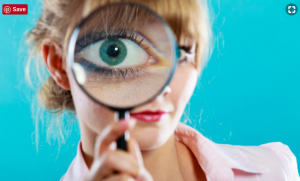
Observable is one of the most important aspect of reactive programming paradigm which represents the data streams such as variables, user inputs, properties, caches, data structures, server responses etc.. These data streams (or Observable) are listened/observed (you may also read subscribe) in asynchronous manner and reacted appropriately as per the application requirement. Observable emits the following three class of outputs which can be processed asynchronously:
- Value
- Error
- Completion status
Different functions can be used to process above mentioned different types of outputs emitted by observable. In this post, we will see the usage of subscribe method to process the value emitted by Observable.
On the lighter note, the following comic strip emphasizes the aspect of observing (the values) by way of subscribing to the observable (streams) outcome, and, reacting appropriately. This is key to the reactive programming paradigm.
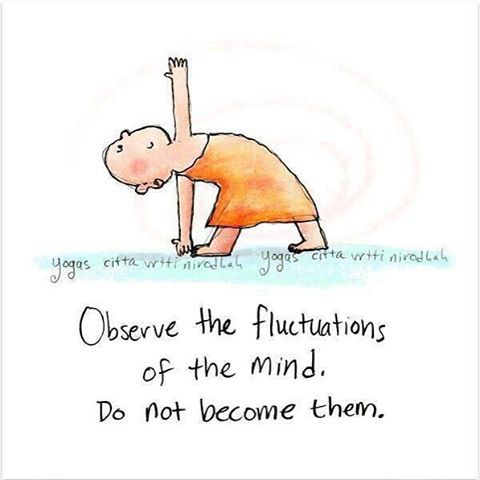
Figure 1. Observe and react appropriately
In this post, you will learn about some of the following:
- Http Get API Observable code example (Angular 4, Angular 2)
- HttpClient Get API Observable code example (Angular 5)
- Sample interview questions
Http Get API Observable code example (Angular 4, Angular 2)
The following code represents the usage of Observable with Http Get API. Note that Http service is deprecated from Angular 5.* onwards. Those working with Angular 4 and Angular 2 versions would find the below code as useful.
import {Http, Headers, RequestOptions} from '@angular/http'; import {Injectable} from '@angular/core'; import {Rx} from './rx'; import {Observable} from 'rxjs/Observable'; import {Cookie} from 'ng2-cookies'; @Injectable() export class RxService { private rxUrl = 'http://localhost:8080/rx'; constructor(private http: Http) { } getRx(): Observable<Rx[]> { let headers = new Headers({ 'Content-type': 'application/json', 'Authorization': 'Bearer ' + Cookie.get('access_token') }); return this.http.get(this.rxUrl, {headers: headers}) .map(res => res.json()) .catch(err => { return Observable.throw(err.json().error || 'Server error'); }); } }
The following code represents the usage of subscribe method to observe the value emitted by the Observable as a result of invoking Http Get API. You may want to recall that the Observable is different than Promise is in the way that Promise object is processed just once whereas the Observable value is listened/observed at all times and processed appropriately everytime the value gets emitted.
Make a note of subscribe method on this.rxService.getRx.
import {RxService} from './rx.service'; import {Rx} from './rx'; import {Component, OnInit} from '@angular/core'; import {Router} from '@angular/router'; @Component({ templateUrl: './rx-list.component.html' }) export class RxListComponent implements OnInit { rxlist: Rx[]; errorMessage: string; constructor(private rxService: RxService, private router: Router) { this.errorMessage = ''; } ngOnInit(): void { this.getRx(); } getRx() { this.rxService.getRx() .subscribe(rxlist => { this.rxlist = rxlist; if (this.rxlist.length === 0) { this.errorMessage = 'There are no prescriptions you have created so far!'; } }, error => { this.router.navigateByUrl('/auth/login'); console.error('An error occurred in retrieving rx list, navigating to login: ', error); }); } }
HttpClient Get API Observable code example (Angular 5)
HttpClient is introduced in later version of Angular 4 (Angular 4.3) and then finalized in Angular 5.*. Angular recommends usage of HttpClient from current version onwards (Angular 5.*). The information on HttpClient APIs can be found from Angular HttpClient API documentation page.
Make a note of some of the following points:
- Usage of HttpHeaders in place of Headers (as with Http API)
- HttpResponse<object> is returned as a response which needs to be processed.
- HttpClient is imported from @angular/common/http library whereas Http is imported from @angular/http.
import {Injectable} from '@angular/core'; import {Rx} from './rx'; import {Observable} from 'rxjs/Observable'; import {Cookie} from 'ng2-cookies'; import {HttpClient, HttpHeaders, HttpResponse} from "@angular/common/http"; @Injectable() export class RxService { private rxUrl = 'http://localhost:8080/rx'; constructor(private httpClient: HttpClient) { } getRx(): Observable<Rx[]> { return this.httpClient.get(this.rxUrl, {observe: 'response', headers: new HttpHeaders().set('Authorization', 'Bearer ' + Cookie.get('access_token'))}) .map(this.extractData) .catch(err => { return Observable.throw(err.json().error || 'Server error'); }); } extractData(res: HttpResponse<Object>) { let array = new Array(); let key, count = 0; for(key in res.body) { array.push(res.body[count++]); } return array; } }
The code for subscription would remain same as what is show in above section related with Http Get API.
Sample Interview Questions
- What is Observable?
- How is Observable different from Promise?
Further Reading/References
- Introduction to Reactive Programming you’ve been missing – I Swear!
- Angular HttpClient API Documentation
- Angular APIs
Summary
In this post, you learned about some of the following:
- How to use Observable with Http Get API; This would be useful for Angular 2 and Angular 4 apps.
- How to use Observable with HttpClient Get API; This would be useful for Angular 5.* (and later versions) apps.
- Sample interview questions
Did you find this article useful? Do you have any questions about this article or understanding usage of Observable with Angular Http Get or HttpClient Get APIs? Leave a comment and ask your questions and I shall do my best to address your queries.
- Completion Model vs Chat Model: Python Examples - June 30, 2024
- LLM Hosting Strategy, Options & Cost: Examples - June 30, 2024
- Application Architecture for LLM Applications: Examples - June 25, 2024
Leave a Reply