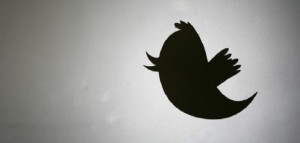
This article represents code samples which could be used to retrieve users tweets for one or more Twitter users, using Twitter HBC Java client. Please feel free to comment/suggest if I missed to mention one or more important points. Also, sorry for the typos.
Following are the key points described later in this article:
- Key Steps in Retrieving the Users Tweets
- Code Sample – Get User Tweets
Key Steps in Retrieving the Users Tweets
Following are key steps required to be taken to retrieve users tweets:
- Get consumer key and access tokens details
- Determine userId for users for whom you want to get the tweets as they appear. For test purpose, you could get the UserIds from GetTwitterId.com
- Use the above details in the code such as below to retrieve the data.
Code Sample – Get User Tweets
Pay attention to some of the following facts:
- You are required to get details such as following to get going. These details could be obtained from twitter developers website.
- Consumer Key
- Consumer Secret
- Access Token
- Access Token Secret
- Get userId for users for whom you want to get the tweets as they appear. For test purpose, you could get the UserIds from GetTwitterId.com
- The class given below consists of a main method which is used to invoke
- StatusesFilterEndpoint class is used to get the user tweets. It has this API “public StatusesFilterEndpoint followings(List<Long> userIds)” which makes it happen.
- A ClientBuilder is used to create a Client object using following information:
- OAuth object created using consumer and access token details
- Host information
- Endpoint information
- Queue object which would be used to deliver message to this program
public class FilterStreamExample {
public static final String CONSUMER_KEY = "Your_consumer_key";
public static final String CONSUMER_SECRET = "Your_consumer_secret";
public static final String ACCESS_TOKEN = "Your_access_token";
public static final String ACCESS_TOKEN_SECRET = "Your_access_token_secret";
public static void process(String consumerKey, String consumerSecret,
String token, String secret) throws InterruptedException {
//
// Create queue which would be used to get message
//
BlockingQueue queue = new LinkedBlockingQueue(10000);
//
// Create an endpoint of type StatusesFilterEndpoint; It has APIs to retrieve
// users tweets or treats related with mention or hashtags
//
StatusesFilterEndpoint endpoint = new StatusesFilterEndpoint();
//
// Add one or more users to follow the tweets;
//
endpoint.followings(Lists.newArrayList( 136976940L));
//
// Create OAuth object using consumer keys/secret and access token/secret
//
Authentication auth = new OAuth1(consumerKey, consumerSecret, token,
secret);
//
// Create a new BasicClient. By default gzip is enabled.
//
Client client = new ClientBuilder().hosts(Constants.STREAM_HOST)
.endpoint(endpoint).authentication(auth)
.processor(new StringDelimitedProcessor(queue)).build();
//
// Establish a connection
//
client.connect();
//
// Code below would extract message as it appears on Twitter
// Do whatever needs to be done with messages; In the code below,
// the message is printed; In real world, the message could be stored
// in Hadoop storage
//
for (int msgRead = 0; msgRead < 1000; msgRead++) {
String msg = queue.take();
System.out.println(msg);
}
client.stop();
}
public static void main(String[] args) {
try {
FilterStreamExample.process(CONSUMER_KEY, CONSUMER_SECRET,
ACCESS_TOKEN, ACCESS_TOKEN_SECRET);
} catch (InterruptedException e) {
System.out.println(e);
}
}
}
Latest posts by Ajitesh Kumar (see all)
- Three Approaches to Creating AI Agents: Code Examples - June 27, 2025
- What is Embodied AI? Explained with Examples - May 11, 2025
- Retrieval Augmented Generation (RAG) & LLM: Examples - February 15, 2025
I found it very helpful. However the differences are not too understandable for me