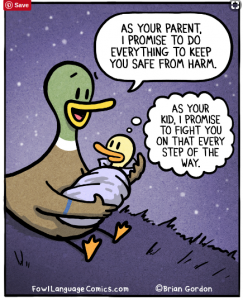
Angular HttpClient got recently released in Angular 4 (later versions) and then formalized in Angular 5. Going forward, Angular recommends usage of HttpClient for communicating with backend services over the HTTP protocol.
Before getting started, on a lighter note, check out this comic strip on Promise. :). In Javascript, Promises are either resolved or rejected. In other words, Promise always transition from state of pending to resolution or reject.
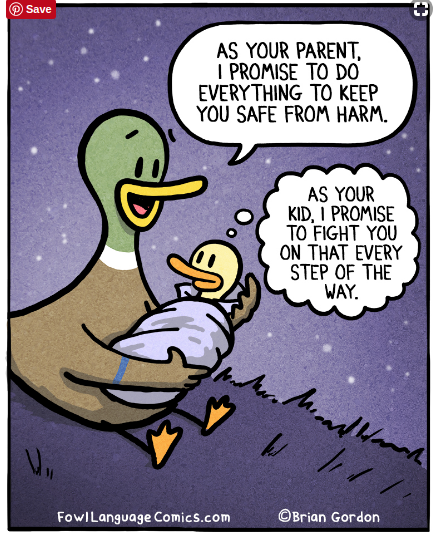
Figure 1. Some keep promises, some reject them 🙂
In this post, you will learn some of the following:
- How to use HttpClient Get API and Promise
- Processing Response with HttpCient Get API made to return Promise
- Sample Interview Questions
How to use HttpClient Get API and Promise
The code given below represents usage of Promise with Angular HttpClient service. This is a prescription service (RxService) which retrieves all the prescriptions for a doctor.
import {Http, Headers, RequestOptions} from '@angular/http'; import {Injectable} from '@angular/core'; import {Rx} from './rx'; import {Observable} from 'rxjs/Observable'; import {Cookie} from 'ng2-cookies'; import {HttpClient, HttpHeaders, HttpResponse} from "@angular/common/http"; @Injectable() export class RxService { private rxUrl = 'http://localhost:8080/rx'; constructor(private httpClient: HttpClient) { } getRx(): Promise<Rx[]> { return this.httpClient.get(this.rxUrl, {observe: 'response', headers: new HttpHeaders().set('Authorization', 'Bearer ' + Cookie.get('access_token'))}) .toPromise() .then(this.extractData) .catch(err => { return Promise.reject(err.error || 'Server error'); }); } extractData(res: HttpResponse<Object>) { var array = new Array(); var key, count = 0; for(key in res.body) { array.push(res.body[count++]); } return array; } }
Note some of the following in above code example:
- Option observe: ‘response’: This instruct the server to return full response including data and header information comprising of special headers or status codes to indicate certain conditions.
- Option HttpHeaders: HttpHeaders object is used to set Authorization information in outgoing request. Note that HttpHeader is an immutable class which implies that invoking set() would return a new instance.
- Instance of HttpResponse<Object> is returned from the GET API. Note the processing of response data.
- Notice usage of RxJS toPromise() method to convert Observable into a Promise object.
- Code within Promise results in resolution or rejection (Promise.reject).
Processing Response with HttpCient Get API made to return Promise
The code given below represents RxListComponent which displays a list of prescription retrieved using RxService shown above. Note the usage of then method to process the returned Promise object.
import {RxService} from './rx.service'; import {Rx} from './rx'; import {Component, OnInit} from '@angular/core'; import {Router} from '@angular/router'; @Component({ templateUrl: './rx-list.component.html' }) export class RxListComponent implements OnInit { rxlist: Rx[]; errorMessage: string; constructor(private rxService: RxService, private router: Router) { this.errorMessage = ''; } ngOnInit(): void { this.getRx(); } getRx() { this.rxService.getRx() .then(rxlist => { this.rxlist = rxlist; if (this.rxlist.length === 0) { this.errorMessage = 'There are no prescriptions you have created so far!'; } }, error => { this.router.navigateByUrl('/auth/login'); console.error('An error occurred in retrieving rx list, navigating to login: ', error); }); } }
Sample Interview Questions
- How to use Promise with HttpClient service?
- How do you send Authorization data in outgoing request from HttpClient service? Explain with code examples.
- How to get entire response object as part of response? What is observe option?
Further Reading
Summary
In this post, you learned about some of the following:
- How is Promise used with Angular HttpClient service?
- Sample interview questions
Did you find this article useful? Do you have any questions about this article or understanding the usage of Promise with Angular HttpClient service (Get API)? Leave a comment and ask your questions and I shall do my best to address your queries.
- Completion Model vs Chat Model: Python Examples - June 30, 2024
- LLM Hosting Strategy, Options & Cost: Examples - June 30, 2024
- Application Architecture for LLM Applications: Examples - June 25, 2024
Leave a Reply