While running unit tests for the Angular app (Angular 2/Angular 4), I came across the error such as following:
Failed: Template parse error:
There is no directive with “exportAs” set to “ngForm”
Here is the screenshot of the error. Note that the form referenced in this blog is a template-driven form.
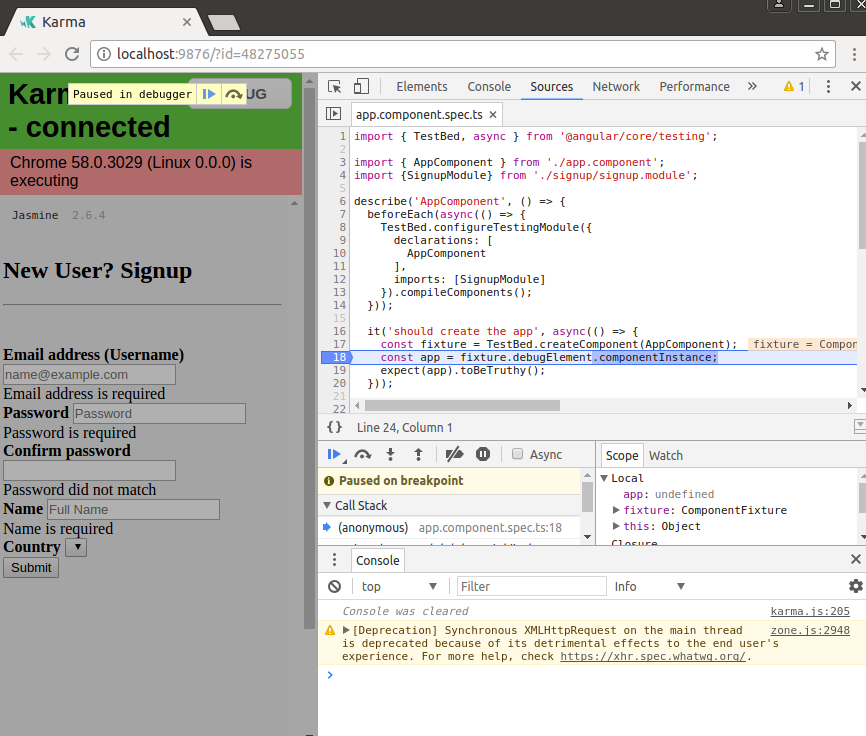
Figure 1. Angular Error No Directive with ExportAs set to ngForm
This blog represents the resolution of above error.
Template and Unit Test Code Before the Fix
The template code defined the template reference variable, #signupForm, for form element as shown in the following code.
<form (ngSubmit)="onSubmit()" #signupForm="ngForm"> </form>
Following was the unit test code prior fix/resolution:
import { TestBed, async } from '@angular/core/testing'; import { SignupComponent } from './signup.component'; import {FormsModule} from '@angular/forms'; describe('SignupComponent', () => { beforeEach(async(() => { TestBed.configureTestingModule({ <strong>declarations: [ SignupComponent ],</strong> }).compileComponents(); })); it('should create the app', async(() => { const fixture = TestBed.createComponent(SignupComponent); const app = fixture.debugElement.componentInstance; expect(app).toBeTruthy(); })); });
Unit Test Code after the Fix/Resolution
Pay attention to the inclusion of FormsModule in imports array.
import { TestBed, async } from '@angular/core/testing'; import { SignupComponent } from './signup.component'; import {FormsModule} from '@angular/forms'; describe('SignupComponent', () => { beforeEach(async(() => { TestBed.configureTestingModule({ <strong>declarations: [ SignupComponent ], imports: [ FormsModule, ],</strong> }).compileComponents(); })); it('should create the app', async(() => { const fixture = TestBed.createComponent(SignupComponent); const app = fixture.debugElement.componentInstance; expect(app).toBeTruthy(); })); });
In case you are developing web apps using Spring and Angular, check out my book, Building web apps with Spring 5 and Angular. Grab your ebook today and get started.
- Agentic Reasoning Design Patterns in AI: Examples - October 18, 2024
- LLMs for Adaptive Learning & Personalized Education - October 8, 2024
- Sparse Mixture of Experts (MoE) Models: Examples - October 6, 2024
I found it very helpful. However the differences are not too understandable for me