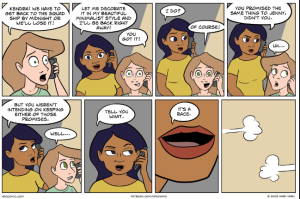
Promise, in Javascript, allows an asynchronous operation to happen in the sense that caller function getting promise object returned by callee function proceeds ahead with the program execution. And, upon the return of promise object, the processing continues. The Promise concept can be used with Angular Http service to achieve asynchronous data retrieval from the server using GET API. The code example in this article applies to both Angular 2 and Angular 4.
On the lighter side, here is a comic strip on whether Promises are kept or not :). In Javascript, promises go from state of “pending” to “resolution” or “rejection”.
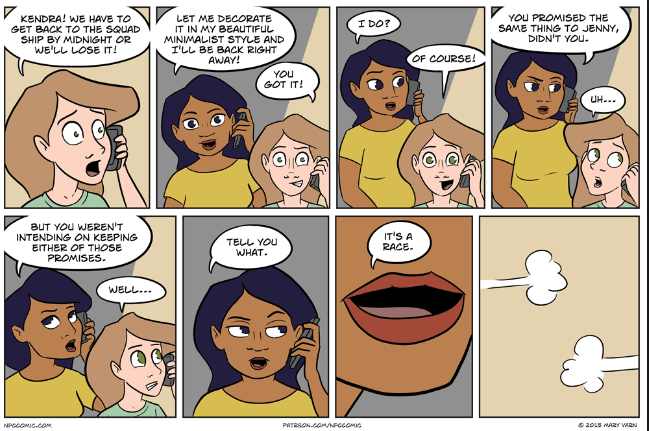
Figure 1. In Javascript, promises are either resolved or rejected
In this post, you will learn about some of the following in relation to using promise concept vis-a-vis an Angular 4 or Angular 2 Http Service:
- Promise used with Angular Http Service (Http Get API)
- Sample interview questions
- Practice Test
Promise used with Angular Http Service (Get API)
In this section, you will learn the usage of Promise with Angular Http service (Get API).
The following represents the code of a custom Angular Service making use of Http service for making REST API call. The code given below has an API namely getRx. The getRx API internally invokes a REST API call over Http to get the prescription information from the server for a logged in user.
import {Http, Headers} from '@angular/http'; import {Injectable} from '@angular/core'; import {Rx} from './rx'; import {Cookie} from 'ng2-cookies'; @Injectable() export class RxService { private rxUrl = 'http://localhost:8080/rx'; constructor(private http: Http) { } getRx(): Promise<Rx[]> { let headers = new Headers({ 'Content-type': 'application/json', 'Authorization': 'Bearer ' + Cookie.get('access_token') }); return this.http.get(this.rxUrl, {headers: headers}) .toPromise() .then(res => res.json()) .catch(err => { return Promise.reject(err.json().error || 'Server error'); }); } }
Make a note of some of the following in above code:
- Http service is injected in the constructor. Http is part of @angular/http library.
- Get API is invoked on Http service.
- The http service get method returns an Observable object.
- On an Observable object, RxJS toPromise() method is called which converts the observable to Promise object.
- On the Promise object, the method then is invoked which returns the Promise<Rx[]>.
The code below represents the processing of returned Promise<Rx[]> in caller method. Make a note of usage of then function.
export class RxListComponent implements OnInit { rxlist: Rx[]; errorMessage: string; constructor(private rxService: RxService, private router: Router) { this.errorMessage = ''; } ngOnInit(): void { this.getRx(); } getRx() { this.rxService.getRx() .then(rxlist => { this.rxlist = rxlist; if (this.rxlist.length === 0) { this.errorMessage = 'There are no prescriptions you have created so far!'; } }, error => { this.router.navigateByUrl('/auth/login'); console.error('An error occurred in retrieving rx list, navigating to login: ', error); }); } }
Sample Interview Questions
- How to use Promise with Http service in Angular App?
- Does Angular Http get API returns a promise object?
- How is a returned promise object processed? Show with code example.
Practice Test
Angular Http Get API returns which of the following?
Angular Http service is part of which of the following library?
Which of the following needs to be invoked to convert Observable into a Promise object?
Share your Results:
Further Reading
Summary
In this post, you learnt about some of the following:
- How is Promise concept used with Angular Http service (Get API)
- Sample interview questions
- Practice tests to check your knowledge
Did you find this article useful? Do you have any questions about this article or understanding the usage of Promise with Angular Http service (Get API)? Leave a comment and ask your questions and I shall do my best to address your queries.
- Completion Model vs Chat Model: Python Examples - June 30, 2024
- LLM Hosting Strategy, Options & Cost: Examples - June 30, 2024
- Application Architecture for LLM Applications: Examples - June 25, 2024
In Practice test, the third question – “Which of the following needs to be invoked to convert Observable into a Promise object?” Both promise() or toPromise() will mark as wrong