.name = “ajitesh shukla”; }]); </script> </head> <body ng-controller=”HelloCtrl”> <div class=”page-header”> <h1>Hello World Sample Program</h1> </div> <div> <form class=”form-horizontal” role=”form”> <div class=”form-group”> <label class=”col-md-2 control-label”>Type Your Name</label> <div class=”col-md-4″> <input type=”text” …
7 Reasons Why One Should Try Chrome Dev Editor (CDE)
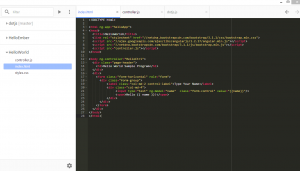
The article presents information on Chrome Dev Editor which was made available to developers in Google IO 2014 event. I played with it and checked various different capabilities. If you think I missed on one or more cool features, please feel free to shout. Also, created a sample AngularJS Hello World program. Following picture represents the following: Ability to clone GIT ptoject Ability to create javascript web apps. Shown is AngularJS helloworld app. Well, frankly speaking, to work with Javascript Web Apps, it is same like any other text editor. However, it brings following benefits to the table which makes it very attractive and WORTH A SPIN: IDE Features: With …
AngularJS – How to Post JSON Data using AJAX & SpringMVC
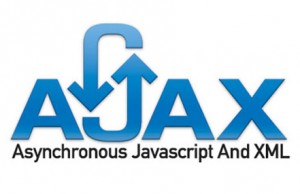
The article presents code samples that one could use to quickly get started with posting JSON data using AngularJS $http service while working with SpringMVC web application. The demo for the code below could be found on this page, http://hello-angularjs.appspot.com/angularjs-http-service-ajax-post-json-data-code-example. Earlier, I posted this article where one could post the html data (text/html) using AJAX & SpringMVC. Following are key steps: Create SpringMVC Controller methods and a POJO Create AngularJS Controller Method Create View SpringMVC Controller methods and a POJO Following are key steps: Create a controller method to access the page consisting of UI that will be posting using AJAX Create a controller method that shall be receiving the …
AngularJS & SpringMVC – How to Fix 415 Unsupported Media Type Error
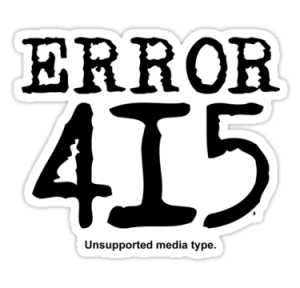
The article presents tips on how to fix 415 Unsupported Media Type error while working with AngularJS & SpringMVC web application when one tries to POST JSON data using $http service. The sole reason I wanted to write this blog is to help many of my friends who are most likely to hit this one when they work on posting JSON data to SpringMVC web controller using $http service. Possible scenarios: Most likely, you might not have included Jackson libraries in the classpath. Thus, go to http://wiki.fasterxml.com/JacksonDownload and download all the jar files. Alternatively, get slightly back-dated version of all of the libraries in one jar file from http://www.java2s.com/Code/Jar/j/Downloadjacksonall199jar.htm. …
AngularJS – How to POST Data using AJAX & Spring MVC – Part 1
The article presents the code example that one may use to post data to server using AngularJS $http service, while working with Spring MVC web application. The same is demonstrated in the following page: http://hello-angularjs.appspot.com/angularjs-http-service-ajax-post-code-example. This article is first in the series of articles on different techniques that could be used to POST different formats of data to the server when working with AngularJS and Spring MVC. In this article, the code samples demonstrate how to post plain HTML text data (text/html) format to the server. Following are key steps: Create Spring MVC web controllers methods Create AngularJS controller method using $http service to post data Create view to receive input …
AngularJS – How to Get Data from AJAX & Spring MVC
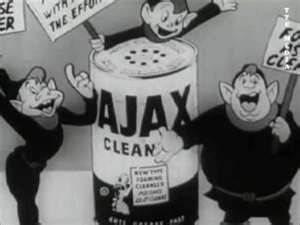
The article presents steps one need to code in order to get data from server using XMLHttpRequest (XHR) while working with Spring MVC Java web application. Watch the demo and code samples at http://hello-angularjs.appspot.com/angularjs-http-service-ajax-get-code-example. Following are key steps: Write two methods in Spring MVC controller, one to load the page and other to serve AJAX request Write AngularJS code to get the data (model) in the controller method using $http Write the view to display the data Spring MVC Controller Methods Following are two methods one need to write in the Spring MVC Controller: Method below serves the page, views/httpservice_get.jsp when accessed using the following URL: http://hello-angularjs.appspot.com/angularjs-http-service-ajax-get-code-example. Try …
OWASP Broken Authentication and Session Management Example
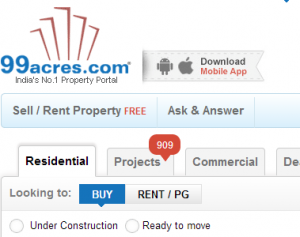
The article presents an example on one of the top OWASP vulnerability related with authentication and session management. This is termed as “Broken Authentication and Session Management”. To know more about this vulnerability and related details, visit OWASP page for broken authentication and session management. I was surfing a website, http://www.99acres.com, few days back and tried to retrieve my password using “Forgot Password” page. As I entered my username, I was amazed to see my email address shown there. I, then, tried another name such as “karthik” and following was the message:“An email has been sent to karthiksundaram@gmail.com. Please click on the link provided in the email to create …
AngularJS Coding Best Practices
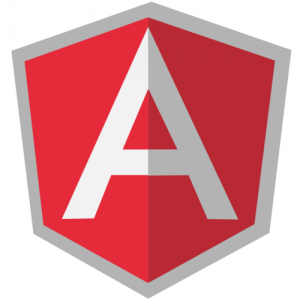
The article lists down some of the best practices that would be useful for developers while they are coding with AngularJS. These are out of my own experiences while working on AngularJS and do not warranty the entire list. I am sure there can be more to this list and thus, request my readers to suggest/comment such that they could be added to the list below. Found some of the following pages which presents a set of good practices you would want to refer. Thanks to the readers for the valuable contribution. AngularJS Style Guide App Structure Best practices Initialization One should try and place the <script> tag including …
How to Become a Technical Architect or an IT Architect?
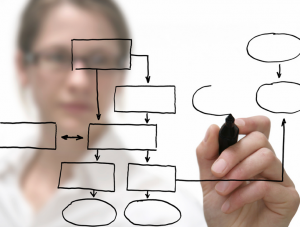
The article presents a perspective on what is required to be done to become a Software Architect given you are currently playing the role of a software developer or a senior software developer. I have put up this blog as an answer to a related question put up by one of the vitalflux.com esteemed reader. Well, there is no right or wrong answer to this question. There can only be different perspectives. In case you have different views, please feel free to comment. Before I go on to present my perspective, check out some of the following links to understand what is Software Architecture? http://en.wikipedia.org/wiki/Software_architecture http://www.sei.cmu.edu/architecture/ http://www.ibm.com/developerworks/rational/library/feb06/eeles/ Different Designations …
AngularJS Interview Questions – Questions Set 1
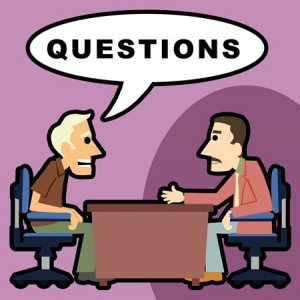
The article lists down some of the interview questions that could be asked in relation with AngularJS. Most of the answers can be found on the home site, http://www.angularjs.org. Following are another set of questions that have already been published earlier. Also, feel free to take tests on this page. Interview questions – Set 2 Interview questions – Set 3 Interview questions – Set 4 Questions Set What is notion of directives in AngularJS? Ans: https://docs.angularjs.org/guide/directive Name some of the most commonly used directives? What is uage of ng-app, ng-controller, ng-view, ng-model etc? Ans: https://docs.angularjs.org/api Explain how MVC is achieved with AngularJS? What are the benefits of client-side MVC, in general? Ans: https://docs.angularjs.org/guide/controller …
AngularJS – 7 Steps for Unit Testing AngularJS Scripts with Jasmine
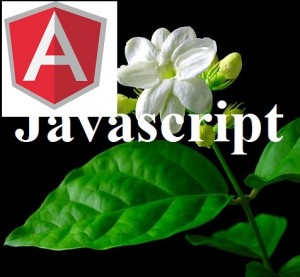
This article presents instructions on what would it take to create a setup and run unit testing for your AngularJS scripts using Jasmine framework. I shall be presenting various samples on my AngularJS website, http://hello-angularjs.appspot.com. Sorry for the typos in this article and please feel free to suggest/add any points if I missed them out. Following are key steps: Learn Jasmine Create Unit Tests using Jasmine Download and install NodeJS Download & Install Karma Configure Karma Place Scripts Appropriately Execute Tests Learn Jasmine Jasmine, one of the very popular Javascript unit testing framework, can be used for unit testing one’s AngularJS scripts. As a matter of fact, Angular …
Javascript Unit Testing using Jasmine – Code Examples
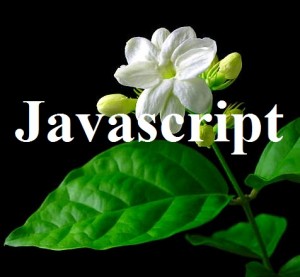
The article lists down some of the unit tests samples for testing Javascript code. The unit tests in this article tests the javascript code presented in this article, “What are Objects in Javascript?”. Before presenting code samples, lets try and understand what is Jasmine? As on the Jasmine website, it is defined as a behavior-driven development framework for testing JavaScript code. It does not depend on any other JavaScript frameworks. It does not require a DOM. And it has a clean, obvious syntax so that you can easily write tests. By what I learnt so far, I could vouch for the statement “And it has a clean, obvious syntax so that …
What are Objects in Javascript ? – Code Samples
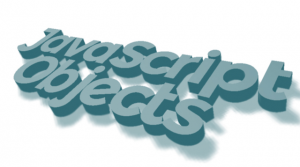
Wrote this article on Objects & Javascript for future reference. Codecademy.org is my favorite go-to-place for learning Javascript. Javascript Objects could be defined in following three manners. Objects created as Variables Objects created using new Object() Objects created using Constructor Objects created as Variables // Defining object as a variable var School = { name: “DAV Public School”, studentsCount: 600, admissionOpen: false, }; School.isAdmissionOpen = function() { return this.admissionOpen; }; School.setAdmissionStatus = function( openOrClosed ) { this.admissionOpen = openOrClosed; }; Objects created using new Object() In the example below, College is an object type. // Creating object using new Object() var College = new Object(); College.name = “IIT …
I found it very helpful. However the differences are not too understandable for me