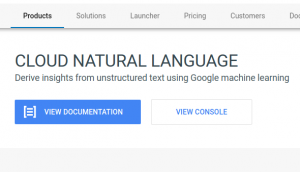
Natural language processing (NLP) is an AI-based technology which is used for creating apps related to speech recognition, natural-language understanding, and natural-language generation. Some of the applications related to NLP are content classification, sentiment analysis, syntactic analysis etc.
In this post, you will learn about how to get set up with a development environment for creating NLP based apps using Google Cloud NLP APIs.
Setup Eclipse-based Development Environment for Google NLP API
The steps below would help you get setup with Eclipse IDE and Java-based development environment for developing apps using Google Cloud Natural Language API.
- Create Google Project: Create a project by logging into Google Cloud console. I created a project such as NLP-Sample. Select the project that you just created.
- Go to Cloud NLP API Page: From Google cloud console, go to Google Natural Language API page by searching using the keyword such as “google cloud natural language API” as shown in the diagram below.
Alternatively, go to Google Cloud Natural Language API page and select the project you created in the previous step.
- Enable Cloud NLP API: Enable the Google cloud NLP API by clicking on Enable button. The following screenshot represents ENABLE button.
- Create Service Account Key: Create Service account key using which you will access the Google NLP APIs from your Java programs. From Google NLP dashboard, click on “Credentials” link as shown in the left navigation. Then, click on “create credentials” and select service account key.
Enter the details in the form as shown in the diagram below and submit.
This would download the service account key in a JSON file. The service account key file looks like following:
{ "type": "service_account", "project_id": "nlp-sample-194807", "private_key_id": "6fcfbf135fd46c60256453621919d60ecea5564b", "private_key": "-----BEGIN PRIVATE KEY-----\nMIIEv...XXkhk=\n-----END PRIVATE KEY-----\n", "client_email": "demo-57@nlp-sample-345807.iam.gserviceaccount.com", "client_id": "118019199276547406057", "auth_uri": "https://accounts.google.com/o/oauth2/auth", "token_uri": "https://accounts.google.com/o/oauth2/token", "auth_provider_x509_cert_url": "https://www.googleapis.com/oauth2/v1/certs", "client_x509_cert_url": "https://www.googleapis.com/robot/v1/metadata/x509/demo-57%40nlp-sample-346807.iam.gserviceaccount.com" }
- Create a Maven Project: Create a Maven project in Eclipse
- Include Google Cloud NLP API Dependency in POM: Put following in pom.xml file.
<dependency> <groupId>com.google.cloud</groupId> <artifactId>google-cloud-language</artifactId> <version>1.14.0</version> </dependency>
- Configure Google Application Credentials: Configure GOOGLE_APPLICATION_CREDENTIALS as environment variable whose value is the file path for service account key which gets downloaded as a result of creating credential as service-account-key.
Figure . Configure Eclipse Project Runtime Environment Variable
- Test a sample Java app related to content classification: Place following code in App.java
package com.vflux.nlpdemo; import com.google.cloud.language.v1.ClassificationCategory; import com.google.cloud.language.v1.ClassifyTextRequest; import com.google.cloud.language.v1.ClassifyTextResponse; import com.google.cloud.language.v1.Document; import com.google.cloud.language.v1.Document.Type; import com.google.cloud.language.v1.LanguageServiceClient; public class App { public static void main(String... args) throws Exception { String text = "The two sides also signed agreements worth around USD 50 million that includes setting up of a USD 30 million super specialty hospital after Palestinian President Mahmoud Abbas received Prime Minister Modi in an official ceremony at the presidential compound, also known as Muqata'a, in Ramallah - the Palestinian seat of government. On his part, President Abbas acknowledged that the Indian leadership has always stood by peace in Palestine. Abbas said Palestine is always ready to engage in negotiations to achieve its goal of an independent state. He asked India to facilitate the peace process with Israel. We rely on India's role as an international voice of great standing and weigh through its historical role in the Non-Aligned Movement and in all international forum and its increasingly growing power on the strategic and economic levels, in a way that is conducive to just and desired peace in our region, said President Abbas. The two sides signed agreements worth USD 50 million. The agreement includes setting up of a super speciality hospital worth USD 30 million in Beit Sahur and construction of a centre for empowering women worth USD 5 million. Three agreements in the education sector worth USD 5 million and for procurement of equipment and machinery for the National Printing Press in Ramallah were also signed."; try (LanguageServiceClient language = LanguageServiceClient.create()) { // set content to the text string Document doc = Document.newBuilder() .setContent(text) .setType(Type.PLAIN_TEXT) .build(); ClassifyTextRequest request = ClassifyTextRequest.newBuilder() .setDocument(doc) .build(); // detect categories in the given text ClassifyTextResponse response = language.classifyText(request); for (ClassificationCategory category : response.getCategoriesList()) { System.out.printf("Category name : %s, Confidence : %.3f\n", category.getName(), category.getConfidence()); } } } }
- Run the above program and you would get the following output:
Category name : /News, Confidence : 0.550 Category name : /Law & Government/Government, Confidence : 0.500
Further Reading / Reference
Summary
In this post, you learned about how to get set up with Google Cloud Natural Language API with Java.
Did you find this article useful? Do you have any questions or suggestions about this article in relation to setting up the development environment for Google cloud natural language API using Eclipse IDE and Java? Leave a comment and ask your questions and I shall do my best to address your queries.
- Completion Model vs Chat Model: Python Examples - June 30, 2024
- LLM Hosting Strategy, Options & Cost: Examples - June 30, 2024
- Application Architecture for LLM Applications: Examples - June 25, 2024
Leave a Reply