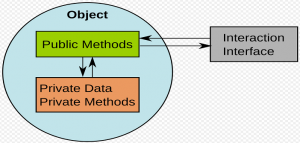
- Encapsulation – Basic Concepts
- Encapsulation – Code Examples
Encapsulation – Basic Concepts
As per Wikipedia page, Encapsulation is the packing of data and functions into a single component….It allows selective hiding of properties and methods in an object… In OOPs based language, access specifier such as “public”, “private” and “protected” are used to specify the access properties of member variables and methods. Following rule applies for a Class member variable and methods:
- Without “this” keyword, the member variable and methods become private. In example below, name is public, and age is private.
function Person() { this.name = "Calvin"; age = 25; }
- In order to make the member variable and methods public, one needs to use “this” keyword.
In order to access the value of private variable, it needs to be accessed using a public method. Take a look at example below where getAge is used to access the value of “age” which is a private variable.
function Person() {
this.name = "Calvin";
age = 25;
this.getAge = function() {
return age;
}
}
Following rule applies for an object:
- Be default, the member variable and method of objects are public. Take a look at the code sample below which demonstrates how methods and attributes could be access:
var calvin = { name: "Calvin Hobbes", age: 15, getAge: function() { return this.age; } }
Pay attention to the fact that “this” or “var” keyword is not needed (not supported as well; throws error) to be used with member variable and methods. However, “this” keyword is used to access the member variable within a method. If not used, it will look for local variable, “age” defined using getAge method.
Encapsulation – Code Examples
// Following represents class, Company, that takes two arguments such as name and age.
// Pay attention to "this" keyword which makes the variable and method, public
function Company(name, age) {
// Name & Age variables are public; Meaning, they can be accessed by the instance of the class
this.name = name;
this.age = age;
// type is a private variable; It can not be accessed publicly
var type = 1;
this.getName = function() {
return this.name;
}
this.getAge = function() {
return this.age;
}
this.getType = function() {
return type;
}
}
var infosys = new Company( "Infosys Technologies");
// Below would print Infosys Technologies
console.log( infosys.name );
// Below would print "undefined". Note the "var" keyword. Even if you do not use "var" and define the variable as it is, it is private in nature.
// In order to access the private variable value, it needs to be accessed using a public method.
console.log( infosys.getType() );
- Completion Model vs Chat Model: Python Examples - June 30, 2024
- LLM Hosting Strategy, Options & Cost: Examples - June 30, 2024
- Application Architecture for LLM Applications: Examples - June 25, 2024
Leave a Reply