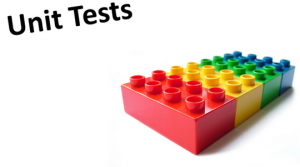
The article presents a perspective on how unit tests could be written in a way that they could be used as an alternate form of documentation for understanding functional requirements.
Many a times, it has been found that developers, primarily rookies, did not have a clarity on how to name unit tests. Thus, many of them have been found to name the unit tests such as testXXX where XXX is name of the method which they wanted to tests. There has been many recommendations on how to name unit tests, some of which are following:
- Given…When…Then… Check the page by Martin Fowler (http://martinfowler.com/bliki/GivenWhenThen.html)
- WhenXXX_ExpectYYY
- Names representing different use case scenarios of a functional requirement
This article takes a shot at naming unit tests representing different use case scenarios of a functional requirement. Feel free to suggest.
public class Calculator {
public Calculator(){
}
public int add( int a, int b ) {
return a + b;
}
}
Unit Tests Generated using Eclipse
Following is a set of of JUnit tests that could be written for above Calculator Class. It can be noted that there is just one unit test named as testAdd which gets added if you create the unit tests for Calculator class using Eclipse. One may require to test different scenarios of addition within testAdd method. And, for developers, it would be difficult to associate tests like these (testXXX) with different scenarios which may be related with one or more functional requirements.
public class CalculatorTest {
private Calculator c;
@Before
public void setUp() throws Exception {
c = new Calculator();
}
@Test
public void testAdd() {
int res = c.add( 3, 4 );
assertEquals( res, 7 );
res = c.add( 3, -4 );
assertEquals( res, -1 );
res = c.add( -3, 4 );
assertEquals( res, 1 );
}
@After
public void tearDown() throws Exception {
c = null;
}
}
Unit Tests Representing Functional Requirements
Following is an another set of JUnit tests that could be written for same Calculator Class. Take a note of following different unit tests methods:
- testAdditionOfPositiveNumbers
- testAdditionOfPositiveAndNegativeNumbers
- testAdditionOfNegativeNumbers
These unit tests’ method names, being self-explanatory, clearly spells out different scenarios which are being tested and not just the method, “add” of the class, Calculator. It helps developers to understand different scenarios which, in turn, be related with one or more functional requirements. Going this approach can achieve some of the following objectives:
- Enhanced readability of unit tests and hence, greater code usability
- Enhanced trace-ability of functionality with unit tests
- Reduction in test smells as a result of increased trace-ability
public class CalculatorTest {
private Calculator c;
@Before
public void setUp() throws Exception {
c = new Calculator();
}
@Test
public void testAdditionOfPositiveNumbers() {
int res = c.add( 3, 4 );
assertEquals( res, 7 );
}
@Test
public void testAdditionOfPositiveAndNegativeNumbers() {
int res = c.add( 3, -4 );
assertEquals( res, -1 );
res = c.add( -3, 4 );
assertEquals( res, 1 );
}
@Test
public void testAdditionOfNegativeNumbers() {
int res = c.add( -3, -4 );
assertEquals( res, -7 );
}
@After
public void tearDown() throws Exception {
c = null;
}
}
- Three Approaches to Creating AI Agents: Code Examples - June 27, 2025
- What is Embodied AI? Explained with Examples - May 11, 2025
- Retrieval Augmented Generation (RAG) & LLM: Examples - February 15, 2025
I found it very helpful. However the differences are not too understandable for me