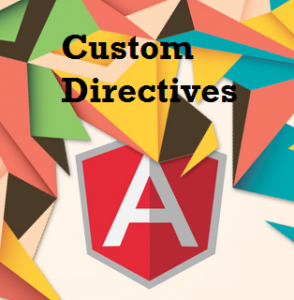
The article presents high level concepts and take away code sample on how to create custom directive in AngularJS. The code given below is demonstrated on this page, http://hello-angularjs.appspot.com/angularjs-how-create-custom-directives. Following are key concepts that shall be discussed in this article:
- What is a directive?
- Why do you need a directive?
- Code sample – Hello World Custom Directive
What is a Directive?
Simply speaking, a directive in AngularJS is all about extending (or attaching) the behavior of an existing element or creating a new element with altogether a new behavior satisfying the specific requirements of your app. From code perspective, directives are markers on a DOM element (such as an attribute, element name, comment or CSS class). Directives asks AngularJS’s HTML compiler ($compile) to attach a specified behavior to that DOM element or even transform the DOM element and its children.
Lets take a look at an example below. Following is the angular code which prints “Hello, {{name}}” as user enters his name in the input. In the code below, directive is NOT used.
<div ng-controller="HelloCtrl">
<input type="text" ng-model="name"/>
Hello, {{name}}
</div>
Lets write the same code with the help of a “say-hello” directive. Please note that “helloApp” is a reference to the app initialized on the page. The code below demonstrates some of the following:
- template property: Creating a custom directive in its simplest form by making use of just the “template” property. One could rather write the template code within an HTML file and get the same thing achieved using a templateUrl property and assigning it the HTML file like following: templateURL: ‘customdirective.html’.
- restrict property: Property named as “restrict” which specifies the fact that the directive could be used as both as an element and also attribute. Other supported types are class (C) and comment (M). You could find further details on this angularjs directives tutorial page.
- scope (isolate scope): Pay attention to the fact that the scope defined within the directive can also be termed as isolate scope, meaning the scope does not inherit from the rootScope and hence is separated from outside scope. The directive could be independently used across different controllers in the same module. Also, notice how the “name” variable of isolate scope is bound to attribute name, “name”.
<div ng-controller="HelloCtrl">
<input type="text" ng-model="name"/>
<say-hello name="name"></say-hello>
</div>
<script type="text/javascript">
helloApp.directive( "customDirective", function() {
return {
restrict: 'EA',
scope: {
name: '='
},
template: 'Hello, {{name}}'
}
});
</script>
In general, following code could be used to create a custom directive. As like controllers, directives are registered to modules. To register a directive, one can use module.directive API as shown below (where helloApp is a module instantiated using code such as angular.module( “helloApp”, [] ) ). In the code below, you would observe that the API takes the name of directive as one parameter, and then, inline annotation array which can be a list of dependent services (such as depService in example below) followed by a factory function.
var helloApp = angular.module( "helloApp", [] );
helloApp.directive( "directiveName", [ "depService", function( depService ) {
...
}]);
Why is needed a Custom Directive?
Following are some of the key reasons from what I understood so far. Please feel free to suggest others.
- Get rid of HTML boiler plate code that needs to be written at multiple places in the page
- Ease of use & to maintain in terms of ease of change where you change at one place and it gets changed for all occurences
- Reusability
- Readable code
Code Sample – Hello World Custom Directive
Following is the take away code which you could use to quickly get started with creating custom hello world directive. In the example below, the directive say-hello is created, both as an attribute and also as an element.
<!DOCTYPE html>
<html ng-app="helloDirectives">
<head>
<title>AngularJS - How to Create Custom Directives</title>
<link rel="stylesheet" href="//maxcdn.bootstrapcdn.com/bootstrap/3.2.0/css/bootstrap.min.css">
</head>
<body ng-controller="DirectivesCtrl">
<div class="page-header">
<h1>Hello World with Custom Directives</h1>
</div>
<form class="form-horizontal" role="form">
<div class="form-group">
<label class="col-md-2 control-label">Type Your Name</label>
<div class="col-md-4">
<input type="text" class="form-control" ng-model="name"/>
<div ng-show="name">
<say-hello name="name"></say-hello>
</div>
</div>
</div>
</form>
<script src="http://ajax.googleapis.com/ajax/libs/angularjs/1.2.21/angular.min.js"></script>
<script>
var helloDirectives = angular.module( "helloDirectives", [] );
helloDirectives.controller( "DirectivesCtrl", [ '$scope', function( $scope ){
$scope.name = "";
}]);
helloDirectives.directive( "sayHello", function() {
return {
restrict: 'EA',
scope: {
name: '='
}
template: '<h2>Hello, {{name}}</h2>'
};
});
</script>
</body>
</html>
…
[adsenseyu1]
- Completion Model vs Chat Model: Python Examples - June 30, 2024
- LLM Hosting Strategy, Options & Cost: Examples - June 30, 2024
- Application Architecture for LLM Applications: Examples - June 25, 2024
Leave a Reply