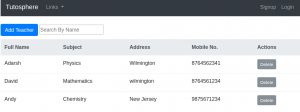
This article represents concepts and code samples in relation to filtering or searching table by one or more column data when working with Angular (Angular 2.*, Angular 4.* Angular 5.*). One can achieve the search/filter table by column functionality by creating custom pipes. Although Angular recommends it by handling the logic in services, one can always create custom pipes as shown in this article.
The following topic is discussed later in this article:
- No Out-of-box support for Filter or OrderBy in Angular
- How to create search filter for filtering table by column
The details on creating custom pipes can be read from this page, Angular Pipes.
No Out-of-box support for Filter or OrderBy in Angular
Unlike AngularJS, Angular 2.* and later versions such as Angular 4.* and Angular 5.* have not provided out-of-box support for search and orderby filter. In AngularJS, for searching or filtering, the filter was used and for sorting, orderBy was used.
Angular newer released decided not to support these filters out-of-box. Filtering and sorting led to a severe impact on the user experience for even moderate-sized lists when Angular calls these pipe methods many times per second. That often led many to say that AngularJS has poor performance.
As a best practice, the Angular team recommends handling filter and orderBy by handling logic within services rather than pipes. Read further on this page, Angular Pipes
How to Create Search Filter for filtering Table by Column
In the code below, a table consisting of teachers’ information is searched by teacher name. The table is filtered by column “Full Name”. In screenshot given below, based on text entered in “Search By Name” field, matching rows are displayed.
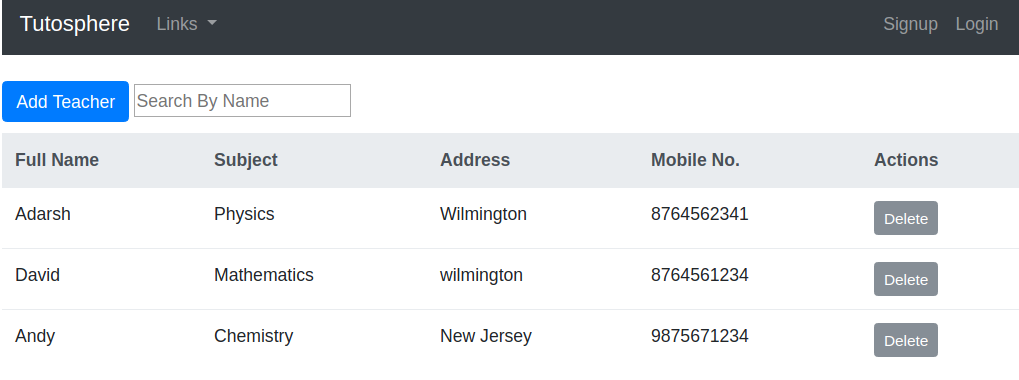
Figure 1. Angular – Search Table by Column Filter
The following steps can be used to create a search filter for filtering table by specific column:
- Create a custom pipe
- Include pipe in the module
- Modify Component and Related View for Search Field
- Apply pipe in component’s view to filter table
Create a Custom Pipe
Create a custom pipe using following code. Notice some of the following:
- The first argument represents data (teachers array) on which filter is applied
- The second argument represents the additional parameter/argument which is passed to the filter.
Save the custom pipe in the file such as search-by-name.pipe.ts.
import { Pipe, PipeTransform } from '@angular/core'; import { Teacher } from './teacher'; @Pipe({ name: 'searchByName' }) export class SearchByNamePipe implements PipeTransform { transform(teachers: Teacher[], searchText: string) { return teachers.filter(teacher => teacher.name.indexOf(searchText) !== -1); } }
Include Custom Pipe in the Module
The code below represents the import of custom pipe, SearchByNamePipe.
import { BrowserModule } from '@angular/platform-browser'; import { NgModule } from '@angular/core'; import { FormsModule } from '@angular/forms'; import { AppComponent } from './app.component'; import { SearchByNamePipe } from './search-by-name.pipe'; @NgModule({ declarations: [ AppComponent, SearchByNamePipe ], imports: [ BrowserModule, FormsModule ], providers: [], bootstrap: [AppComponent] }) export class AppModule { }Modify Component and Related View for Search Field
- Create a field, searchText in the component. Here is the how the full code of app.component.ts would look like:
import { Component } from '@angular/core'; import {Teacher} from './teacher'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent { title = 'Tutosphere'; searchText = ''; id = 1; teacher = new Teacher(0, '', '', '', 0); TEACHERS = [ new Teacher(this.id, 'Adarsh', 'Physics', 'Wilmington', 8764562341) ]; addTeacher() { this.id += 1; const teacherEntry = new Teacher(this.id, this.teacher.name, this.teacher.subject, this.teacher.address, this.teacher.mobile); this.TEACHERS.push(teacherEntry); this.resetTeacher(); } deleteTeacher(id: number) { for (let i = this.TEACHERS.length - 1; i >= 0; i--) { if (this.TEACHERS[i].id === id) { this.TEACHERS.splice(i, 1); } } } resetTeacher() { this.teacher.name = ''; this.teacher.subject = ''; this.teacher.address = ''; this.teacher.mobile = 0; } }
- Create following entry in app.component.html.
<input type="text" id="searchTextId" [(ngModel)]="searchText" name="searchText" placeholder="Search By Name">
For detailed code on app.component.ts and app.component.html, take a look at this page, Add/Remove Table Row.
Apply Custom Pipe in Component’s view to filter Table by Column
The code below represents how the pipe is applied to TEACHERS array.
<tr *ngFor="let teacher of (TEACHERS | searchByName: searchText)"> <td>{{teacher.name}}</td> <td>{{teacher.subject}}</td> <td>{{teacher.address}}</td> <td>{{teacher.mobile}}</td> <td><button type="button" class="btn btn-secondary btn-sm" (click)="deleteTeacher(teacher.id)">Delete</button></td> </tr> Latest posts by Ajitesh Kumar (see all)
- Mathematics Topics for Machine Learning Beginners - July 6, 2025
- Questions to Ask When Thinking Like a Product Leader - July 3, 2025
- Three Approaches to Creating AI Agents: Code Examples - June 27, 2025
Great post. helped me a ton! You do have a typo in your search-by-name.pipe.ts:
“return teachers.filter(teacher =>”
should be “return teachers.filter(teacher =>”