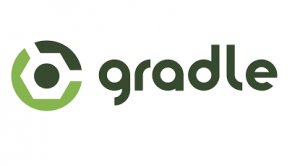
The article describes the configuration one would need to create WAR file for an Eclipse-base web application (Dynamic Web) project.
Below code represents whats needed to compile your Java Eclipse-based web application project and create WAR file which can be deployed later, on any Java server such as Tomcat. Pay attention to some of the following facts:
- repositories: “repositories” is referred in a local directory rather than external repository such as MavenCentral etc.
- sourceSets: sourceSets define the path under which Java source code exists. It MUST BE NOTED that failing to define this would have Gradle look into Maven-based folder such as “src/main/java” for source code that would eventually result into failure. This primarily because Eclipse-based dynamic web projects place the Java code under “src” folder.
- dependencies: Compile time dependencies is referred from within local directory.
- war: Following are key war-related configurations:
- WebInf is used to store files within WebContent/WEB-INF folder
- classpath is used to copy libraries within WebContent/WEB-INF/lib folder
- WebXML is used to locate path to web.xml (WebContent/WEB-INF/web.xml)
- from { path } is used to copy files under WebContent folder. These are files such as static resources, html/jsp files if any.
apply plugin: 'java'
apply plugin: 'war'
// Depending upon the fact that one uses default
// WebContent folder to store web resources. Change
// name to name of WebContent folder appropriately.
def webInfPath = "WebContent/WEB-INF"
// Repositories accessed from local directory
repositories {
flatDir { dirs webInfPath + "/" + "lib" }
}
// Source folder defined as Eclipse stores Java source code
// in non-maven based folder structure
sourceSets {
main {
java { srcDir 'src' }
}
}
dependencies {
// Compile time dependencies read from local directory
compile fileTree(dir: webInfPath + "/" + "lib", include: '*.jar')
}
war {
webInf { from webInfPath} // adds a file-set to the WEB-INF dir.
classpath fileTree(webInfPath + "/" + "lib") // adds a file-set to the WEB-INF/lib dir.
webXml = file(webInfPath + "/" + "web.xml") // copies a file to WEB-INF/web.xml
from('WebContent') { include ('bootstrap/css/*.css', 'bootstrap/js/*.js', 'bootstrap/img/*.*') } //Copy Bootstrap folder to WEB-INF level
}
[adsenseyu1]
Latest posts by Ajitesh Kumar (see all)
- What is Embodied AI? Explained with Examples - May 11, 2025
- Retrieval Augmented Generation (RAG) & LLM: Examples - February 15, 2025
- How to Setup MEAN App with LangChain.js - February 9, 2025
I have a small problem connected to Gradle and web plugin.
I got spring mvc resources imported to my web project. But after running my web project under Tomcat, it seems that I have no src/main/resources files under my classpath. I was expecting gradle to take care of it, but it seems I have to config war manually. Any hints on this?