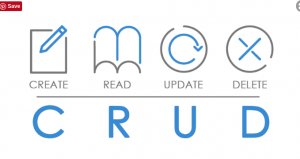
ElasticSearch Java APIs can be used to create, update, query (retrieve items) and delete the index. In this post, you will learn about using Java APIs for performing CRUD operations in relation with managing indices and querying items in ElasticSearch.
- Create an empty index with data type mapping
- Create/update the index using BulkRequest APIs
- Search Index using QueryBuilder and SearchRequestBuilder APIs
- Delete the index
Create an Empty Index with Data-type Mapping
public class App { public static void main(String[] args) { String indexName = "recruitment"; String indexType = "companies"; // // Create an instance of Transport Client // TransportClient client = new PreBuiltTransportClient(Settings.EMPTY) .addTransportAddress(new TransportAddress(InetAddress.getByName("127.0.0.1"), "9300")); // // Create the JSON mapping // String jsonMapping = "{\n" + " \"properties\": {\n" + " \"created_on\": { \"type\": \"date\", \"format\": \"dd-MM-YYYY\" },\n" + " \"name\": { \"type\": \"text\" },\n" + " \"emp_count\": { \"type\": \"integer\" }\n" + " }\n" + " }"; // // Create an empty index // client.admin().indices().prepareCreate(indexName).get(); // // Put the mapping // PutMappingRequest pmr = Requests.putMappingRequest(indexName).type(indexType).source(jsonMapping, XContentType.JSON); client.admin().indices().putMapping(pmr).actionGet(); // // Close the client // client.close(); } }
Create/Update the Index using BulkRequest APIs
Pay attention to some of the following:
- Create an instance of TransportClient
- Prepare bulk request for inserting multiple entries in index
public class App { public static void main(String[] args) { String[] data = {{"vitalflux.com", "15", "15-10-2011"},{"amazon.com", "112110", "10-08-2001"},{"facebook.com", "45123", "16-03-2006"}}; String indexName = "recruitment"; String indexType = "companies"; Map<;String, Object>; source = new HashMap<;String, Object>;(); // // Create an instance of Transport Client // TransportClient client = new PreBuiltTransportClient(Settings.EMPTY) .addTransportAddress(new TransportAddress(InetAddress.getByName("127.0.0.1"), "9300")); // // Prepare Bulk Request // BulkRequestBuilder bulkRequest = client.prepareBulk(); for (String[] entry : data) { source.put("name", entry[0]); source.put("emp_count", entry[1]); source.put("created_on", entry[2]); bulkRequest.add(client.prepareIndex(indexName, indexType).setSource(source)); } // // Invoke the API for creating the index // BulkResponse bulkResponse = bulkRequest.get(); // // Close the client // client.close(); } }
Delete the Index
Pay attention to some of the following:
- Create an instance of TransportClient
- Create an instance of DeleteIndexRequest for deleting an index
public class App { public static void main(String[] args) { String indexName = "recruitment"; // // Create an instance of TransportClient // TransportClient client = new PreBuiltTransportClient(Settings.EMPTY) .addTransportAddress(new TransportAddress(InetAddress.getByName("127.0.0.1"), "9300")); // // Delete the index with name as indexName // DeleteIndexRequest request = new DeleteIndexRequest(indexName); DeleteIndexResponse deleteIndexResponse = this.client.admin().indices().delete(request).actionGet(); } }
Search Index using QueryBuilder and SearchRequestBuilder APIs
Pay attention to some of the following:
- Create an instance of TransportClient
- Create one or more instances of QueryBuilder using APIs such as matchQuery, rangeQuery
- Create an instance of SearchRequestBuilder for building the search request
- Invoke the Get API to get the search results
- Iterate through the search result
public class App { public static void main(String[] args) { String indexName = "recruitment"; String indexType = "companies"; // // Create an instance of TransportClient // TransportClient client = new PreBuiltTransportClient(Settings.EMPTY) .addTransportAddress(new TransportAddress(InetAddress.getByName("127.0.0.1"), "9300")); // // Create instances of QueryBuilders // QueryBuilder nameQueryBuilder = QueryBuilders.matchQuery("name", "faceboak").fuzziness(Fuzziness.ONE).boost(1.0f).prefixLength(0).fuzzyTranspositions(true); QueryBuilder countQueryBuilder = QueryBuilders.rangeQuery("emp_count").from(10000).to(50000); QueryBuilder dateQueryBuilder = QueryBuilders.rangeQuery("created_on").format("yyyy-MM-dd").from("2006-01-01"); // // Create an instance of SearchRequestBuilder; Below represents the single query builder // SearchRequestBuilder requestBuilderWithSingleQueryBuilder = client.prepareSearch(indexName).setTypes(indexType).setQuery(queryBuilder).setSize(100); // // Create Bool Query Builder with different queries // QueryBuilder boolQueryBuilder = QueryBuilders.boolQuery().must(nameQueryBuilder).must(countQueryBuilder).must(dateQueryBuilder); SearchRequestBuilder requestBuilderWithBoolQuery = client.prepareSearch(INDEX_NAME).setTypes(INDEX_TYPE).setQuery(boolQueryBuilder).setSize(1000); // // Get the search result // SearchResponse response1 = requestBuilderWithSingleQueryBuilder.get(); SearchResponse response2 = requestBuilderWithBoolQuery.get(); // // Iterate through search results // SearchHit[] srchHits = response.getHits().getHits(); Object[] result = new Object[srchHits.length]; int i = 0; for (SearchHit srchHit : srchHits) { result[i++] = srchHit.getSourceAsMap(); } // // Print the results // System.out.println("Results Count: " + result.length); for (Object detail : result) { Map<;String, Object>; map = (Map<;String, Object>;) detail; System.out.println("Name: " + map.get("name") + ", Date: " + map.get("created_on") + ", Employee Count: " + map.get("emp_count")); } } }
Further Reading / References
- Field Datatypes
- Built-in Date Formats
- Lucene query string examples
- Lucene query syntax
- Term level queries
- Date range aggregation
You may also some of the following pages in relation to ElasticSearch on our website:
Summary
In this post, you learned about using CRUD operations on indices with ElasticSearch Java APIs.
Did you find this article useful? Do you have any questions or suggestions about this article in relation to working with Elasticsearch Java APIs for doing CRUD operations on indices? Leave a comment and ask your questions and I shall do my best to address your queries.
- Completion Model vs Chat Model: Python Examples - June 30, 2024
- LLM Hosting Strategy, Options & Cost: Examples - June 30, 2024
- Application Architecture for LLM Applications: Examples - June 25, 2024
Leave a Reply