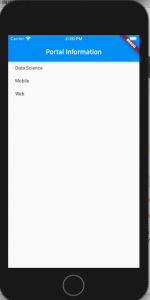
In this post, we will understand the Flutter concepts related to Map<String, Dynamic> with some code example / sample. For greater details, visit Flutter website.
Map<String, dynamic> maps a String key with the dynamic value. Since the key is always a String and the value can be of any type, it is kept as dynamic to be on the safer side. It is very useful in reading a JSON object as the JSON object represents a set of key-value pairs where key is of type String while value can be of any type including String, List<String>, List<Object> or List<Map>. Take a look at the following example:
{
"name": "Vitalflux.com",
"domain": ["Data Science", "Mobile", "Web"],
"noOfArticles": [
{"type": "data science", "count": 50},
{"type": "web", "count": 75}
]
}
Above is an example of JSON object comprising of three keys such as name with value of type String, “domain” with value of type List<String> and “noOfArticles” with value of type List<Object> (List<Map>)
Such JSON objects can easily be read with Map<String, dynamic> object and displayed on screen appropriately.
There are various ways in which one could work with Map<String, dynamic> object. They are some of the following:
- Process it as a Map object
- Parse it and use it as a Dart object
The following will be required to be done in case the Map<String, dynamic> is parsed and read as a Dart object.
- A parse method which will parse the Map
- A Dart object which is created as a result of parsing
The following code represents the parse method which is used to create a Dart object.
class PortalInfoWidget extends StatelessWidget {
Map<String, dynamic> _portaInfoMap = {
"name": "Vitalflux.com",
"domains": ["Data Science", "Mobile", "Web"],
"noOfArticles": [
{"type": "data science", "count": 50},
{"type": "web", "count": 75}
]
};
@override
Widget build(BuildContext context) {
PortalInfo portalInfo = PortalInfo.fromJson(_portaInfoMap);
return ListView(
children: List.generate(portalInfo.domains.length,(index){
return Container(
padding: const EdgeInsets.fromLTRB(20.0, 10.0, 10.0, 10.0),
child: Text(portalInfo.domains[index].toString())
);
}),
);
}
}
Note the fromJson factory method called on the PortalInfo (Dart object) which takes the Map<String, dynamic> object and returns the parsed PortalInfo object. A ListView widget is then created on portalInfo.domains object which is List<String> as represented in Map<String, dynamic> JSON representation.
Here is the code for PortalInfo object.
class PortalInfo {
final String name;
final List<String> domains;
final List<Object> noOfArtcles;
PortalInfo({
this.name,
this.domains,
this.noOfArtcles
});
factory PortalInfo.fromJson(Map<String, dynamic> parsedJson){
return PortalInfo(
name: parsedJson['name'],
domains : parsedJson['domains'],
noOfArtcles : parsedJson ['noOfArticles']
);
}
}
Running the app will create a view like the following:
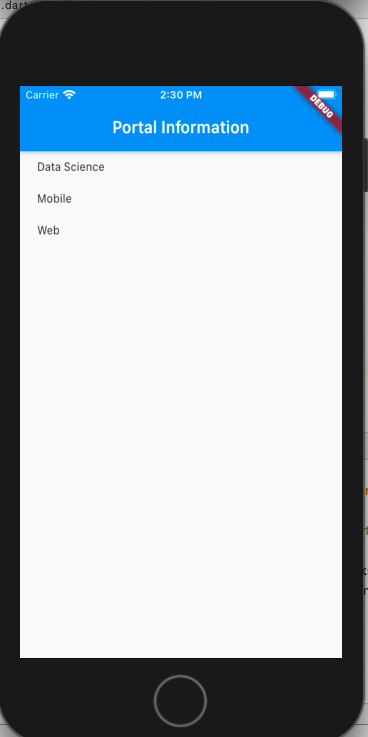
- Mathematics Topics for Machine Learning Beginners - July 6, 2025
- Questions to Ask When Thinking Like a Product Leader - July 3, 2025
- Three Approaches to Creating AI Agents: Code Examples - June 27, 2025
That was amazing man. so as I understood we can show data that comes from server to a list like this Yap?
Thanks