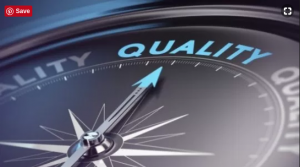
Code quality concepts must be understood well by software developers to write a good quality code. Also, technical lead and technical architects must understand the code quality (or coding) concepts very well in order to review the code (termed as code review) and ensure that good quality software is delivered to the customer.
Pictorially, the following represents some of the experiences that software developers, tech leads or technical architects go through while developing software or reviewing software code quality. Enjoy the pictures :).
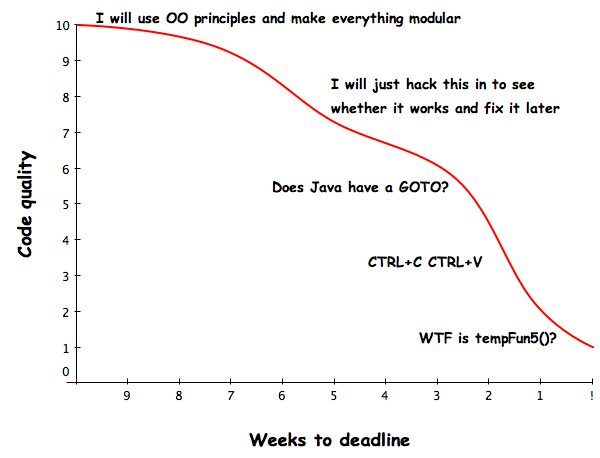
Figure 1. Code Quality Statuses in SDLC
Often code quality review of poorly written code results in lot of frustrations among project stakeholders. Thus, it is of utmost important to understand different nuances of writing code of good quality.
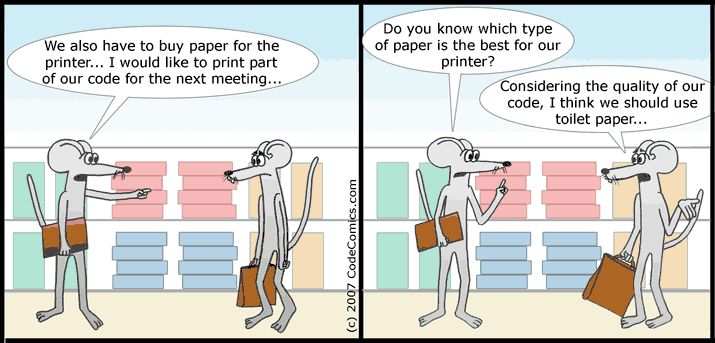
Figure 2. Poor Code Quality Sucks
Who would not want a good code review sessions with very less number of review comments? Good for both code reviewers and the software developers who developed that code.
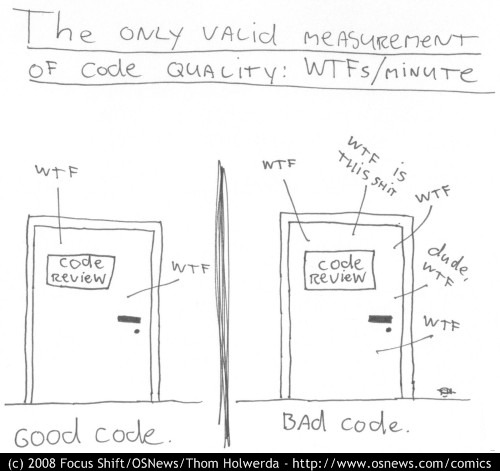
Figure 3. Code Review Comments vis-a-vis Code Quality
In this post, you would learn about some of the following:
- A quick introduction to object-oriented coding principles, code smells etc.
- Sample interview questions
- Practice test
Object-oriented Coding Principles vis-a-vis Code Quality
- One can write good quality code by following SOLID principles
- S: Single Responsibility Principle (SRP) – A block of code must have just one reason to change or perform single functionality.
- O: Open-closed Principle (OCP) – A block of code such as class is open for extension but closed for modification.
- L: Liskov substitution principle (LSP) – A reference of derived class can be used (substituted) in place of base class.
- I: Interface segregation principle (ISP) – Large interfaces can be broken down into small interfaces representing cohesive contracts.
- D: Dependency inversion principle (DIP) – A high-level module should not depend on low-level modules. Rather, both should depend on abstractions.
- DRY is another principle which states that code must not be duplicated. DRY stands for Don’t Repeat Yourself. Following DRY principle would enhance code maintainability.
- A code following SRP will have higher cohesiveness and reusability.
- A code having high cohesiveness will have high reusability.
- Code quality can be measured as a function of the cyclomatic complexity of the code; Cyclomatic complexity is a count of linearly independent paths through the program source code. One can determine the cyclomatic complexity of code by counting the number of conditional expressions (such as if-then-else, do-while, switch-else, for etc) in the code.
- Higher the cyclomatic complexity of the source code, lower is the possibility of achieving high unit test coverage of code which could impact the code maintainability.
- The following are some of the code smells which could be found with code violating single responsibility principle:
- Long class
- Long method
- Large number of conditional expressions
- The following are some of the code refactoring techniques which could fix the violation of single responsibility principle:
- Extract class
- Extract method
- Move method
Common Interview Questions
- What are SOLID principles?
- Ans: SOLID principles are a set of five design principles for object-oriented programming. The acronym SOLID stands for:
S: Single responsibility principle
O: Open closed principle
L: Liskov substitution principle
I: Interface segregation principle
D: Dependency inversion principle
These principles help to create high-quality code by ensuring that individual classes and modules are cohesive and well-defined. They also promote modularity and code reuse, making it easier to maintain and update software over time.
- Ans: SOLID principles are a set of five design principles for object-oriented programming. The acronym SOLID stands for:
- What is Single Responsibility Principle (SRP)? How is it related to reusability and testability of the code?
- Ans:The Single Responsibility Principle is a code quality principle that says every class, module, or function should have a single responsibility. They should have only one reason to change. This makes the code easier to understand, reuse, and test. The single responsibility principle is one of the foundations of good software design. It helps to make code more maintainable and easier to change. When code is written with the single responsibility principle in mind, it is typically more reusable and testable as well.
There are a few things to keep in mind when following the single responsibility principle:
- Every class, module, or function should have a single responsibility.
- The responsibility should be well defined.
- The code should be written in such a way that it is easy to change the code if the responsibility changes.
- Ans:The Single Responsibility Principle is a code quality principle that says every class, module, or function should have a single responsibility. They should have only one reason to change. This makes the code easier to understand, reuse, and test. The single responsibility principle is one of the foundations of good software design. It helps to make code more maintainable and easier to change. When code is written with the single responsibility principle in mind, it is typically more reusable and testable as well.
- What code refactoring techniques could be applied to the code which violates SRP?
- Ans: There are a few code refactoring techniques that we can use to fix code that violates the single responsibility principle. One technique is to move code into a new method or new class. This can help break up the code into smaller, more manageable chunks. Another technique is to use pseudo-code to help clarify the purpose of each method. Finally, we can use data abstraction to create cleaner, more organized code.
- What are some of the code smells that can be found with code violating SRP?
- Ans: There are several code smells that can indicate that a piece of code is violating the single responsibility principle. One smell is excessive coupling, which can occur when two or more methods are too closely linked. Another smell is long methods, which can be difficult to read and understand. Another smell is poorly named variables and methods, which can make it difficult to tell what a piece of code is doing. Finally, another smell is duplicated code, which can lead to confusion and maintenance problems.
- What is the cyclomatic complexity of the code?
- Ans: The cyclomatic complexity of a piece of code is a measure of how complex it is. This number is calculated by counting the number of independent paths through the code. A high cyclomatic complexity can indicate that a piece of code is difficult to understand and maintain. It can also be an indicator of code that is likely to contain errors.
- How is cyclomatic complexity related to unit test coverage?
- Ans: Cyclomatic complexity is a measure of how complex a code base is. The higher the cyclomatic complexity, the more difficult it is to unit test the code. This is because with high cyclomatic complexity, there are more paths through the code that need to be tested. Unit test coverage measures how well unit tests have been written to cover all of these paths.
- What is the open-closed principle? Explain with an example.
- Ans: The open-closed principle (OCP) is a software design principle that states that classes should be open for extension, but closed for modification. This means that you should be able to add new functionality to a class without modifying the class, and you should be able to modify the class without breaking existing code. An example of this would be adding a new method to a collection class. The new method could be added without modifying the existing code, and it would still work with existing objects.
- Explain interface segregation principle with an example.
- What are some of the code refactoring techniques which could help fix single responsibility principle violations?
Practice Questions to Test Your Code Quality Concepts
A class with ______ cohesiveness will have _____ reusability?
A block of code such as class or method serving multiple different functionality tends to violate which of the following?
Which of the following refactring technique can be helpful in increasing the cohesiveness of the code?
A class having high maintainability will tend to have which of the following characteristics?
Which of the following principle states that a class should be closed for modification and open for extension?
Which of the following tends to decrease code maintainability?
The code smell such as long classes or long methods tend to violate which of the following principle?
Which of the following principle is related with inheritance concept?
Which of the following principle states that the reference of derived class can be used in place of base class?
DRY principle is related to which of the following?
“Don’t call us, we will call you” is related to which of the following principle?
Large interfaces can be related with which of the following principle?
Which of the following principle is related with polymorphism concept?
Share your Results:
Further Reading
Summary
In this post, you learned about some of the following:
- Quick introduction to code quality principles
- Practice tests on code quality concepts
- Sample interview questions
Did you find this article useful? Do you have any questions about this article or understanding code quality principles? Leave a comment and ask your questions and I shall do my best to address your queries.
- Completion Model vs Chat Model: Python Examples - June 30, 2024
- LLM Hosting Strategy, Options & Cost: Examples - June 30, 2024
- Application Architecture for LLM Applications: Examples - June 25, 2024
Leave a Reply