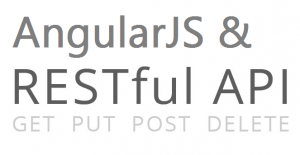
Following are two different use-case scenarios that has been explained in this article:
- Save/Persist new data objects
- Update existing data objects
The code snippets shall cover both AngularJS code and Spring MVC take away codes for your ease of getting started as quickly as possible.
For one to work with $resource service, following needs to be done prior to hitting actual code:
Include angular-resource.js file. You could use Google Hosted Libraries for this purpose. Following code works for the most recent version of angularJS.
<script src="//ajax.googleapis.com/ajax/libs/angularjs/1.2.19/angular-resource.js"></script>
Include ngResource module and, inject $resource service while creating controller object as shown in the code below:
var helloApp = angular.module("helloApp", [ 'ngResource' ]);
helloApp.controller("HttpController", [ '$scope', '$resource', function($scope, $resource) {
//
// The actual code goes here...
//
} ]);
Save/Persist New Object
Following code demonstrates the end user adding the data through various forms fields, which then sends the data to the server at REST URL “/user/new” using POST method. Also look at the corresponding Spring MVC method.
AngularJS code
var helloApp = angular.module("helloApp", [ 'ngResource' ]);
helloApp.controller("HttpController", [ '$scope', '$resource', function($scope, $resource) {
$scope.users = [
{ 'firstname':'Ajitesh',
'lastname':'Shukla',
'address':'Hyderbad',
'email':'ajitesh101@gmail.com'},
{ 'firstname':'Sumit',
'lastname':'Jha',
'address':'Muzaffarpur',
'email':'sumitjha2011@yahoo.com'},
];
$scope.saveUser = function(){
// Create a resource class object
//
var User = $resource('/user/new');
// Call action method (save) on the class
//
User.save({firstname:$scope.firstname,lastname:$scope.lastname,address:$scope.address,email:$scope.email}, function(response){
$scope.message = response.message;
});
}
} ]);
Spring MVC code
Pay attention to the fact that a User object needs to be created matching the JSON data. Also, make sure that Jackson library is included in the path for the following code to work. This is one of the MOST IMPORTANT STEP. I recommend to read this article on how to fix 415 Unsupported Mediatype error which one may hit if one forgets to implement this step. This is one error that may haunt most of the newbies.
// Create a new user
//
@RequestMapping(value = "/user/new", method = RequestMethod.POST)
public @ResponseBody String saveUserRestful( @RequestBody User user ) {
//
// Code processing the input parameters
//
String response = "{\"message\":\"Post With ngResource: The user firstname: " + user.getFirstname() + ", lastname: " + user.getLastname() + ", address: " + user.getAddress() + ", email: " + user.getEmail()+"\"}";
return response;
}
Update Existing Data Objects
Following code demonstrates the end user updating the data through various forms fields, which then sends the data to the server at REST URL “/user/{id}” using POST method. Also look at the corresponding Spring MVC method.
AngularJS code
var helloApp = angular.module("helloApp", [ 'ngResource' ]);
helloApp.controller("HttpController", [ '$scope', '$resource', function($scope, $resource) {
$scope.users = [
{ 'firstname':'Ajitesh',
'lastname':'Shukla',
'address':'Hyderbad',
'email':'ajitesh101@gmail.com'},
{ 'firstname':'Sumit',
'lastname':'Jha',
'address':'Muzaffarpur',
'email':'sumitjha2011@yahoo.com'},
];
$scope.updateUser = function(){
// Create a resource class object
//
var User = $resource('/user/:userId', {userId:'@id'});
// Create an instance of User resource
var user = User.get({userId:25});
// Update the new values entered in the form fields
//
user.id = 25;
user.firstname = $scope.firstname;
user.lastname = $scope.lastname;
user.address = $scope.address;
user.email = $scope.email;
// Call '$' prefixed action menthod to post ("save" )
//
user.$save(function(response){
$scope.message = response.message;
});
// Push the new objects in the $scope.users
//
$scope.users.push({ 'firstname':$scope.firstname, 'lastname': $scope.lastname, 'address':$scope.address, 'email':$scope.email });
$scope.firstname='';
$scope.lastname='';
$scope.address='';
$scope.email='';
}
} ]);
Spring MVC code
Pay attention to some of the following facts:
– Usage of PathVariable
– A User object is created matching the JSON data.
– Make sure that Jackson library is included in the path for the following code to work.
// Update the user
//
@RequestMapping(value = "/user/{id}", method = RequestMethod.POST)
public @ResponseBody String updateUserProfile( @PathVariable("id") long userId, @RequestBody User user ) {
//
// Code processing the input parameters
//
String response = "{\"message\":\"Post With ngResource - id: " + String.valueOf( userId ) + ",firstname: " + user.getFirstname() + ", lastname: " + user.getLastname() + ", address: " + user.getAddress() + ", email: " + user.getEmail() +"\"}";
return response;
}
…
[adsenseyu1]
- Completion Model vs Chat Model: Python Examples - June 30, 2024
- LLM Hosting Strategy, Options & Cost: Examples - June 30, 2024
- Application Architecture for LLM Applications: Examples - June 25, 2024
Hi Ajitesh … i’m developing login module using Angularjs with MySql database and using spring MVC….already developed Ui and java backend coding … but i get stuck in calling Rest Service via Angularjs controller using json object ??how can i proceed??
Arun,
Take a look at the http://www.angularcode.com/user-authentication-using-angularjs-php-mysql/