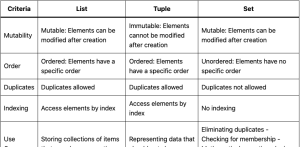
When working with Python programming, data structures play a crucial role in organizing and manipulating data efficiently. Among several data structures available, lists, tuples, and sets are three fundamental ones that every Python programmer/developer should understand. Lists, tuples, and sets are unique in terms of their properties and functionality, making them most appropriate for different scenarios. Not only are these data structures most frequently used in everyday programming tasks, but they are also frequently asked about in interviews with data analysts and data scientists. Therefore, grasping the concepts of lists, tuples, and sets becomes essential. In this blog, we will delve deeper into the specifics of lists, tuples, and sets, discussing their characteristics, use cases, and practical examples.
What are Lists, Tuples & Sets in Python Programming?
In Python, data structures are essential tools for organizing and manipulating data effectively. Three commonly used data structures in Python are lists, tuples, and sets. Each of these structures has its unique characteristics and use cases. Let’s look into each of these data structures and learn different aspects of them.
List in Python
A list is an ordered collection of elements, enclosed in square brackets ([ ]). It is mutable, which means you can modify its elements after creation. Lists are versatile and widely used due to their flexibility. Let’s explore an example to understand this better.
Imagine you’re building a task management application. You can use a list to store the tasks entered by the user. Each task can be represented as a string. Here’s how you can create a list of tasks:
# List
tasks = ["Complete project", "Review presentation", "Send email"]
We can access individual tasks by their index and perform operations like adding, removing, or updating tasks in the list. Lists are suitable when you need an ordered collection that can be modified. Here is the Python code for performing different operations with List data structures such as accessing, adding, removing, and updating an element in the list.
# Sample list
tasks = ["Complete project", "Review presentation", "Send email"]
# Accessing tasks by index
print("Task at index 0:", tasks[0])
print("Task at index 1:", tasks[1])
print("Task at index 2:", tasks[2])
# Adding a new task
tasks.append("Submit report")
print("Updated tasks:", tasks)
# Removing a task
removed_task = tasks.pop(1)
print("Removed task:", removed_task)
print("Updated tasks:", tasks)
# Updating a task
tasks[0] = "Finish project"
print("Updated tasks:", tasks)
Here is the output of the above code would look like:

Tuples in Python
A tuple is an ordered collection of elements, enclosed in parentheses ( ). Unlike lists, tuples are immutable, meaning their elements cannot be changed after creation. Tuples are useful when you want to store a sequence of values that should not be modified. Let’s look at an example to understand this further.
Suppose you’re building an application to track the weather forecast for different cities. You can use tuples to store the weather information for each city, such as the city name, temperature, and weather conditions. Here’s how you can represent this data using tuples:
# Tuple
city1 = ("New York", 75, "Sunny")
city2 = ("London", 62, "Cloudy")
city3 = ("Mumbai", 85, "Rainy")
In the above example, each tuple represents the weather information for a specific city. Once created, the elements of a tuple cannot be modified. Tuples are suitable when you need to store data that should remain constant. The following represents the operations you can perform with the tuples.
city1 = ("New York", 75, "Sunny")
# Accessing elements of the tuple
print("City:", city1[0])
print("Temperature:", city1[1])
print("Weather condition:", city1[2])
# Unpacking the tuple into variables
city, temperature, weather = city1
print("City:", city)
print("Temperature:", temperature)
print("Weather condition:", weather)
# Concatenating tuples
city2 = ("London", 62, "Cloudy")
combined_cities = city1 + city2
print("Combined cities:", combined_cities)
# Length of a tuple
print("Length of the tuple:", len(city1))
# Checking for an element in the tuple
print("Is 'Sunny' in the tuple?", "Sunny" in city1)
# Counting occurrences of an element in the tuple
print("Occurrences of 'New York':", city1.count("New York"))
# Finding the index of an element in the tuple
print("Index of '75':", city1.index(75))
The following is how the output would look like:
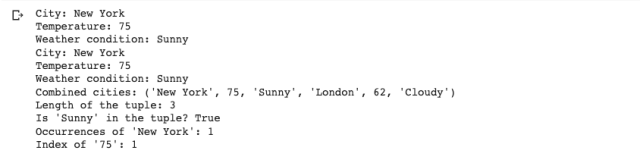
Set in Python
A set in Python is an unordered collection of unique elements, enclosed in curly braces {}. Sets do not allow duplicate values, and their elements are not indexed. Sets provide efficient membership testing and operations like union, intersection, and difference. Let’s see a real-world example to understand sets better.
Consider an online shopping platform where customers can browse and add products to their cart. You can use sets to store the unique items in a customer’s cart. This way, you can ensure that each product is added only once, regardless of how many times the customer attempts to add it. Here’s how you can represent the customer’s cart using sets:
# Set
cart = {"Shirt", "Jeans", "Sunglasses", "Shoes"}
Sets automatically remove duplicate items, making them suitable for scenarios that require uniqueness, such as eliminating duplicate entries in data or checking membership efficiently. The following code represents some of the operations that you could perform with one or more elements in the set.
cart = {"Shirt", "Jeans", "Sunglasses", "Shoes"}
# Adding an element to the set
cart.add("Hat")
print("Updated cart:", cart)
# Removing an element from the set
cart.remove("Jeans")
print("Updated cart:", cart)
# Checking for an element in the set
print("Is 'Shoes' in the cart?", "Shoes" in cart)
# Set union
additional_items = {"Socks", "Belt"}
updated_cart = cart.union(additional_items)
print("Updated cart after union:", updated_cart)
# Set intersection
common_items = cart.intersection(updated_cart)
print("Common items:", common_items)
# Set difference
removed_items = cart.difference(updated_cart)
print("Removed items:", removed_items)
# Set symmetric difference
unique_items = cart.symmetric_difference(updated_cart)
print("Unique items:", unique_items)
# Clearing the set
cart.clear()
print("Cleared cart:", cart)
Here is how the output of the code would look like:

When to Use List, Tuple & Set in Python?
When working with data in Python, choosing the appropriate data structure such as list, tuple, and set is crucial for efficient programming and data manipulation. Understanding the differences between these data structures is essential for making informed decisions and optimizing your code. In this section, we will compare lists, tuples, and sets in Python, highlighting their features, pros, and cons, to help you choose the right one for your specific needs.
Criteria | List | Tuple | Set |
---|---|---|---|
Mutability | Mutable: Elements can be modified after creation | Immutable: Elements cannot be modified after creation | Mutable: Elements can be modified after creation |
Order | Ordered: Elements have a specific order | Ordered: Elements have a specific order | Unordered: Elements have no specific order |
Duplicates | Duplicates allowed | Duplicates allowed | Duplicates not allowed |
Indexing | Access elements by index | Access elements by index | No indexing |
Use Cases | Storing collections of items that can change over time | Representing data that should not change | Eliminating duplicates – Checking for membership – Mathematical operations (union, intersection) |
Pros | Mutable and flexible | Immutable and safe | Efficient duplicate removal; Operation such as whether a member is available in the set is faster |
Cons | Operations such as whether a member is present in the list is slower compared to sets | Elements cannot be modified | Elements have no specific order – No indexing |
In summary, lists are suitable for storing collections of items that can change over time and require indexing. Tuples, on the other hand, are useful for representing data that should not change, ensuring data integrity. Sets excel in eliminating duplicates and performing efficient membership testing, making them ideal for scenarios that require unique elements or set operations.
- Logistic Regression in Machine Learning: Python Example - April 26, 2024
- MSE vs RMSE vs MAE vs MAPE vs R-Squared: When to Use? - April 25, 2024
- Gradient Descent in Machine Learning: Python Examples - April 22, 2024
Leave a Reply