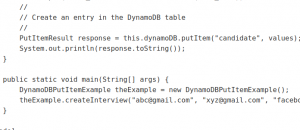
In this post, you will learn about Java code example related to creating an entry in the DynamoDB table using PutItem API. The following are some of the points considered later in this article:
- Configure Dev Environment with AWS Credentials
- Update POM.xml or Gradle file to include DynamoDB library
- Use PutItem API to create an Item in DynamoDB table
- PutItem API example with Spring Boot app
Configure Dev Environment with AWS Credentials
Make sure you have configured your dev environment with appropriate Access Key ID and secret access key. You could do that by executing the command such as following:
aws configure
If you have configured it correctly, you should be able to find your key details in credentials which can be found at the following path: ~/.aws/credentials
Update POM.xml or Gradle file to include DynamoDB library
In case, you are working with a Java Maven project, put following in the pom.xml file for including DynamoDB library.
<!-- https://mvnrepository.com/artifact/com.amazonaws/aws-java-sdk-dynamodb --> <dependency> <groupId>com.amazonaws</groupId> <artifactId>aws-java-sdk-dynamodb</artifactId> <version>1.11.289</version> </dependency>
Use PutItem API to create an Item in DynamoDB table
Before looking at the code, make sure you have created a table by using command or AWS DynamoDB console. In the following code example, an item is created in a table named as “candidate” which represents a candidate scheduled for one or more interviews.
The following is the table schema:
- recruiter_id: It is the partition key. It represents recruiter’s email address. Email address is considered the primary key used to identify a unique user.
- date_time: It is the sort key. The idea is to use date-time for range queries. Note the code which is used to convert Java date object to ISO 8601 date string.
- candidate_id: Candidate’s email address
- position: Representing role of the job opening
- job_descr: Job description
public class DynamoDBPutItemExample { private AmazonDynamoDB dynamoDB; public DynamoDBPutItemExample() { this.dynamoDB = AmazonDynamoDBClientBuilder.standard().build() } /** * Create new interview * @param recruiter_id - email address * @param candidate_id - email address * @param company * @param role */ public void createInterview(String recruiter_id, String candidate_id, String company, String role) { // // Create a map of key-value where key represents attribute name and // value represents attribute value // Map<String, AttributeValue> values = new HashMap<String, AttributeValue>(); values.put("recruiter_id", new AttributeValue(recruiter_id)); values.put("candidate_id", new AttributeValue(candidate_id)); values.put("company", new AttributeValue(company)); values.put("position", new AttributeValue(role)); // // Create ISO 8601 compliant date // Date date = new Date(); TimeZone tz = TimeZone.getTimeZone("UTC"); DateFormat df = new SimpleDateFormat("yyyy-MM-dd'T'HH:mm'Z'"); df.setTimeZone(tz); String date_time = df.format(date); values.put("date_time", new AttributeValue(date_time)); // // Create an entry in the DynamoDB table // PutItemResult response = this.dynamoDB.putItem("candidate", values); System.out.println(response.toString()); } public static void main(String[] args) { DynamoDBPutItemExample theExample = new DynamoDBPutItemExample(); theExample.createInterview("abc@gmail.com", "xyz@gmail.com", "facebook", "software engineer"); } }
DynamoDB PutItem Example with Spring Boot
In case, you are working with Spring Boot, the instantiation code could look like following:
@Component public class DynamoDBInterviewRepository implements InterviewRepository { public static final String TABLE_CANDIDATE = "candidate"; private AmazonDynamoDB dynamoDB; public DynamoDBInterviewRepository(@Autowired AmazonDynamoDB amazonDynamoDB) { this.dynamoDB = amazonDynamoDB; } @Override public void createInterview(String recruiter_id, String candidate_id, String company, String role) { Map<String, AttributeValue> values = new HashMap<String, AttributeValue>(); values.put("recruiter_id", new AttributeValue(recruiter_id)); values.put("candidate_id", new AttributeValue(candidate_id)); values.put("company", new AttributeValue(company)); values.put("position", new AttributeValue(role)); // // Create ISO 8601 compliant date // Date date = new Date(); TimeZone tz = TimeZone.getTimeZone("UTC"); DateFormat df = new SimpleDateFormat("yyyy-MM-dd'T'HH:mm'Z'"); df.setTimeZone(tz); String date_time = df.format(date); values.put("date_time", new AttributeValue(date_time)); // // Create an entry in the DynamoDB table // PutItemResult response = this.dynamoDB.putItem(TABLE_CANDIDATE, values); System.out.println(response.toString()); } }
AmazonDynamoDB instance could be created as configuration bean. The following is the sample code:
@Configuration public class AWSAppConfig { @Bean(name = "amazonDynamoDB") public AmazonDynamoDB amazonDynamoDB() { return AmazonDynamoDBClientBuilder.standard().build(); } }
Use DynamoDBMapper and PutItem API to create an Item in DynamoDB table
You can also use DynamoDBMapper and related techniques to create an entry in the table. This would help you to map a class to DynamoDB table and perform operations using appropriate APIs. The following needs to be done:
- Create a class mapping to the table in the DynamoDB; As per the code mentioned above, you will create a class, namely, Candidate. The following is the sample code:
package com.vflux.rbot.services; import com.amazonaws.services.dynamodbv2.datamodeling.DynamoDBAttribute; import com.amazonaws.services.dynamodbv2.datamodeling.DynamoDBHashKey; import com.amazonaws.services.dynamodbv2.datamodeling.DynamoDBRangeKey; import com.amazonaws.services.dynamodbv2.datamodeling.DynamoDBTable; @DynamoDBTable(tableName="candidate") public class Candidate { private String recruiterId; private String candidateId; private String company; private String role; private String jobDescr; private String dateTime; @DynamoDBHashKey(attributeName="recruiter_id") public String getRecruiterId() { return recruiterId; } public void setRecruiterId(String recruiterId) { this.recruiterId = recruiterId; } @DynamoDBAttribute(attributeName="candidate_id") public String getCandidateId() { return candidateId; } public void setCandidateId(String candidateId) { this.candidateId = candidateId; } @DynamoDBAttribute(attributeName="company") public String getCompany() { return company; } public void setCompany(String company) { this.company = company; } @DynamoDBAttribute(attributeName="role") public String getRole() { return role; } public void setRole(String role) { this.role = role; } @DynamoDBAttribute(attributeName="job_descr") public String getJobDescr() { return jobDescr; } public void setJobDescr(String jobDescr) { this.jobDescr = jobDescr; } @DynamoDBRangeKey(attributeName="date_time") public String getDateTime() { return dateTime; } public void setDateTime(String datetime) { this.dateTime = datetime; } }
- In the above code, pay attention to the usage of some of the following:
- DynamoDBTable annotation to map the table
- DynamoDBHashKey to map the partition key
- DynamoDBRangeKey to map the sort or range key
- DynamoDBAttribute to map other attributes
- Use the following code to create an entry in the table. Pay attention to usage of the class DynamoDBMapper:
public class DynamoDBPutItemExample { private DynamoDBMapper dynamoDBMapper; public DynamoDBPutItemExample() { AmazonDynamoDB dynamoDB = AmazonDynamoDBClientBuilder.standard().build() this.dynamoDBMapper = new DynamoDBMapper(dynamoDB); } /** * Create new Candidate * @param recruiter_id - email address * @param candidate_id - email address * @param company * @param role */ public void createInterview(String recruiter_id, String candidate_id, String company, String role) { // // Create a Candidate object where key represents attribute name and // value represents attribute value // Candidate candidate = new Candidate(); candidate.setRecruiterId(recruiter_id); candidate.setCandidateId(candidate_id); candidate.setCompany(company); candidate.setRole(role); // // Create ISO 8601 compliant date // Date date = new Date(); TimeZone tz = TimeZone.getTimeZone("UTC"); DateFormat df = new SimpleDateFormat("yyyy-MM-dd'T'HH:mm'Z'"); df.setTimeZone(tz); String date_time = df.format(date); candidate.setDateTime(date_time); // // Create an entry in the DynamoDB table // this.dynamoDBMapper.save(candidiate); } public static void main(String[] args) { DynamoDBPutItemExample theExample = new DynamoDBPutItemExample(); theExample.createInterview("abc@gmail.com", "xyz@gmail.com", "facebook", "software engineer"); } }
Further Reading / References
- DynamoDB PutItem
- AmazonDynamoDB API
- AmaazonDynamoDBClient
- AWS Temporary Credentials using Java & Spring Boot
Summary
In this post, you learned about using DynamoDB PutItem Java API to create an entry in the table.
Did you find this article useful? Do you have any questions or suggestions about this article in relation to using DynamoDB PutItem Java API for creating an entry in DynamoDB table? Leave a comment and ask your questions and I shall do my best to address your queries.
- Completion Model vs Chat Model: Python Examples - June 30, 2024
- LLM Hosting Strategy, Options & Cost: Examples - June 30, 2024
- Application Architecture for LLM Applications: Examples - June 25, 2024
Leave a Reply