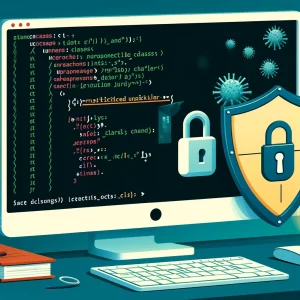
Suppose your machine learning model is serialized as a Python pickle file and later loaded for making predictions. In that case, you need to be aware of security risks/issues associated with loading the Python Pickle file.
Security Issue related to Python Pickle
The Python pickle module is a powerful tool for serializing and deserializing Python object structures. However, its very power is also what makes it a potential security risk. When data is “pickled,” it is converted into a byte stream that can be written to a file or transmitted over a network. “Unpickling” this data reconstructs the original object in memory. The danger lies in the fact that unpickling data from an untrusted source can execute arbitrary code embedded in the pickle file, potentially leading to severe security breaches.
Reducing Security Risks in the Pickling Process
To mitigate the security risks associated with pickling, here are several strategies one can employ:
- Use Alternatives to Pickle:
- JSON: For many use cases, JSON is a safer alternative to pickle. JSON serialization is text-based and does not execute code, thus reducing the risk of code injection attacks.
- XML: Another alternative is XML, which, like JSON, does not allow arbitrary code execution during the deserialization process.
- Restrict the Use of Pickle:
- Only use pickle for data serialization within a trusted and controlled environment. Avoid using pickle for data received from untrusted or unknown sources.
- Isolate the Unpickling Process:
- If you must unpickle data from an untrusted source, do so in a secure, isolated environment. This can be achieved by using:
- Virtual Machines (VMs): Create a virtual machine specifically for the unpickling process. This VM should not have access to sensitive data or network resources.
- Docker Containers: Use Docker to create a containerized environment for unpickling. Containers provide a lightweight and easily disposable environment that can be tightly controlled and restricted.
- If you must unpickle data from an untrusted source, do so in a secure, isolated environment. This can be achieved by using:
- Limit the Scope of Objects That Can Be Unpickled:
- Use a custom Unpickler class that overrides the find_class method to restrict the types of objects that can be unpickled. This approach ensures that only safe, known object types are deserialized.
Example: Using a Custom Unpickler
Here is an example of how you can implement a custom unpickler to limit the types of objects that can be deserialized. In the code below, the RestrictedUnpickler class inherits from pickle.Unpickler, the standard class used to unpickle objects. The find_class method is overridden to control which classes can be instantiated during the unpickling process. A set called safe_classes is defined to include only safe and commonly used built-in types: list, dict, str, int.
If the class is deemed safe, the method delegates to the superclass (super().find_class(module, name)) to complete the unpickling process for that class.
import pickle
class RestrictedUnpickler(pickle.Unpickler):
def find_class(self, module, name):
# Only allow safe modules and classes
safe_classes = {
('builtins', 'list'),
('builtins', 'dict'),
('builtins', 'str'),
('builtins', 'int'),
}
if (module, name) not in safe_classes:
raise pickle.UnpicklingError(f"Attempting to unpickle unsafe class {module}.{name}")
return super().find_class(module, name)
def restricted_loads(data):
return RestrictedUnpickler(io.BytesIO(data)).load()
# Example usage
try:
data = restricted_loads(serialized_data)
except pickle.UnpicklingError as e:
print(f"Security error: {e}")
Conclusion
The pickle module’s ability to serialize and deserialize complex Python objects comes with significant security risks, particularly when dealing with data from untrusted sources. By employing safer alternatives, isolating the unpickling process, and restricting the scope of objects that can be unpickled, developers can significantly reduce these risks and protect their applications from potential exploits.
- Retrieval Augmented Generation (RAG) & LLM: Examples - February 15, 2025
- How to Setup MEAN App with LangChain.js - February 9, 2025
- Build AI Chatbots for SAAS Using LLMs, RAG, Multi-Agent Frameworks - February 8, 2025
I found it very helpful. However the differences are not too understandable for me