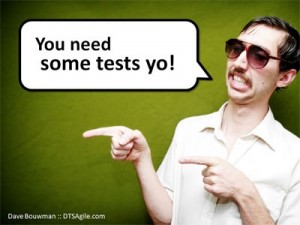
The article presents an example of unit tests which tests both happy path and exception scenario.
Business Requirement
- User trying to open an account must be validated against the business rules related with users’ registration.
- Following are different business rules:
- User must provide a valid email address
- User must provide his first name
- User’s age must be 18 and above
Class Design
To demonstrate the unit tests with happy and exception scenarios, following classes have been shown below:
- SignupValidation: Consists of code validating business rules
- SignupException: Custom exception representing Signup business rules failure
- EmailValidator (Get the code from this page).
- SignupValidationTests: Unit tests for testing signup validation code
Class SignupValidation
The class consists of the three validateXXX methods to validate three different business rules mentioned above. The need is to test these three validation methods in the unit tests.
public class SignupValidation {
private EmailValidator emailValidator;
public SignupValidation() {
emailValidator = new EmailValidator();
}
public boolean validate( User u ) throws SignupException{
validateEmailAddress( u.getEmailAddress() );
validateAge( u.getAge() );
validateFirstName( u.getFirstName() );
return true;
}
public boolean validateFirstName(String firstName) throws SignupException {
if( firstName.trim().length() == 0 ) {
throw new SignupException( "Firstname can not be empty" );
}
return true;
}
public boolean validateEmailAddress( String email ) throws SignupException {
boolean isValid = emailValidator.validate( email );
if( !isValid ) {
throw new SignupException( "Invalid email address" );
}
return isValid;
}
public boolean validateAge( int age ) throws SignupException {
if( age < 18 ) {
throw new SignupException( "Age must be 18 and above" );
}
return true;
}
}
Class SignupValidationTest
The unit tests consist of methods to test both, happy path and the exception scenarios. Take a look at following for details:
- validateValidFirstName: This tests the happy path when users enter the valid first name. In case, there is a change in business rule due to which exception thrown is caught, the unit test fails. See the code, “fail( e.getMessage() )“
- validateInvalidFirstName: This tests the exception scenario when users enter invalid first name. As a result, the validateFirstName method in SignupValidation class throws exception. Here, in unit test, the exception thrown is matched with expected exception class. See the code, “@Test( expected = SignupException.class)“
public class SignupValidationTest {
private SignupValidation sv;
private User u;
@Before
public void setUp() throws Exception {
sv = new SignupValidation();
}
@After
public void tearDown() throws Exception {
sv = null;
}
@Test
public void testPassedValidation() {
User u = new User();
u.setEmailAddress( "xyz@gmail.com" );
u.setFirstName( "Chris" );
u.setAge( 20 );
try {
sv.validate( u );
} catch (SignupException e) {
fail( e.getMessage() );
}
}
@Test (expected = SignupException.class)
public void testFailedValidation() throws SignupException {
User u = new User();
u.setEmailAddress( "xyz@gmail.com" );
u.setFirstName( "Chris" );
u.setAge( 16 );
sv.validate( u );
}
@Test
public void validateValidFirstName() {
try {
sv.validateFirstName( "Chris" );
} catch (SignupException e) {
fail( e.getMessage() );
}
}
@Test (expected = SignupException.class)
public void validateInvalidFirstName() throws SignupException {
sv.validateFirstName( "" );
sv.validateFirstName( " " );
}
@Test
public void validateValidEmailAddress() {
try {
sv.validateEmailAddress( "xyz@gmail.com" );
} catch (SignupException e) {
fail( e.getMessage() );
}
}
@Test (expected = SignupException.class)
public void validateInvalidEmailAddress() throws SignupException {
sv.validateEmailAddress( "xyz@gmail." );
sv.validateEmailAddress( "xyzgmail.com" );
sv.validateEmailAddress( "gmail.com" );
sv.validateEmailAddress( "gmail" );
sv.validateEmailAddress( "@gmail.com" );
}
@Test
public void validateAgeGreaterThan17() {
try {
sv.validateAge( 18 );
} catch (SignupException e) {
fail( e.getMessage() );
}
}
@Test (expected = SignupException.class)
public void validateAgeLessThan18() throws SignupException {
sv.validateAge( 17 );
}
}
Class SignupException
public class SignupException extends Exception {
public SignupException( String message ) {
super( message );
}
}
[adsenseyu1]
Latest posts by Ajitesh Kumar (see all)
- Mathematics Topics for Machine Learning Beginners - July 6, 2025
- Questions to Ask When Thinking Like a Product Leader - July 3, 2025
- Three Approaches to Creating AI Agents: Code Examples - June 27, 2025
I found it very helpful. However the differences are not too understandable for me