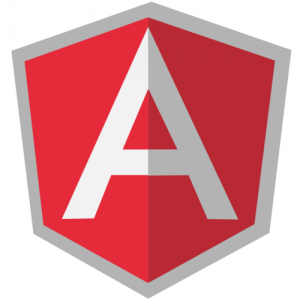
This article demonstrates how one could create custom services in AngularJS and use them within controllers or other services using dependency injection. In this article, the service demonstrated is Calculator service with just one API named as “calculate”. The API takes two numbers and operation type and calculate the result appropriately. The demo could be found on this page, http://hello-angularjs.appspot.com/angularjs-create-custom-services
Following are three key steps in creating using custom services:
- Create the code for service (take a look at example below showing Calculator class with a constructor, Calculator()
- Register the recipe or method to inject the service. Injector service uses this to inject the service
- Inject the new custom service in the Controller or in other services
Step 1: Create/Define the Service
The code below represents how to create a custom service. The Calculator class is defined with a constructor and an API namely, calculate. Other methods such as add, subtract etc are private methods of this class. The method “calculate” is invoked on the “calculator” service object that is injected into the controller component (Look at step 3).
function Calculator() {
this.calculate = function( no1, no2, optype ) {
var result = 0;
switch( optype ) {
case '+':
result = add( no1, no2);
break;
case '-':
result = subtract( no1, no2);
break;
case '*':
result = multiply( no1, no2);
break;
case '/':
result = divide( no1, no2);
break;
default:
}
return result;
}
var add = function( no1, no2 ) {
return no1 + no2;
}
var subtract = function( no1, no2 ) {
return no1 - no2;
}
var multiply = function( no1, no2 ) {
return no1 * no2;
}
var divide = function( no1, no2 ) {
return no1 / no2;
}
}
Step 2: Defining Factory Method for Injecting Calculator Service
In following code, the Calculator service is created using factory method. The injector service invokes the factory method on the app, (calcApp), and obtains an instance of Calculator service. The “factory” method constructs a new service using a function with zero (as shown below) or more arguments (dependencies on other services). The return value of this function is the service instance.
It has been found many a times, that one tries and inject $scope object in function() such as function($scope) with the thought that the parameters of $scope object could be used within the service by reference. However, Angular throws error with that. With factory method, only services can be injected. And, $scope object can not be considered as service. The best practice is to pass the parameters in the method as shown in the API, calculate.
calcApp.factory('calculator', function() {
return new Calculator();
});
There are other ways of creating a service, the details of which could be found on this page (https://docs.angularjs.org/guide/providers).
Step 3: Injecting Calculator Service and Invoking the Method
Following code represents how one could inject a custom service. In the code below, “calculator” service is injected by Angular injector service. Also, pay attention to the method invocation, calculator.calculate.
var calcApp = angular.module( "calcApp", [] );
calcApp.controller( "CalculatorCtrl", [ '$scope','calculator', function($scope, calculator ) {
$scope.types = [
{ name : 'Add', value : '+' },
{ name : 'Subtract', value : '-' },
{ name : 'Multiply', value : '*' },
{ name : 'Divide', value : '/' },
];
$scope.type = $scope.types[0];
$scope.calculate = function() {
$scope.result = calculator.calculate( $scope.no1, $scope.no2, $scope.type.value );
}
}]);
…
[adsenseyu1]
- Completion Model vs Chat Model: Python Examples - June 30, 2024
- LLM Hosting Strategy, Options & Cost: Examples - June 30, 2024
- Application Architecture for LLM Applications: Examples - June 25, 2024
Leave a Reply