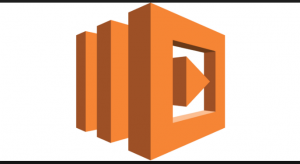
One of the key aspects of AWS Lambda Function in Java is creating deployment package (jar or zip file) for uploading/deploying on AWS Lambda service. In this post, you will learn about different ways in which you could create a Deployment Jar file for deploying it as AWS Lambda project using Maven. The following are different ways:
- Deployment jar using Maven and Eclipse IDE
- Deployment jar using Maven and command prompt
I recommend using Maven and commmand prompt technique for creating deployment jar package.
Before getting started, download AWS Toolkit for Eclipse from Eclipse Marketplace. Here is the information on getting setup with AWS Toolkit for Eclipse
Deployment jar using Maven and Eclipse IDE
The following are steps required to create a AWS Lambda deployment jar file using Eclipse IDE and Maven:
- Create a AWS Lambda Java Project using Eclipse > New > AWS Lambda Java Project
- You will asked to provide project details including input type which is an Amazon services trigger used to invoke the Lambda function.
- Create your function. The following is a sample AWS Lambda function whose input type is an SNS event.
public class LambdaFunctionHandler implements RequestHandler<SNSEvent, String> { @Override public String handleRequest(SNSEvent event, Context context) { context.getLogger().log("Received event: " + event); String message = event.getRecords().get(0).getSNS().getMessage(); context.getLogger().log("From SNS: " + message); return message; } }
- Follow steps mentioned in this page, Creating a Deployment Jar Package Using Maven and Eclipse IDE to create the deployable jar file. Primarily, the following needs to be done:
- From the context menu for the project in Package Explorer, click on Run As > Maven Build…. In the Edit Configuration window, type package in the Goals textfield and submit by clicking RUN.
- This step is needed for creating standalone jar file which contains the compiled customer code, and the resolved dependencies from the pom.xml. Add the maven-shade-plugin plugin by right clicking on pom.xml and clicking on Maven > Add Plugin and adding following details:
- Group Id: org.apache.maven.plugins
- Artifact Id: maven-shade-plugin
- Version: 2.3
- From the context menu for the project in Package Explorer, click on Run As > Maven Build…. In the Edit Configuration window, type package shade:shade in the Goals textfield and submit by clicking RUN.
- You would see two jar files based on your artifactId name and version. For example, if your artifactId name is demo, you would see demo-1.0.0.jar and original-demo-1.0.0.jar.
- Pick the file named as “demo-1.0.0.jar” file for deploying it as AWS Lambda deployment jar file.
- Upload the appropriate jar file as mentioned above in AWS S3 (recommended).
- Provide the link to jar file when creating Lambda function
Deployment jar using Maven Only (Without Eclipse IDE)
- Place the following maven-shade-plugin detail in pom.xml
<plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-shade-plugin</artifactId> <version>3.0.0</version> <configuration> <createDependencyReducedPom>false</createDependencyReducedPom> </configuration> <executions> <execution> <phase>package</phase> <goals> <goal>shade</goal> </goals> </execution> </executions> </plugin>
- Execute the command such as the following:
mvn package
- You would see two jar files based on your artifactId name and version. For example, if your artifactId name is demo, you would see demo-1.0.0.jar and original-demo-1.0.0.jar.
- Pick the file named as “demo-1.0.0.jar” file for deploying it as AWS Lambda deployment jar file.
- Upload the appropriate jar file as mentioned above in AWS S3 (recommended).
- Provide the link to jar file when creating Lambda function
Further Reading / References
- Creating a Deployment Jar Using Maven without any IDE (Java)
- Creating a Deployment Jar Package Using Maven and Eclipse IDE
Summary
In this post, you learned about creating Deployment Jar package for AWS Lambda using Maven.
Did you find this article useful? Do you have any questions or suggestions about this article in relation to techniques used for creating Deployment jar for AWS Lambda? Leave a comment and ask your questions and I shall do my best to address your queries.
- Questions to Ask When Thinking Like a Product Leader - July 3, 2025
- Three Approaches to Creating AI Agents: Code Examples - June 27, 2025
- What is Embodied AI? Explained with Examples - May 11, 2025
I found it very helpful. However the differences are not too understandable for me