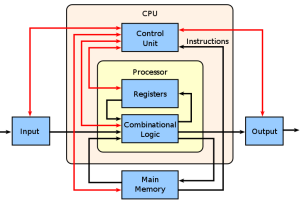
Computer science is an expansive field with a variety of areas that are worth exploring. Whether you’re just starting out or already have some experience in computer science, there are certain topics that every aspiring software engineer should understand. This blog post will cover the basic computer science topics that are essential for any software engineer or software programmer to know.
Computer Architecture
Computer architecture is a course of study that explores the fundamental elements of computer building and design. It’s an important field of study for software engineers to understand, since it provides basic principles and concepts related to hardware and software interactions. Computer architecture courses typically cover a variety of topics, such as some of the following:
- Processor structure
- Computer networks
- Logic gates
- System buses
- Memory hierarchy, virtual memory, multi-level caches
- I/O system design
Through this in-depth knowledge of computer components and how they operate together, software engineers gain insight on how best to interface with the hardware when building their applications. Additionally, understanding computer architecture helps software engineers develop efficient programs by taking full advantage of available technologies such as multiple core processors and multi-threading coding techniques. Computer architects bridge the gap between the mathematics used by engineers to design computing components and the skills a software developer needs to create sophisticated algorithms for computing systems that take maximum advantage of today’s highly advanced technology implementations. By learning everything from instruction set architectures to microprogramming processes, students are able to connect knowledge gained in different Computer Architecture courses into a working whole while preparing them for future Software Engineering challenges.
Data structures & Algorithms
Data structure & algorithm are central to most aspects of computer science. Data structures provide an effective way to store, organize, and manage data so that it can be efficiently used to solve various problems. Algorithms provide the instructions for manipulating data and solving complex problems in a computationally efficient manner. For software engineers, it is essential to have a strong understanding of Data structure & algorithm in order to design, develop, optimize, and analyze applications that rely heavily on computers. A Data structure & algorithm course covers a variety of topics such as some of the following:
- Linear and nonlinear data structures
- Sorting algorithms
- Graph algorithms
- Dynamic programming
- String matching algorithms
- Numerical methods
The theoretical concepts discussed in such courses equip learners with the necessary tools needed to design efficient implementations of 3rd party abstractions. Additionally, such skills can help software engineers gain an advantage when faced with difficult coding problems or complex real-world data sets. Data structures & algorithms are not only important for software engineers but can also benefit other professionals such as data scientists or web developers who require solid knowledge of computational foundations. In summary Data structure & algorithm is fundamental for aspiring professionals who work with digital technologies.
Mathematics for Computer Science
Mathematics for computer science is the mathematical knowledge and tools necessary for the effective use of computers in addressing advanced problems. It is designed to provide students with a foundation for understanding and utilizing methods developed in mathematics to solve computer science intractable problems. Mathematics for computer science is important for software engineers because it provides them with a wide variety of theoretical concepts that can be applied to virtually any programming situation. Mathematics for Computer Science covers all the necessary topics such as some of the following:
- Basic number theory to discrete mathematics (Sets, functions, algorithms, probability, etc)
- Matrices and vectors
- Computability theory
- Automata theory
- Graph theory
- Linear algebra
With each of the above mentioned courses, software engineers can develop strong problem solving skills while also gaining valuable insights into other aspects of computing such as probability or data analysis. Mathematics for Computer Science is vital to the development of new software systems; without it, software engineers would not be able to incorporate sophisticated techniques into their programs and applications.
Operating Systems
Operating systems are programs that manage the hardware and software resources of a computer, allowing it to run applications. Operating systems also provide services such as running programs in virtual memory space, file system management, security, object-oriented programming features, networking capabilities and more. Operating Systems are an essential part of any software engineering program. A course in Operating Systems provides students with a unique opportunity to gain familiarity with both hardware and software as they develop an understanding of how Operating Systems interact with other system components. Specific topics covered in Operating System classes can be some of the following:
- Processes and threads
- Processor scheduling algorithms
- Mutual exclusion algorithms and synchronization constructs
- Inter-process communication mechanisms
- Memory management strategies
- Techniques for managing concurrent access to shared data
Learning Operating Systems helps software engineers develop comprehensive problem solving skills by providing them with the tools necessary to analyze system performance issues at all stages of the production process. It is therefore essential for software engineers to have knowledge in Operating Systems before attempting to design or build large scale software systems.
Computer Networking
Computer networking is one of the most important concepts for software engineers to learn. Computer networks allow people to transfer data, share resources and communication quickly and securely. Therefore, learning Computer networking is essential for software engineering as it covers the basic understanding of technology needed to develop modern software systems. In a Computer Networking course, topics such as some of the following are taught:
- IP addressing & subnetting
- Network security protocols
- Local area networks (LANs)
- Wide area networks (WANs)
- Switching technologies
- Wireless communications
- Variety of network devices such as routers, switches, etc. and their functions.
Computer networking can also provide an understanding of how Computer hardware works together and help professionals troubleshoot issues that may be found in web development projects. Knowledge in Computer networking is not only necessary for web development but also required to create software applications that run on computer networks; this requires an understanding of how to code for different types of equipment or platforms. All in all, Computer networking provides critical knowledge for helping engineers understand how computers communicate with one another which is essential for any successful software engineer today.
Databases
Databases are computerized storage management systems that allow software engineers to organize, store and retrieve data quickly and accurately. Databases are particularly important in software engineering as they provide the ability to store large amounts of data while allowing efficient retrieval methods and ensuring reliability and accuracy. Databases also allow software engineers to build up a complete record of user interactions over time, allowing them to gain insights into customer behavior. In order to understand Databases fully, it is essential for software engineers to take courses dedicated Databases. These courses will teach key topics such as some of the following:
- Relational Databases
- Database design techniques
- Indexing techniques.
- Manipulating complex datasets
- Advanced concepts such as distributed Databases and NoSQL Databases
- Content structure and architecture
- Space analysis
- Query optimization strategies
- Recovery strategy
- Database security
Students will also learn the basics of database structures including relational models, databases management systems and transaction processing. Databases can be complex but understanding them is essential for any experienced developer. That’s why it so important for software engineers to take the time to learn about Databases, so that they can develop better applications with more reliable data management capabilities.
Distributed Systems
Distributed Systems provide software engineers with an understanding of the theoretical and practical aspects of designing, implementing, managing, and evaluating distributed systems such as peer-to-peer networks. Distributed systems encompass a wide range of topics, from hardware security to performance optimization. Distributed Systems courses provide students with the knowledge and skills to address challenging problems encountered in enterprise applications. Learning Distributed Systems is essential for modern software engineering because it enables effective design of distributed systems that can take advantage of parallelism for scalability, fault tolerance for reliability, optimized communication between components, and better management of storage resources. Distributed Systems covers various key topics such as some of the following:
- Consistency models
- Coordination protocols
- Fault-tolerance & replication mechanisms
- Distributed transactions
- Distributed database systems
- Distributed file systems
- Distributed storage technologies
- Distributed scheduling algorithms
- Remote calls/ invocations
- Distribution algorithms such as map-reduce
- Other data processing methods
By learning Distributed Systems principles in depth through coursework or self-study, software engineers can unlock their full engineering potential. With this valuable knowledge set they will be prepared to create highly dependable services at scale while maintaining robust security across their designs.
Languages & Compilers
Languages & Compilers is a course designed to help software engineers learn how to understand and create different programming languages. This course explores the topics such as some of the following:
- Fundamentals of programming language development, including language structure and design, parsing algorithms, type systems, debugging techniques and optimization methods
- Object-oriented programming paradigms
- Code translation techniques
- Logic handling
- Program execution
Languages & Compilers plays an important role in helping software engineers develop a deep understanding of programming language design and implementation. The course therefore not only teaches students essential principles of Languages & Compilers but also introduces them to the nuances of each language they study. In addition to providing a comprehensive overview of Languages & Compilers concepts, the Languages & Compilers course also helps students develop key skills that are required for successful design and implementation of any programming language. As such, Languages & Compilers is an important tool for software engineers that want to stay current with their craft and understand the various approaches for creating flawless code.
Programming
Programming is the process of writing instructions for a computer to achieve a desired outcome. In software engineering, Programming plays an essential role in allowing engineers to quickly create unique applications that can automate certain tasks. Programming courses generally cover a wide range of topics such as some of the following:
- Coding
- Debugging
- Web development
- Object-oriented programming
- Algorithm design
- Programming language syntax fundamentals
- Core data structures and algorithms
Learning Programming requires deep technical knowledge, but it also takes patience and perseverance. As engineers become more adept at Programming they gain the confidence needed to tackle challenging problems with creativity and skill. Programming also helps increase problem-solving speed while improving communication with team members. All of these skills are invaluable to those working in software engineering and make learning Programming an important component of their training. By understanding why Programming is valuable, software engineers can take ownership of their mastering this key area enabling them to develop powerful software solutions efficiently.
Cloud Computing Fundamentals
Cloud computing fundamentals is an essential course for software engineers interested in advancing their skills and staying up to date with current trends. Cloud computing involves outsourcing computing services such as data storage, networking, and databases to remote infrastructure hosted on the Internet. The Cloud computing fundamentals course teaches some of the following:
- Fundamentals of cloud-based platforms
- Methods, and technologies that make cloud computing possible
- Cloud service models such as Infrastructure as a Service (IaaS), Platform as a Service (PaaS) and Software as a Service (SaaS)
- Cloud deployment models
- Virtualization technologies and containerization tools
- Cloud-based DevOps pipelines using CI/CD toolsets
Learning the fundamentals gives one a comprehensive understanding of the technology behind Cloud Computing and serves as an excellent foundation for those who wish to pursue higher qualifications in Cloud Computing architecture design or implementation. Additionally, with knowledge about Cloud fundamentals, software engineers can develop Cloud optimised designs for applications. This makes them highly sought after in the rapidly evolving cloud industry where new advancements are taking place every day. In summary, it is important for software engineers to learn Cloud computing fundamentals so they can be well informed about current industry standards and have all the necessary knowledge for designing complex application architectures utilising the Cloud infrastructure effectively.
Conclusion
Computer science is a vast field with many areas worth exploring. Some topics, like algorithms and data structures, programming, computer architecture, networking fundamentals, etc are essential concepts every aspiring software engineer should understand before embarking on their software development and coding journey. With proper knowledge in these topics, you’ll be well on your way towards becoming an expert software engineer!
- Completion Model vs Chat Model: Python Examples - June 30, 2024
- LLM Hosting Strategy, Options & Cost: Examples - June 30, 2024
- Application Architecture for LLM Applications: Examples - June 25, 2024
It’s good