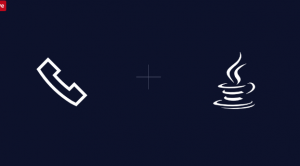
This post represents sample Java code (example) to demonstrate how to invoke Twilio Rest API to play pre-recorded audio in a phone call made to the users. The code could be used to in some of the following usecase:
- Alert users via phone call in relation to different kind of critical events such as security issues, production downtime, production issues etc.
- Play different kind of messages to users in relation to different occasions such as birthdays, anniversaries etc.
The code can be used to play audio stored at locations such as cloud platform storage (AWS storage, Google/Azure storage etc.) The key is to provide public access (READ permission) to these buckets in which audio files get stored in order to be played in the phone call.
Pay attention to some of the following in the code given below:
- Retrieve account SID and auth token details from Twilio web console
- Retrieve/buy phone number in Twilio; Use the phone number as fromPhoneNumber (in the code given below)
- Use TwilioRestClient APIs to make the phone calls
package com.vflux.core; import com.twilio.http.HttpMethod; import com.twilio.http.Request; import com.twilio.http.Response; import com.twilio.http.TwilioRestClient; import com.twilio.http.TwilioRestClient.Builder; public class CustomTwilioRestClient { public static void main(String[] args) { // // Retrieve Account SID, Auth Token and fromPhoneNumber // from Twilio Web Console // String accountSID = "VC5a1234f11ecca26b32123c8e8513e44"; String authToken = "abc7e512df653421c7a71b9c9810fc66"; String fromPhoneNumber = "+13111912340"; // // This is the number to which phone call will be made // String toPhoneNumber = "+919888000012"; // // This is the audio file which will be played // once users picks up the call // String audioPath = "https://remainders-12123.s3.amazonaws.com/hello.mp3"; // // Twilio Rest Client // TwilioRestClient twilioRestClient = (new Builder(accountSID, authToken)).build(); // // URL of the audio message which will be played to the user // String url = "http://twimlets.com/message?Message%5B0%5D=" + audioPath; // // Instantiate Twilio Request; Add request parameters such as fromPhoneNumber, toPhoneNumber and // URL // Request request = new Request(HttpMethod.POST, "https://api.twilio.com/2010-04-01/Accounts/"+ accountSID + "/Calls"); request.addPostParam("From", fromPhoneNumber); request.addPostParam("To", toPhoneNumber); request.addPostParam("Url", url); // // Invoke the Call // Response response = twilioRestClient.request(request); System.out.println(response.getContent()); } }
Further Reading / References
Summary
In this post, you learned about invoking Twilio REST API from standalone Java program to play audio to a destined phone number.
Did you find this article useful? Do you have any questions or suggestions about this article in relation to using Twilio REST APIs from standalone Java program? Leave a comment and ask your questions and I shall do my best to address your queries.
- Mathematics Topics for Machine Learning Beginners - July 6, 2025
- Questions to Ask When Thinking Like a Product Leader - July 3, 2025
- Three Approaches to Creating AI Agents: Code Examples - June 27, 2025
I found it very helpful. However the differences are not too understandable for me