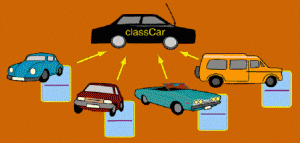
- What are Classes?
- What are Objects?
- Code Examples – Classes & Objects
What are Classes?
Class is a construct used in several object-oriented programming languages such as Java/C++ etc, primarily, to model real-world objects. For instance, company, vehicle, shape, car, circle etc. Unlike programming languages such as Java where “class” keyword is used to represent class, in Javascript, a class is represented or created using “function” such as following. In example below, name of the class is Company.
function Company() {
}
A class could have one or more properties/attributes and one or more methods that are used to represent behaviour. Lets take a look at example below. In example below, “name” is the attribute of the class, and getName is the method.
function Company(name) {
this.name = name;
this.getName = function() {
return this.name;
}
}
“this” keyword makes the member variables and methods publicly accessible. Remove the “this” keyword and you would get “undefined” message if you try to access the variable on the object of the class. If you do not define “this” with a member variable or a method, it becomes “private“. To make an instance of the class, Company, one needs to use “new” keyword. Take a look at code example below.
What are Objects?
Objects are instances of the class. Following demonstrates objects created of type “Company”.
var microsoft = new Company("Microsoft");
console.log( microsoft.getName() ); // This prints the name, "Microsoft"
var infosys = new Company( "Infosys" );
console.log( infosys.getName() ); // This prints the name, "Infosys"
In above example, infosys and microsoft are instances of class, Company. In other words, infosys and microsoft is of data type, Company. In Javascript, you could create simple objects in following manner:
var microsoft = {
name: "Microsoft",
hq: "Seattle",
getName: function() {
return this.name;
}
}
When creating objects of above types, member variables and methods do not need the usage of “this” keyword as these variables/methods are specific to the object. Thus, member variables and methods are, by default, public. You could access the variables (such as name) of these objects using following:
console.log( microsoft.name );
console.log( microsoft[ "name" ] );
Code Examples – Classes & Objects
Following represents some code examples on classes and objects.
// Following represents class, Company, that takes two arguments such as name and age.
// Pay attention to "this" keyword which makes the variable and method, public
function Company(name, age) {
this.name = name;
this.age = age;
this.getName = function() {
return this.name;
}
this.getAge = function() {
return this.age;
}
}
// See how "new" operator is used to create an instance of the class, Company
//
var hcl = new Company("HCL Technologies", 30 );
console.log( hcl.getName() );
console.log( hcl.name );
console.log( hcl["age"] ); // Represents the fact that member variables can be accessed using [ "property_name" ]
// Simple objects in Javascript
//
var microsoft = {
name: "Microsoft",
hq: "Seattle",
getName: function() {
return this.name;
}
}
console.log( microsoft.name );
console.log( microsoft.getName() );
- Mathematics Topics for Machine Learning Beginners - July 6, 2025
- Questions to Ask When Thinking Like a Product Leader - July 3, 2025
- Three Approaches to Creating AI Agents: Code Examples - June 27, 2025
I found it very helpful. However the differences are not too understandable for me