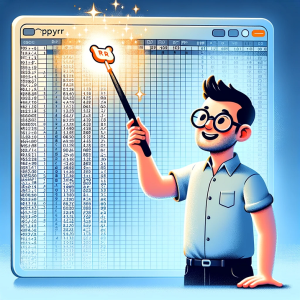
Data manipulation is a fundamental aspect of data analysis, and R, with its dplyr package, offers an efficient and readable way to perform such tasks. In my experience working with various datasets, I have often encountered situations where I needed to add rows to an existing DataFrame. The dplyr package, part of the tidyverse collection, makes these tasks intuitive and efficient. In this blog post, I’ll share two common scenarios: adding a single row and adding multiple rows to a DataFrame using dplyr. If you would want to learn about how to add rows to Pandas Dataframe using Python, check out my related post – Pandas Dataframe: How to Add Rows & Columns.
Adding a Single Row to an Existing R DataFrame Using dplyr
There are times when you need to append just one row to your dataset. Perhaps it’s a new entry or a correction. The add_row() function from dplyr is perfectly suited for this.
In a project, I had a DataFrame containing customer details, and I needed to add a new customer record. Here’s how I did it.
# Install dplyr if you haven't already # install.packages("dplyr") # Load the dplyr package library(dplyr) # Existing data frame customers <- data.frame( CustomerID = c(101, 102, 103), Name = c("Alice", "Bob", "Charlie"), Age = c(29, 35, 40) ) # Adding a new customer customers <- customers %>% add_row(CustomerID = 104, Name = "Diana", Age = 32) # Viewing the updated DataFrame print(customers)
In this example, a new row with CustomerID = 104, Name = “Diana”, and Age = 32 is seamlessly added to the existing customers DataFrame. The %>% operator, a hallmark of the tidyverse, makes the code readable and easy to understand.
Adding Multiple Rows to an Existing R DataFrame Using dplyr
Sometimes, you might have a batch of records to add. For instance, in a data analysis project, I received additional data after the initial processing. Using bind_rows(), I could easily integrate this new data into the existing DataFrame.
Here’s how I added multiple rows to a DataFrame of product information:
# Load the dplyr package library(dplyr) # Original product DataFrame products <- data.frame( ProductID = c(1, 2, 3), Name = c("Laptop", "Camera", "Smartphone"), Price = c(1200, 500, 800) ) # New products to add new_products <- data.frame( ProductID = c(4, 5), Name = c("Tablet", "Headphones"), Price = c(600, 150) ) # Adding the new products products <- bind_rows(products, new_products) # Viewing the updated DataFrame print(products)
In this case, new_products, containing two new product entries, is added to the products DataFrame. bind_rows() is ideal for this kind of operation, especially when dealing with larger datasets.
Most Common Scenarios for Adding Rows to Dataframe
Here are the top five most common scenarios for adding rows to a DataFrame in R using dplyr, based on frequency and general applicability in data analysis and manipulation:
- Merging Data from Different Sources: Combining datasets from multiple sources is a very common task. You might have data spread across different files or databases, and consolidating it into one DataFrame is often a necessary step in data analysis.
- Data Correction or Updating: As new information becomes available or errors are discovered in existing datasets, adding rows with corrected or updated data is a frequent necessity. This ensures that analyses are based on the most accurate and up-to-date information.
- Time Series Data: In handling time series data, such as financial, meteorological, or sales data, new data points are continually generated. Adding these new data points (rows) to an existing dataset is a routine task, especially for ongoing analyses.
- Simulation or Testing: In simulations or algorithm testing, generating and adding new data rows is common. This might involve adding simulated results to test hypotheses or to evaluate the performance of statistical models and machine learning algorithms.
- Incremental Data Loading: In many real-world scenarios, data is not available all at once but is instead collected or received incrementally (e.g., daily, weekly, or monthly updates). In these cases, new data is routinely added to existing datasets for cumulative analysis.
These scenarios are widely encountered across various fields, including business, science, and technology, making them highly relevant for a broad range of R users.
- Mathematics Topics for Machine Learning Beginners - July 6, 2025
- Questions to Ask When Thinking Like a Product Leader - July 3, 2025
- Three Approaches to Creating AI Agents: Code Examples - June 27, 2025
I found it very helpful. However the differences are not too understandable for me