This blog represents code samples and related concepts on how to create a feature module in an Angular app (Angular 2/Angular 4). In this app, a feature module for Signup is created.
The following is the screenshot for Signup module:
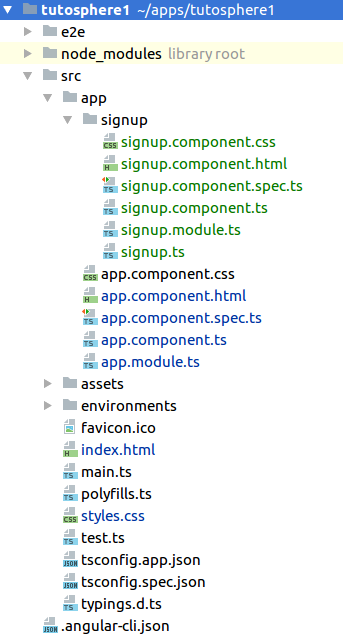
Figure 1. Sample Feature Module in Angular
The following are some of the key aspects to consider when creating a separate feature module:
- Place all the files for feature module in the seperate folder. Note the “signup” directory under src/app folder.
- Feature module would have an NgModule module. Following is how the code looks like for signup module:
import { NgModule } from '@angular/core'; import { SignupComponent } from './signup.component'; import {FormsModule} from '@angular/forms'; import {BrowserModule} from '@angular/platform-browser'; <strong>@NgModule</strong>({ <strong>declarations: [ SignupComponent ], imports: [ BrowserModule, FormsModule ],</strong> providers: [], exports: [SignupComponent] }) export class SignupModule { }
Make a note of some of the following in above code:
- SignupComponent is declared in declarations array.
- SignupComponent is exported and thus, declared in exports array. Thus, the selector of SignupComponent can be used in external modules.
- FormsModule is declared in imports array as the module uses template-driven forms for creating the signup form.
- Define one or more component’s selector of feature module which can be used in other modules. The following code represents the same for SignupComponent:
import { Component } from '@angular/core'; import {Signup} from './signup'; @Component({ <strong>selector: 'app-signup',</strong> templateUrl: './signup.component.html', styleUrls: ['./signup.component.css'] }) export class SignupComponent { title = ''; passwordConfirmationFailed = false; passwordConfirmationTxt = ''; signup = new Signup('', '', '', ''); countries = ['india', 'canada', 'us']; confirmPassword() { if (this.signup.password === this.passwordConfirmationTxt) { this.passwordConfirmationFailed = false; } else { this.passwordConfirmationFailed = true; } } onSubmit() { console.log('Name: ' + this.signup.name + ', Email: ' + this.signup.email + ', Password: ' + this.signup.password ); } }
- Declare feature module (such as SignupModule) in root level module such as app.module.ts or any other module where the feature selector needs to be used. The following code represents the declaration of SignupModule in app.module.ts.
import { NgModule } from '@angular/core'; import {AppComponent} from './app.component'; import {SignupModule} from './signup/signup.module'; @NgModule({ declarations: [ AppComponent ], imports: [ <strong> SignupModule</strong> ], providers: [], bootstrap: [AppComponent] }) export class AppModule { }
- Use selector for feature module appropriately in other modules. The following code represents usage of app-signup selector in app.component.html:
<app-signup></app-signup>
Above would require that SignupModule is imported in the concerned module (app.module.ts) in the current example and, SignupComponent is exported as part of SignupModule.
The following is how the source code of app.component.html looks like:
import { Component } from '@angular/core'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent { }
In case you are developing web apps using Spring and Angular, check out my book, Building web apps with Spring 5 and Angular. Grab your ebook today and get started.
- Mathematics Topics for Machine Learning Beginners - July 6, 2025
- Questions to Ask When Thinking Like a Product Leader - July 3, 2025
- Three Approaches to Creating AI Agents: Code Examples - June 27, 2025
I found it very helpful. However the differences are not too understandable for me