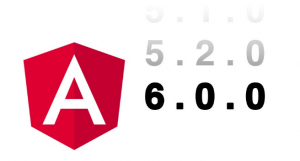
In this post, you will learn about How to update or migrate your Angular 5 app (using Angular 5.1 or Angular 5.2 version) to use Angular 6.0.0. The following are some of the points covered:
- Pre-requisites
- Upgrade/Update steps for migrating Angular 5 to Angular 6.0.0 app.
Pre-requisites
The following needs to be done before you start on the migration:
- Make sure your NodeJS version is Node 8 or later.
- Make sure your code used HttpClientModule and the HttpClient service instead of HttpModule and Http service.
- Make sure to import animation services from @angular/animations rather than @angular/core.
Upgrade/update Angular 5.* to Angular 6.0.0
The following commands need to be executed as part of migrating Angular 5.1 or Angular 5.2 app to Angular 6.*.
- Update Angular CLI globally and locally: The following command will update Angular CLI globally (-g option) and locally.
sudo npm install -g @angular/cli sudo npm install @angular/cli
- Update package.json: Execute the following command to update the package.json file with Angular 6.* changes.
sudo ng update @angular/cli
This is how my package.json file looked before executing above command. Note some of the following changes:
- All of the dependencies and devDependencies is updated from version 5.2.* to version 6.0.0
- rxjs is migrated from version 5.5.6 to version 6.1.0
- typescript is migrated from version 2.5.3 to version 2.7.2
{ "name": "rbot-ui", "version": "0.0.0", "license": "MIT", "scripts": { "ng": "ng", "start": "ng serve", "build": "ng build --prod", "test": "ng test", "lint": "ng lint", "e2e": "ng e2e" }, "private": true, "dependencies": { "@angular/animations": "^5.2.10", "@angular/common": "^5.2.0", "@angular/compiler": "^5.2.0", "@angular/core": "^5.2.0", "@angular/forms": "^5.2.0", "@angular/http": "^5.2.0", "@angular/platform-browser": "^5.2.0", "@angular/platform-browser-dynamic": "^5.2.0", "@angular/router": "^5.2.0", "@angular/service-worker": "^5.2.0", "core-js": "^2.4.1", "rxjs": "^5.5.6", "zone.js": "^0.8.19" }, "devDependencies": { "@angular/cli": "~1.7.3", "@angular/compiler-cli": "^5.2.0", "@angular/language-service": "^5.2.0", "@types/jasmine": "~2.8.3", "@types/jasminewd2": "~2.0.2", "@types/node": "~6.0.60", "codelyzer": "^4.0.1", "jasmine-core": "~2.8.0", "jasmine-spec-reporter": "~4.2.1", "karma": "~2.0.0", "karma-chrome-launcher": "~2.2.0", "karma-coverage-istanbul-reporter": "^1.2.1", "karma-jasmine": "~1.1.0", "karma-jasmine-html-reporter": "^0.2.2", "protractor": "~5.1.2", "ts-node": "~4.1.0", "tslint": "~5.9.1", "typescript": "~2.5.3" } }
This is how the new package.json file looked like after executing ng update command.
{ "name": "rbot-ui", "version": "0.0.0", "license": "MIT", "scripts": { "ng": "ng", "start": "ng serve", "build": "ng build --prod", "test": "ng test", "lint": "ng lint", "e2e": "ng e2e" }, "private": true, "dependencies": { "@angular/animations": "6.0.0", "@angular/common": "6.0.0", "@angular/compiler": "6.0.0", "@angular/core": "6.0.0", "@angular/forms": "6.0.0", "@angular/http": "6.0.0", "@angular/platform-browser": "6.0.0", "@angular/platform-browser-dynamic": "6.0.0", "@angular/router": "6.0.0", "@angular/service-worker": "6.0.0", "core-js": "^2.4.1", "rxjs": "^6.1.0", "zone.js": "~0.8.26" }, "devDependencies": { "@angular/cli": "6.0.0", "@angular/compiler-cli": "6.0.0", "@angular/language-service": "6.0.0", "@types/highcharts": "^5.0.22", "@types/jasmine": "~2.8.3", "@types/jasminewd2": "~2.0.2", "@types/node": "~6.0.60", "codelyzer": "^4.0.1", "jasmine-core": "~2.8.0", "jasmine-spec-reporter": "~4.2.1", "karma": "~2.0.0", "karma-chrome-launcher": "~2.2.0", "karma-coverage-istanbul-reporter": "^1.2.1", "karma-jasmine": "~1.1.0", "karma-jasmine-html-reporter": "^0.2.2", "protractor": "~5.1.2", "ts-node": "~4.1.0", "tslint": "~5.9.1", "typescript": "2.7.2" } }
- Update @Angular/Core: Execute the following command to update Angular core.
sudo ng update @angular/core
- Update @Angular/Material: In case you used Angular Material in your project, execute following command to update @angular/material.
sudo ng update @angular/material
- Update other dependencies: Execute command such as ng update to identify and update other dependencies. If all is good, you should see the following message: We analyzed your package.json and everything seems to be in order. Good work!
- Update @Angular/CLI from 1.7.3 to Latest: Angular 6.0.0 requires angular.json file. In case, you used Angular-cli versions of 1.7.*, you would have to upgrade it. Or else, you would get the error such as Local workspace file (‘angular.json’) could not be found.
sudo ng update @angular/cli --migrate-only --from=1.7.3
- In case, Typescript is configured with script = true, you would want to put following within compiler options in tsconfig.json
"compilerOptions": { ... "strictPropertyInitialization": false }
With above, you should be all set. Execute the command, ng serve and make sure the app compiles.
You may see the error related to has no exported member ‘Observable’. You could get rid of such errors using the following commands:
- Remove deprecated RxJS 6 features by executing the following commands:
npm install -g rxjs-tslint rxjs-5-to-6-migrate -p src/tsconfig.app.json
- Remove rxjs-compat by executing the following command:
npm uninstall rxjs-compat
You may still see errors such as has no exported member ‘Observable’ in one or more of the packages. You would be required to upgrade those packages to the higher version using Angular 6.0.0. Thus, I would recommend you still wait for a while before going for the upgrade.
References
Summary
In this post, you learned about how to migrate Angular 5.1 or Angular 5.2 app to use Angular 6.0.0.
Did you find this article useful? Do you have any questions or suggestions about this article? Leave a comment and ask your questions and I shall do my best to address your queries.
- Logistic Regression in Machine Learning: Python Example - April 26, 2024
- MSE vs RMSE vs MAE vs MAPE vs R-Squared: When to Use? - April 25, 2024
- Gradient Descent in Machine Learning: Python Examples - April 22, 2024
Leave a Reply