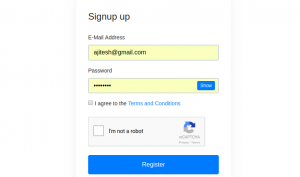
In this post, you will learn about how to implement Google Recaptcha with Angular and Spring/Java App.
- Get Recaptcha code (Key) from Google
- Angular – Process the Recaptcha code in Login/Sign up Component
- Spring/Java – RecaptchaVerifier utility class
- Spring/Java – Verify the Recaptcha Response in Server Side Code
Get Recaptcha Code (Secret Keys) from Google
Go to Google Recaptcha website and get the code for your website. The Google recaptcha code looks like following:
<div class="g-recaptcha" ng-model="g-recaptcha-response" data-sitekey="6YbGpACUBBBBBJ9pa2Km3vYeVCBV8UHYGic-dbGD"></div>
Place the code in appropriate place in login, signup pages. In the login form, it would with code such as following:
<div class="form-group no-margin"> <div class="g-recaptcha" ng-model="g-recaptcha-response" data-sitekey="6YbGpACUBBBBBJ9pa2Km3vYeVCBV8UHYGic-dbGD"></div> <button type="submit" class="btn btn-primary btn-block">Login</button> </div>
The Signup form with Google Recaptcha would look like following. The working example can be found on this signup page.
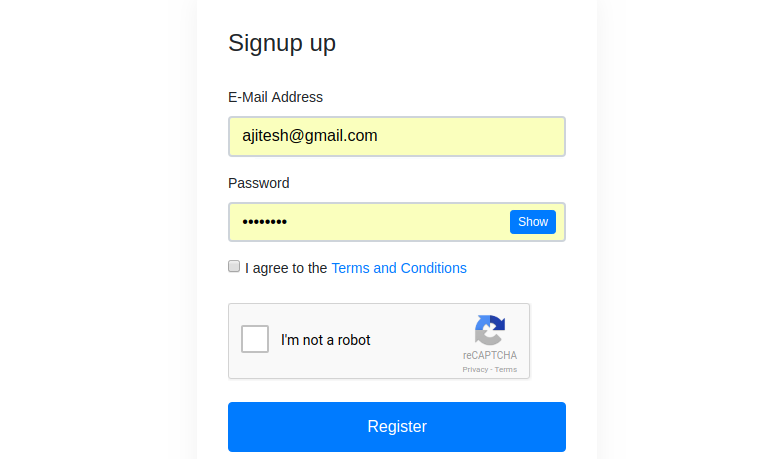
Figure 1. Google Recaptcha with Angular and Spring App
Angular – Process the Recaptcha Code in Login/Sign up Component
Process the code in Login/Sign up component such that unless the user has clicked on Google Recaptcha, they should not be allowed to proceed further. Pay attention to the code such as grecaptcha.getResponse() and the check related to the length of response.
declare var grecaptcha: any; @Component({ selector: 'app-singup', templateUrl: './signup.component.html', styleUrls: ['./signup.component.css'] }) export class SignupComponent implements OnInit { errormsg: string; constructor() { } ngOnInit() { } onSubmit() { const response = grecaptcha.getResponse(); if (response.length === 0) { this.errormsg = 'Recaptcha not verified. Please try again!'; return; } // // Rest of the code goes here // } }
Spring/Java RecaptchaVerifier Utility Class
Create a RecaptchaVerifier utility class for verifying recaptcha response code which arrives in request message. The following is the sample code with verify(String response) API. Pay attention to some of the following in the code given below:
- Google Recaptcha URL such as https://www.google.com/recaptcha/api/siteverify
- Google googleRecaptchaSecretKey is autowired. You may want to take the value of RecaptchaSecretKey from Google Recaptcha Website and place it in application.properties file and read it as one of the Configuration bean. The following is the sample code for application file and configuration bean.
- Application.properties file code
google.recaptcha.site.key = 6YbGpACUBBBBBJ9pa2Km3vYeVCBV8UHYGic-dbGD
google.recaptcha.secret.key = 6LdWpVEUBBBBBI4xJU7n1MN6tOZablGjnguy123B - AppConfig.java code
@Configuration @PropertySource("classpath:application.properties") public class AppConfig { @Value("${google.recaptcha.site.key}") String googleRecaptchaSiteKey; @Value("${google.recaptcha.secret.key}") String googleRecaptchaSecretKey; @Bean public String googleRecaptchaSiteKey() { return this.googleRecaptchaSiteKey; } @Bean public String googleRecaptchaSecretKey() { return this.googleRecaptchaSecretKey; } }
- Application.properties file code
- A RestClient is used autowired. You can create a simple RestClient using Spring RestTemplate.
import java.net.URI; import org.json.JSONException; import org.json.JSONObject; import org.slf4j.Logger; import org.slf4j.LoggerFactory; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Service; import com.vf.nimki.rest.RestClient; @Service public class RecaptchaVerifier { final static Logger logger = LoggerFactory.getLogger(RecaptchaVerifier.class); private String secretKey; private RestClient restClient; private static final String RECAPTCHA_URL = "https://www.google.com/recaptcha/api/siteverify"; @Autowired public RecaptchaVerifier(String googleRecaptchaSecretKey, RestClient springRestClient) { this.secretKey = googleRecaptchaSecretKey; this.restClient = springRestClient; } public boolean verify(String recaptchaResponse) { URI verifyUri = URI .create(String.format(RECAPTCHA_URL + "?secret=%s&amp;amp;response=%s", this.secretKey, recaptchaResponse)); JSONObject jsonResponse = null; try { jsonResponse = this.restClient.get(verifyUri); } catch (JSONException e) { logger.error("Error in Recaptcha Verification: " + e.getMessage()); return false; } boolean isVerified = false; try { isVerified = jsonResponse.getBoolean("success"); } catch (JSONException e) { logger.error("Error in JSON processing: " + e.getMessage()); return false; } return isVerified; } }
Spring/Java App – Verify the Recaptcha Response in Server Side Code
The following code can be used to verify the Recaptcha response which arrives in the request message.
@PostMapping(value ="/signup") public UserDTO signup(@RequestBody UserDTO userDTO, @RequestParam(name="g-recaptcha-response") String recaptchaResponse){ LOGGER.info("Initiating the user signup processing"); boolean verified = recaptchaVerifier.verify(recaptchaResponse); if(!verified) { userDTO.setErrorcode("RECAPTCHA_VERIFICATION_ERROR"); userDTO.setErrormsg("Recaptcha verification error. Please try again!"); return userDTO; } }
Further Reading/References
Summary
In this post, you learned about how to implement Google Recaptcha in your Angular and Spring/Java app.
Did you find this article useful? Do you have any questions or suggestions about this article? Leave a comment and ask your questions and I shall do my best to address your queries.
- Logistic Regression in Machine Learning: Python Example - April 26, 2024
- MSE vs RMSE vs MAE vs MAPE vs R-Squared: When to Use? - April 25, 2024
- Gradient Descent in Machine Learning: Python Examples - April 22, 2024
Leave a Reply