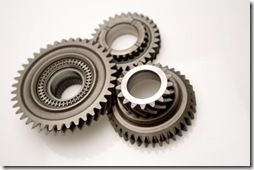
The article intends to provide take away code to get started with unit testing while working with AngularJS. The underlying Javascript unit testing framework used for testing the AngularJS code sample is Jasmine.
- Controllers.js
- ControllersSpecs.js
You may want to pay attention to some of the following facts in relation with unit testing vis-a-vis unit testing controller methods:
- Use AngularJS dependency injection feature to pass on dependencies to Angular components such as controller, filters, directives etc. Following code does the magic of injecting $rootScope and $controller which is also represented in the code samples later in this article. For rookies, following should be sufficient for cut-copy-paste to get started with unit testing.
// Module helloApp initialized // module("helloApp"); // Controller and rootScope injected for initializing // the controller and working with scope object in "it" function blocks // inject(function($controller, $rootScope){ $scope = $rootScope.$new(); $controller( "HelloCtrl", {$scope:$scope}); });
- In Angular, the controllers are strictly separated from the DOM manipulation logic and this results in a much easier testability. You would not see any HTML code in the example shown below.
Controllers.js
Following represents the AngularJS code representing Hello world app consisting of one function named as addProfile. The demo apps could be found on following pages:
var helloApp = angular.module("helloApp", []);
helloApp.controller("HelloCtrl", function($scope) {
$scope.name = "Calvin Hobbes";
$scope.profiles = [];
$scope.addProfile = function(){
var profile = {firstname:$scope.firstname,lastname:$scope.lastname,email:$scope.email};
$scope.profiles.push( profile );
}
});
ControllersSpecs.js
Following represents the Jasmine code sample used to test the above code. Pay attention to “BeforeEach” setup function which initializes the HelloApp and create a controller with name “HelloCtrl”. Note that the HTML page should consist of directives such as ng-app=”helloApp” and ng-controller=”HelloCtrl” appropriately.
describe( "HelloWorld Controller", function() {
//
// Defining the scope variable to be accessed within "it" function
// blocks
//
var $scope;
//
// Setup function called prior to each "it" function block
//
beforeEach(function(){
// Module helloApp initialized
//
module("helloApp");
// Controller and rootScope injected for initializing
// the controller and working with scope object in "it" function blocks
//
inject(function($controller, $rootScope){
$scope = $rootScope.$new();
$controller( "HelloCtrl", {$scope:$scope});
});
});
it( "should have default name set to Calvin Hobbes", function() {
expect($scope.name).toEqual("Calvin Hobbes");
});
it( "should have an empty profiles array to store profiles", function() {
expect($scope.profiles.length).toEqual(0);
});
it( "should add a profile to profiles store", function(){
$scope.firstname = "Chris";
$scope.lastname = "Johnson";
$scope.email = "chris.johnson@gmail.com";
$scope.addProfile();
//
// Expectations
//
expect( $scope.profiles.length ).toEqual(1);
expect( $scope.profiles[0].firstname ).toEqual( "Chris" );
expect( $scope.profiles[0].lastname ).toEqual( "Johnson" );
expect( $scope.profiles[0].email ).toEqual( "chris.johnson@gmail.com" );
});
});
How to Run Above Tests
We recommend you to get setup with Karma & Jasmine using instructions that could be found on following page: http://vitalflux.com/angularjs-7-steps-unit-testing-angularjs-scripts-jasmine/
…
[adsenseyu1]
- Three Approaches to Creating AI Agents: Code Examples - June 27, 2025
- What is Embodied AI? Explained with Examples - May 11, 2025
- Retrieval Augmented Generation (RAG) & LLM: Examples - February 15, 2025
I found it very helpful. However the differences are not too understandable for me