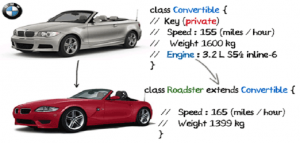
This article represents OOPs inheritance concepts in Javascript along with code examples. Please feel free to comment/suggest if I missed to mention one or more important points. Also, sorry for the typos.
Following are the key points described later in this article:
- What is Inheritance?
- Inheritance – Code Example
What is Inheritance?
As per Wikipedia page on Inheritance, In object-oriented programming (OOP), inheritance is when an object or class is based on another object or class, using the same implementation (inheriting from a class) or specifying implementation to maintain the same behavior (realizing an interface; inheriting behavior).
As per Webopedia page on inheritance, In object-oriented programing (OOP) inheritance is a feature that represents the “is a” relationship between different classes. Inheritance allows a class to have the same behavior as another class and extend or tailor that behavior to provide special action for specific needs.
Pay attention to the fact that “is-a” relationship exists between base and the derived class. In example below, ITCompany (derived class) “is-a” Company (base class). The class which is inherited is called as “base” class and the class that inherits is called as derived class. Pay attention to some of the following in relation with inheritance:
- “prototype” keyword is used for inheritance. Thus, in code example below, the fact that ITCompany inherits from Company is represented using following:
ITCompany.prototype = new Company();
- Once inherited, the derived class can access properties and methods of the base class. In the code example below, instance of ITCompany, hcl, invoked setEmployee and getEmployee although it was not defined as part of ITCompany class.
- Once inherited, the derived class can extend the methods and properties of base class and provide its own implementation. The method, getType(), invoked on hcl instance returns 2 rather than 0.
Inheritance – Code Example
// Class Company modeling any company
// This represents what is called as base class; Later, we shall make derived class which inherits
// the properties and methods of this class
function Company(name) {
this.name = name;
this.type = 0;
this.employee = 0;
this.getName = function() {
return this.name;
}
this.getType = function() {
return this.type;
}
this.setEmployee = function(count) {
this.employee = count;
}
this.getEmployee = function() {
return this.employee;
}
}
function ITCompany(name) {
this.name = name;
this.getType = function() {
return 2;
}
}
// Lets make an instance of class Company
//
var rel = new Company( "Reliance Pvt Ltd" );
console.log( rel.getName() ); // Prints Reliance Pvt Ltd
console.log( rel.getType() ); // Prints 0
console.log( rel.getEmployee() ); // Prints 0
rel.setEmployee( 150000 );
console.log( rel.getEmployee() ); // Prints 150000
// Lets create an instance of ITCompany
//
var hcl = new ITCompany( "HCL Technologies" );
console.log( hcl.getName() ); // Prints "undefined is not a function"
// Lets inherit the ITCompany from Company; This is also natural as
// an ITCompany "is a" Company.
// Pay attention that "prototype" is used with ITCompany to inherit the properties and
// methods of base class
ITCompany.prototype = new Company();
var hcl = new ITCompany( "HCL Technologies" );
console.log( hcl.getName() ); // Prints "HCL Technologies"
hcl.setEmployee( 125000 );
console.log( hcl.getEmployee() ); // Prints 125000
console.log( hcl.type );
console.log( hcl.getType() );
Latest posts by Ajitesh Kumar (see all)
- Completion Model vs Chat Model: Python Examples - June 30, 2024
- LLM Hosting Strategy, Options & Cost: Examples - June 30, 2024
- Application Architecture for LLM Applications: Examples - June 25, 2024
Leave a Reply