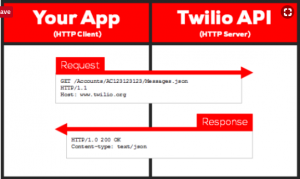
Twilio is a very popular communication service which could be used to send SMS, make the phone or video calls to people in different countries. In this post, you will learn about creating Java and Spring Boot app in relation to how to use Twilio phone service to make a phone call and play audio stored at AWS S3 bucket. The following are some of the related use cases:
- Play security and other alerts via phone calls
- Play messages of any kind via phone calls
The following are some of the topics which would be covered in this post:
- Define VoiceService interface defining APIs
- Create Twilio Voice Service implementation
- Load Configuration Properties from application.properties file
- Application.properties file defining configuration properties
- Create an AWS S3 Bucket
- Invoke TwilioVoiceService from SpringBoot App
VoiceService interface defining APIs
The interface represents API, playVoice, which plays an audio found at the path, audioPath, in the phone call made to the number, toNumber.
public interface VoiceService { void playVoice(String toNumber, String voicePath); }
Twilio Voice Service Implementation
Pay attention to some of the following:
- An instance of TwilioRestClient is used for invoking request API. The following code represents the same:
Response response = this.twilioRestClient.request(request);
- A Call object needs to be created with following parameters in order for playing the audio in the phone call. The following are key parameters:
- From phone number
- To phone number
- URL: Audio file URL which needs to be played in the phone call
- TwilioRestClient instance
import java.net.URI; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Component; import com.twilio.http.TwilioRestClient; import com.twilio.rest.api.v2010.account.Call; import com.twilio.type.PhoneNumber; @Component public class TwilioVoiceService implements VoiceService { private static final String APIVERSION = "2010-04-01"; private static final String TWIMLET_BASE_URL = "http://twimlets.com/message?Message%5B0%5D="; private TwilioRestClient twilioRestClient; private String fromPhoneNumber; public TwilioVoiceService(@Autowired TwilioRestClient twilioRestClient, @Autowired String fromPhoneNumber) { this.twilioRestClient = twilioRestClient; this.fromPhoneNumber = fromPhoneNumber; } @Override public void playVoice(String toNumber, String voicePath) { String url = TWIMLET_BASE_URL + voicePath; PhoneNumber to = new PhoneNumber(toNumber); // Replace with your phone number PhoneNumber from = new PhoneNumber(this.fromPhoneNumber); // Replace with a Twilio number URI uri = URI.create(url); // Make the call Call call = Call.creator(to, from, uri).create(this.twilioRestClient); System.out.println(call.getSid()); } }
Load Configuration Properties from application.properties file
Pay attention to some of the following:
- TwilioRestClient bean is created using following code. Information such as ACCOUNT_SID and AUTH_TOKEN needs to be obtained from Twilio console and put in the application.properties file against the key such as twilio.account.sid and twilio.auth.token respectively.
(new Builder(this.accountSID, this.authToken)).build()
- Information such as fromPhoneNumber is loaded from application.properties file using key such as twilio.from.phone.number. This number needs to be bought in Twilio account. It could be otained from Twilio, Manage Numbers page.
import org.springframework.beans.factory.annotation.Value; import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration; import org.springframework.context.annotation.PropertySource; import com.twilio.http.TwilioRestClient; import com.twilio.http.TwilioRestClient.Builder; @Configuration @PropertySource("classpath:application.properties") public class TwilioAppConfig { @Value("${twilio.account.sid}") String accountSID; @Value("${twilio.auth.token}") String authToken; @Value("${twilio.from.phone.number}") String fromPhoneNumber; @Bean(name = "twilioRestClient") public TwilioRestClient twilioRestClient() { return (new Builder(this.accountSID, this.authToken)).build(); } @Bean(name = "fromPhoneNumber") public String fromPhoneNumber() { return this.fromPhoneNumber; } }
Application.properties file defining configuration properties
Pay attention to some of the following:
- Key, twilio.account.sid, represents Account SID.
- Key, twilio.auth.token, represents Auth token
- Key, twilio.from.phone.number, represents phone number from which phone will be made.
All of the above information can be retrieved from Twilio Web UI Console.
# # Twilio properties # twilio.account.sid = HFG7607sjhgdjsd45363c8346gdsh twilio.auth.token = fddg7461df92846shsdc7564jhsg twilio.from.phone.number = +13202895440
Create an AWS S3 Bucket
Create an AWS S3 bucket, say, audiofiles. Provide read permission to files stored in this bucket. Record and upload an audio file, say, helloworld.mp3 in this bucket.
Invoke TwilioVoiceService from SpringBoot App
Pay attention to some of the following:
- TwilioVoiceService is autowired
- TwilioVoiceService.playVoice plays the audio found at the path, filePath (AWS S3 bucket path), to the number, toPhoneNumber.
import java.io.IOException; import java.io.InputStream; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.boot.CommandLineRunner; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; import com.vflux.rbot.storage.CloudStorage; import com.vflux.rbot.texttospeech.CustomPolly; import com.vflux.rbot.voice.VoiceService; @SpringBootApplication public class RecruiterbotApplication implements CommandLineRunner { @Autowired VoiceService twilioVoiceService; public static void main(String[] args) { SpringApplication app = new SpringApplication(RecruiterbotApplication.class); app.run(args); } @Override public void run(String... arg0) throws IOException { String toPhoneNumber = "+919875169056"; String filePath = "http://audiofiles.s3.amazonaws.com/helloworld.mp3"; this.twilioVoiceService.playVoice(toPhoneNumber, filePath); } }
Further Reading / References
Summary
In this post, you learned about getting started with integrating Twilio & AWS S3 using Java and Spring Boot.
Did you find this article useful? Do you have any questions or suggestions about this article in relation to using Twilio REST APIs to play audio file placed in AWS S3? Leave a comment and ask your questions and I shall do my best to address your queries.
- Mathematics Topics for Machine Learning Beginners - July 6, 2025
- Questions to Ask When Thinking Like a Product Leader - July 3, 2025
- Three Approaches to Creating AI Agents: Code Examples - June 27, 2025
I found it very helpful. However the differences are not too understandable for me