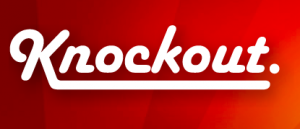
This article represents high level concepts and code example for KnockoutJS, A Javascript framework based on MVVM pattern. The code below is demonstrated on the following page: http://tuts-javascript.appspot.com/helloworld-knockoutjs. Feel free to comment/suggest if I missed on any aspect.
Following is demonstrated as part of hello world demo:
- ViewModel used to represent data and operations on a UI
- Data Binding: Declarative data binding used to create an associated view
- Activating Knockout
- Dynamic Updates: How UI updates dynamically
- Code Example: Hello World Code Sample
ViewModel Representation
View Model (in below example) could be represented in one of the following ways. In code below, the viewmodel object has a property namely, name. Following is an object representation of ViewModel.
var AppViewModel = {
name: ko.observable("Calvin Hobbes")
}
Following is another way of representing ViewModel using “function” call.
function AppViewModel () {
this.name = ko.observable("Calvin Hobbes");
}
Declarative Data Binding
Following code represents how data is bound with input text field and later accessed. Notice the usage of “data-bind” directive. “value: name” is used to bind value related with input textfield with model, “name”. Also, make a note of the fact that
<div class="input-group input-group-lg">
<input class="form-control col-md-8" data-bind='value: name, valueUpdate: "afterkeydown"'/>
</div>
<h1>Hello, <span data-bind="text: name"></span></h1>
Activating Knockout
Following code is used to activate Knockout framework if ViewModel (function AppViewModel() {…} in the example above) is defined using function call
ko.applyBindings(new AppViewModel());
Following code is used to activate Knockout framework if ViewModel is defined like an object:
ko.applyBindings(AppViewModel);
How UI is updated Dynamically?
The code that does the magic is “observable()” method call. To achieve dynamic update behavior, one needs to declare your model properties as “observables”. These are special JavaScript objects that can notify subscribers about changes, and can automatically detect dependencies. Read more about observables on this page. Following code within ViewModel object in the example below does the magic:
this.name = ko.observable("Calvin Hobbes");
Hello World Code Example
Pay attention to some of the following in the code below:
- Input text field is bound (data-bind attribute) with model name, and an event, afterkeydown that updates the model, name. valueUpdate makes sure that “name” used elsewhere (<span data-bind=”text: name”></span>) in the view (UI) gets updated as the input is updated.
<!DOCTYPE html>
<html>
<head>
<title>HelloKO</title>
<link rel="stylesheet" href="//netdna.bootstrapcdn.com/bootstrap/3.1.1/css/bootstrap.min.css">
</head>
<body class="container">
<div class="page-header">
<h1>Knockout HelloWorld - Code Sample</h1>
</div>
<div class="input-group input-group-lg">
<input class="form-control col-md-8" data-bind='value: name, valueUpdate: "afterkeydown"'/>
</div>
<h1>Hello, <span data-bind="text: name"></span></h1>
<script type="text/javascript" src="http://ajax.aspnetcdn.com/ajax/knockout/knockout-3.1.0.js"></script>
<script>
function AppViewModel() {
this.name = ko.observable("Calvin Hobbes");
}
ko.applyBindings(new AppViewModel());
</script>
</body>
</html>
…
[adsenseyu1]
- Book: First principles thinking for building winning products - April 19, 2022
- Free AI / Machine Learning Courses at Alison.com - November 16, 2021
- 12 Weeks Free course on AI: Knowledge Representation & Reasoning (IIT Madras) - November 14, 2021
I found it very helpful. However the differences are not too understandable for me