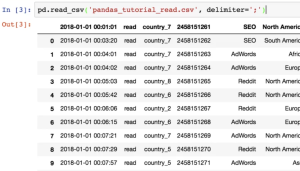
Converting CSV files to DataFrames is a common task in data analysis. In this blog, we’ll explore a Python code example using the Pandas library to efficiently convert CSV files to DataFrames. This approach offers flexibility, speed, and convenience, making it a valuable technique for handling large datasets.
Read CSV into Pandas Dataframe
The following is the code which can be used to read the CSV file from local drive:
import pandas as pd
# File path of the CSV file
csv_file = 'path/to/your/file.csv'
# Read CSV file into a DataFrame
df = pd.read_csv(csv_file)
# Perform operations on the DataFrame
# ...
# Display the DataFrame
print(df.head())
In case, you want to read CSV file from the URL, the following will be the code. As a matter of fact, nothing changes except for the fact that you pass the URL to read_csv function.
import pandas as pd
# URL of the CSV file
csv_url = 'https://example.com/data.csv'
# Read CSV file from the URL into a DataFrame
df = pd.read_csv(csv_url)
# Perform operations on the DataFrame
# ...
# Display the DataFrame
print(df.head())
The following are some common errors one can face while executing the above code:
- File Not Found Error: This error occurs when the specified file path or URL is incorrect or the file does not exist at the given location. Double-check the file path or URL to ensure it is accurate and that the file exists.
- Permission Denied Error: If you do not have the necessary permissions to access the file, you may encounter a permission denied error. Make sure you have the appropriate read permissions for the file or directory.
- Parsing Error: If the CSV file has formatting issues or contains unexpected characters, it may result in a parsing error. Common examples include mismatched quotes, delimiters within quoted fields, or encoding-related problems. Verify the file’s formatting and encoding, and consider using additional parameters like delimiter, quotechar or encooding in the read_csv() function to handle specific scenarios.
- Memory Error: Reading a large CSV file into a DataFrame consumes memory. If the file size exceeds the available memory, a memory error can occur. In such cases, consider using chunked reading or other memory optimization techniques, like specifying the chunksize parameter in the read_csv() function.
- Module Dependency Errors: The code requires the Pandas library to be installed. If you encounter a “module not found” error, ensure that Pandas is installed in your Python environment. You can install it using pip install pandas command
Why read CSV file into Dataframe?
The following can be few use cases where you would be required to read CSV file into Pandas Dataframe for further data processing.
- Data Analysis: When analyzing data stored in CSV files, converting them to DataFrames enables easy exploration, manipulation, and visualization of the data. It facilitates tasks like filtering, aggregating, and transforming data for gaining insights.
- Building Machine Learning Models: CSV files are commonly used to store training datasets. By converting CSV files to DataFrames, you can preprocess the data, perform feature engineering, and prepare it for machine learning algorithms.
- Data Integration: Many real-world scenarios involve integrating data from multiple sources. Converting CSV files to DataFrames allows you to merge, join, or concatenate datasets easily, providing a unified view of the data.
- Data Cleaning and Transformation: CSV files often contain missing values, inconsistent formatting, or outliers. With DataFrames, you can leverage Pandas’ extensive functionalities to clean and transform the data, ensuring its quality and usability.
- Completion Model vs Chat Model: Python Examples - June 30, 2024
- LLM Hosting Strategy, Options & Cost: Examples - June 30, 2024
- Application Architecture for LLM Applications: Examples - June 25, 2024
Leave a Reply