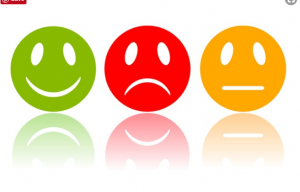
Sentiment analysis of a text document such as speech, articles on websites etc is about assessing sentiments associated with the document as a function of overall emotions expressed in form of different words. Sentiment analysis is primarily used for tracking voice of customer (VOC) by analyzing customer reviews, survey responses, etc., in social media websites such as Facebook, Twitter etc. The VOC can be related to products in general, an event, movies etc.
In this post, you will learn about how to use Google Cloud NLP API for performing sentiment analysis of a text document. Java code is used for programming the sentiment analysis.
Google NLP API – Sentiment Analysis Metrics
Google NLP API helps to measure positive and negative emotions related to a document. Thus, a document with sentiments such as sad or angry or hatred would be labeled as “negative”. The document with sentiments as happy or good or great would be labeled as positive. The sentiment having positive or negative emotions is scored in terms of values greater than or lesser than 0 respectively. Thus, the following holds true:
- Positive emotion score (greater than 0 to +1): A document with positive emotion would have the value greater than 0 up to 1.
- Negative emotion score (less than 0 to -1): A document with negative emotion would have value lesser than 0 up to -1.
- Neutral score (0): A document with the score as 0 would be considered as neutral. That essentially means that the document does not have any emotion or have mixed emotions (both positive and negative) adding up to zero. In case of neutral documents, the value of magnitude can be used to provide greater clarification in relation to the amount of emotional content present in the document. A truly neutral document will have a low magnitude value, while documents with mixed sentiments will have higher magnitude values.
The following is a sample set of sentiment analysis score vs magnitude value vis-a-vis document sentiment analysis outcome:
Score | Magnitude | Outcome |
---|---|---|
0.5 | 5.0 | Largely positive |
-0.1 | 0.0 | Neutral |
-0.65 | 3.0 | Largely Negative |
0.1 | 4.0 | Mixed emotions |
Google NLP Sentiment Analysis API
Google NLP API, analyzeSentiment is used for measuring sentiments associated with a document. When invoked on an instance of LanguageServiceClient, it returns an object of type Sentiment. The following are two APIs on Sentiment object which are used for measuring the sentiments:
- getScore(): Returns emotion score in the range of -1 to +1. As mentioned above, a score less than 0 up to -1 implies negative emotion and a score more than 0 up to +1 implies positive emotions.
- getMagnitude(): Magnitude is used to measure the amount of emotional content found in the text. Its value can range from 0 to infinity.
Google NLP Sentiment Analysis Code Example
Before running following Java code within your Eclipse IDE, make sure you are set up with Google NLP. Refer to this page, How to Create Java NLP Apps using Google NLP API.
The following code performs sentiment analysis of an article written on AUTO Expo 2018 currently underway in Greater Noida.
package com.vflux.nlpdemo; import com.google.cloud.language.v1.Document; import com.google.cloud.language.v1.LanguageServiceClient; import com.google.cloud.language.v1.Sentiment; import com.google.cloud.language.v1.Document.Type; public class SentimentAnalysis { public static void main(String... args) throws Exception { // Instantiates a client String text = "With the Modi government keen on making India a 100% electric vehicle nation by 2030, automakers too are shifting their focus to electric vehicles, a glimpse of which is being witnessed at the ongoing Auto Expo 2018 in Greater Noida. In fact, while dozens of electric cars are being showcased by auto majors here, many have even forged new partnerships in a bid to introduce electric cars over the next few years. Experts say that the demand for electric vehicles (EVs) is increasing day by day and it will continue to grow faster in the years to come, driven by stricter emission norms and growing consumer awareness. In fact, sales of EV vehicles are likely to witness high double-digit growth rates annually in India till 2020, as per a recent study. However, apart from higher price, there are a few other things to keep in mind while going for an electric vehicle. One is insurance. Are you aware that you may have to shell out more for getting an electric vehicle insured? According to a recent report by the UK-based The Telegraph, drivers swapping gas-guzzling cars for greener electric vehicles face paying nearly 50% higher insurance bills. Although no such data is available in India, but industry sources say that here too getting an electric vehicle insured may be costlier as per global norms. Is electric car insurance available in India? The biggest question is: Is electric car insurance available in India? Insurers say as the market of electric vehicle is at a nascent stage in India, electric cars here are covered under the traditional car insurance policies that also cover petrol and diesel cars. However, the general problem that is faced while applying for an insurance policy is that there is no defined engine and cubic capacity of electric cars and the premium while applying for an insurance cover is basically based on the cubic capacity (CC) of the vehicle as per the guidelines laid by India Motor Tariff. Therefore, it is crucial to categorise cubic capacity of electric cars as per the guidelines to underwrite the premium, says Tarun Mathur, Director, Policybazaar.com, adding that for example, Mahindra’s Reva is categorised to be a 1000 cubic capacity car and hence its insurance cover is available in the market."; try (LanguageServiceClient language = LanguageServiceClient.create()) { // The text to analyze Document doc = Document.newBuilder().setContent(text).setType(Type.PLAIN_TEXT).build(); // Detects the sentiment of the text Sentiment sentiment = language.analyzeSentiment(doc).getDocumentSentiment(); System.out.printf("Text: %s%n", text); System.out.printf("Sentiment: %s, %s%n", sentiment.getScore(), sentiment.getMagnitude()); } } }
As the above code is run, the score comes as +0.1 and magnitude come as +3.0. This makes the document slightly more than neutral with mixed emotions.
Use the following text and you would see the sentiment score and magnitude as -0.2 and +3.0 respectively. This represents that document looks to have the negative emotion.
Thousands of motorists could be unwittingly driving around without valid car insurance, police have warned after identifying a worrying new scam known as ‘ghost broking’. With premiums soaring, fraudsters have been taking advantage of car owners’ desperation to find the best deal. Using popular social media sites, such as Facebook and Instagram, they pose as legitimate brokers working for reputable insurance firms, and contact motorists directly, offering to save them hundreds of pounds on their renewal. But the policy documents they produce are worthless and many victims only becoming aware they have been duped, when they have an accident and attempt to make a claim. To their horror they then discover they are liable for the entire cost of the accident, which can run into many thousands of pounds
Further Reading
Summary
In this post, you learned about details and code examples related to using Java for doing sentiment analysis using Google NLP APIs.
Did you find this article useful? Do you have any questions or suggestions about this article in relation to sentiment analysis using Google NLP API? Leave a comment and ask your questions and I shall do my best to address your queries.
- Mathematics Topics for Machine Learning Beginners - July 6, 2025
- Questions to Ask When Thinking Like a Product Leader - July 3, 2025
- Three Approaches to Creating AI Agents: Code Examples - June 27, 2025
I found it very helpful. However the differences are not too understandable for me