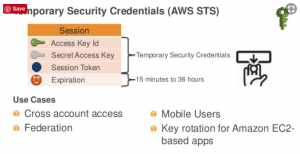
AWS Security Token Service (STS) is an Amazon web service which enables you to request temporary, limited-privilege credentials for AWS Identity and Access Management (IAM) users or for users that you authenticate (federated users). By default, the AWS Security Token Service (AWS STS) is available as a global service, and all AWS STS requests go to a single endpoint at https://sts.amazonaws.com. You can optionally send your AWS STS requests to endpoints in any of the AWS regions shown in the table that follows.
In this post, you would learn how to use AWS security token service to create temporary security credentials with Spring Boot and Java app. I would recommend using AWS STS for creating temporary credentials for development purpose with Amazon/AWS services.
- Configure AWS Credentials
- Create BasicSessionCredentials bean
- Inject/Autowire BasicSessionCredentials Bean to instantiate Amazon Services
- Configure the properties in application.properties
- Run/Test the Temporary Security Credentials
Configure AWS Credentials
Execute command such as the following to configure AWS credentials; This would be used to create temporary security credentials.
aws configure
Make sure to appropriately set the AWS region parameter. Ensure that AWS credentials have been set properly by access the file such as ~/.aws/credentials
Create BasicSessionCredentials Bean
First and foremost, create beans such as SessionCrendentials which is used for creating temporary credentials. The following are some of the key steps in creating the session credential bean:
- Create an instance of AWSSecurityTokenServiceClient
- Get session token request object; Set credentials validity duration
- Create an instance of GetSessionTokenResult using session token object
- Create an instance of BasicSessionCredentials using the information such as access key id, access key secret and session token result object.
- Return the instance of BasicSessionCredentials as bean
@Configuration @PropertySource("classpath:application.properties") public class AppConfig { private static final Integer TEMPORARY_CREDENTIALS_DURATION_DEFAULT = 7200; @Value("${aws.region}") String awsRegion; @Value("${aws.s3.data.bucket}") String awsS3DataBucket; @Value("${aws.temporary.credentials.validity.duration}") String credentialsValidityDuration; @Bean(name = "awsRegion") public Region awsRegion() { return Region.getRegion(Regions.fromName(awsRegion)); } @Bean(name = "sessionCredentials") public BasicSessionCredentials sessionCredentials() { // // Create an instance of AWSSecurityTokenServiceClient // AWSSecurityTokenServiceClient sts_client = (AWSSecurityTokenServiceClient) AWSSecurityTokenServiceClientBuilder.defaultClient(); // // Get session token request object; Set credentials validity duration // GetSessionTokenRequest session_token_request = new GetSessionTokenRequest(); if(this.credentialsValidityDuration == null || this.credentialsValidityDuration.trim().equals("")) { session_token_request.setDurationSeconds(TEMPORARY_CREDENTIALS_DURATION_DEFAULT); } else { session_token_request.setDurationSeconds(Integer.parseInt(this.credentialsValidityDuration)); } // // Create an instance of GetSessionTokenResult using session token object // GetSessionTokenResult session_token_result = sts_client.getSessionToken(session_token_request); Credentials session_creds = session_token_result.getCredentials(); // // Create an instance of BasicSessionCredentials // BasicSessionCredentials sessionCredentials = new BasicSessionCredentials( session_creds.getAccessKeyId(), session_creds.getSecretAccessKey(), session_creds.getSessionToken()); return sessionCredentials; } @Bean(name = "awsS3DataBucket") public String awsS3DataBucket() { return awsS3DataBucket; } }
Inject/Autowire BasicSessionCredentials Bean to Instantiate Amazon Services
The code given below uses BasicSessionCredentials Bean to instantiate the Amazon S3 service.
@Component("awsCloudStorage") public class AWSCloudStorage implements CloudStorage { @Autowired String awsS3DataBucket; private AmazonS3 amazonS3; public AWSCloudStorage(@Autowired Region awsRegion, @Autowired BasicSessionCredentials sessionCredentials) { this.amazonS3 = AmazonS3ClientBuilder.standard() .withCredentials(new AWSStaticCredentialsProvider(sessionCredentials)).build(); } public void uploadFile(String keyName, String filePath) { try { this.amazonS3.putObject(this.awsS3DataBucket, keyName, filePath); } catch (AmazonServiceException e) { System.err.println(e.getErrorMessage()); } } }
Pay attention to the following code which is used to create Amazon S3 instance:
this.amazonS3 = AmazonS3ClientBuilder.standard() .withCredentials(new AWSStaticCredentialsProvider(sessionCredentials)).build();
Amazon S3 instance could as well be created with region using code such as following:
this.amazonS3 = AmazonS3ClientBuilder.standard() .withRegion(awsRegion.getName()) .withCredentials(new AWSStaticCredentialsProvider(sessionCredentials)).build();
Configure the properties in application.properties
You could provide configuration details such as temporary credentials validity duration, aws region etc in the src/main/resources/application.properties file. The following is the sample code:
aws.access.key.id = aws.access.key.secret = aws.region = us-east-1 aws.s3.data.bucket = remainders-25022018 aws.temporary.credentials.validity.duration =
Run/Test the Temporary Security Credentials
The following represents the Spring Boot app code which tests the AWSCloudStorage which is instantiated using temporary credentials as shown in the code given above.
@SpringBootApplication public class RecruiterbotApplication implements CommandLineRunner { @Autowired CloudStorage awsCloudStorage; public static void main(String[] args) { SpringApplication app = new SpringApplication(RecruiterbotApplication.class); app.run(args); } @Override public void run(String... arg0) throws IOException, JavaLayerException { this.awsCloudStorage.uploadFile("message123.txt", "/home/support/Documents/corpus.lm"); } }
Further Reading/References
- AWS Security Token Service
- Activating and Deactivating AWS STS in an AWS Region
- Set up AWS Credentials and Region for Development
- Getting temporary credentials using AWS STS
- Bots using Spring Boot & Java: This is the place where above code could be found on Github.
Summary
In this post, you learned about getting started with using temporary security credentials for accessing Amazon/AWS services from Spring Boot, Java app.
Did you find this article useful? Do you have any questions or suggestions about this article in relation to getting started with temporary security credentials using AWS STS? Leave a comment and ask your questions and I shall do my best to address your queries.
- Three Approaches to Creating AI Agents: Code Examples - June 27, 2025
- What is Embodied AI? Explained with Examples - May 11, 2025
- Retrieval Augmented Generation (RAG) & LLM: Examples - February 15, 2025
I found it very helpful. However the differences are not too understandable for me