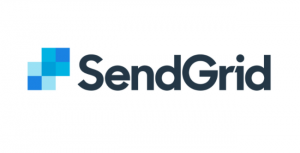
In this post, you will learn about integrating your Spring Boot & Java app with SendGrid Web API. The following are some of the points covered:
- Create & Configure/Load SendGrid API Key
- Configure SendGrid Maven entry in POM.xml
- SendGrid EmailService custom implementation
- Invoke/Test Email Service
Create & Configure/Load SendGrid API Key
- Create an account with SendGrid.com
- Go to SendGrid Setup Guide using Web API page. Create an API key.
- Execute the following script in your development environment.
echo "export SENDGRID_API_KEY='Paste_Your_API_Key'" > sendgrid.env echo "sendgrid.env" >> .gitignore source ./sendgrid.env
- Alternatively, you could also configure the SendGrid API key in your application.properties file and read the key details as part of loading configuration beans. The following is the sample code:
import org.springframework.beans.factory.annotation.Value; import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration; import org.springframework.context.annotation.PropertySource; import com.sendgrid.SendGrid; @Configuration @PropertySource("classpath:application.properties") public class SendGridConfig { @Value("${sendgrid.api.key}") String sendGridAPIKey; return new SendGrid(sendGridAPIKey); } }
In the above code sample, the SendGrid API Key is read from application.properties file. The following is the content of application.properties file.
sendgrid.api.key = ABCcvbdTYDRS_ksjdhIUYICS.-836jksjsdhYTkPt12hdgsT-whdk ahdjfpT3shdBCVNS
Make sure that you put API key and not API Key Id. Or, else, you would get the exception such as SendGrid API Key not working. “The provided authorization grant is invalid, expired or revoked”.
Configure SendGrid Maven Entry in POM.xml
Get the latest SendGrid Java dependency from Maven SendGrid Java page.
<!-- https://mvnrepository.com/artifact/com.sendgrid/sendgrid-java --> <dependency> <groupId>com.sendgrid</groupId> <artifactId>sendgrid-java</artifactId> <version>4.1.2</version> </dependency>
SendGrid EmailService Custom Implementation
The following represents the implementation for SendGrid APIs. Pay attention to some of the following:
- SendGrid instance is auto-wired at the time of construction.
- Content type “text/plain” is used for sending email in plain text format.
- Content type “text/html” is used for sending email in HTML format.
@Service public class SendGridEmailService implements EmailService { private SendGrid sendGridClient; @Autowired public SendGridEmailService(SendGrid sendGridClient) { this.sendGridClient = sendGridClient; } @Override public void sendText(String from, String to, String subject, String body) { Response response = sendEmail(from, to, subject, new Content("text/plain", body)); System.out.println("Status Code: " + response.getStatusCode() + ", Body: " + response.getBody() + ", Headers: " + response.getHeaders()); } @Override public void sendHTML(String from, String to, String subject, String body) { Response response = sendEmail(from, to, subject, new Content("text/html", body)); System.out.println("Status Code: " + response.getStatusCode() + ", Body: " + response.getBody() + ", Headers: " + response.getHeaders()); } private Response sendEmail(String from, String to, String subject, Content content) { Mail mail = new Mail(new Email(from), subject, new Email(to), content); mail.setReplyTo(new Email("abc@gmail.com")); Request request = new Request(); Response response = null; try { request.setMethod(Method.POST); request.setEndpoint("mail/send"); request.setBody(mail.build()); this.sendGridClient.api(request); } catch (IOException ex) { System.out.println(ex.getMessage()); } return response; } }
The above class implements the following custom Email Service interface:
public interface EmailService { void sendText(String from, String to, String subject, String body); void sendHTML(String from, String to, String subject, String body); }
Invoke/Test SendGrid Email Service
The following is the sample Spring Boot code which can be used to invoke the custom SendGrid email implementation created in above example.
@SpringBootApplication public class RecruiterbotApplication implements CommandLineRunner { @Autowired EmailService sendGridEmailService; public static void main(String[] args) { SpringApplication app = new SpringApplication(RecruiterbotApplication.class); app.run(args); } @Override public void run(String... arg0) throws IOException, URISyntaxException { this.sendGridEmailService.sendHTML("abc@gmail.com", "efg@gmail.com", "Hello World", "Hello, <strong>how are you doing?</strong>"); } }
Further Reading / References
Summary
In this post, you learned about how to integrate your Spring Boot application using SendGrid Java Web APIs.
Did you find this article useful? Do you have any questions or suggestions about this article? Leave a comment and ask your questions and I shall do my best to address your queries.
- Mathematics Topics for Machine Learning Beginners - July 6, 2025
- Questions to Ask When Thinking Like a Product Leader - July 3, 2025
- Three Approaches to Creating AI Agents: Code Examples - June 27, 2025
I found it very helpful. However the differences are not too understandable for me